


Minimizing Variable Scope in Java: Best Practices for Secure and Efficient Code
This article underscores the critical role of minimizing variable scope in Java for cleaner, more maintainable, and secure code. It leverages Java's object-oriented nature, contrasting it with procedural approaches found in languages like C , and illustrates best practices, such as encapsulation and controlled access via methods.
In Java, a variable's scope dictates its accessibility within the program (Mahrsee, 2024). This scope can be class-level, method-level, or block-level. Unlike C , Java lacks global variables—variables accessible throughout the entire program. This inherent limitation on scope is a key strength.
Java's strict object-oriented design (OOP) inherently minimizes scope by encapsulating data within classes. This contrasts with C , which supports both OOP and procedural programming. Scope minimization enhances readability, simplifies maintenance, and reduces errors (Carter, 2021). The SEI CERT Oracle Coding Standard for Java (CMU, n.d.) recommends this practice to prevent common coding mistakes, improve readability by linking variable declaration and usage, and facilitate the removal of unused variables. It can also boost garbage collection efficiency and prevent identifier shadowing.
Restricted scope also bolsters security by limiting variable access to necessary contexts. This reduces the risk of unauthorized modification or misuse, mitigating potential vulnerabilities. For instance, declaring a class variable as private
confines its access to the class itself, preventing external modification. Access and modification are then managed through controlled methods (getters and setters), which can incorporate validation or additional logic to ensure proper usage.
The following Java example demonstrates scope minimization:
public class Employee { private String name; private double salary; public Employee(String name, double salary) { this.name = name; this.salary = salary; } public String getName() { return name; } public double getSalary() { return salary; } public void setSalary(double salary) { if (salary > 0) { this.salary = salary; } else { throw new IllegalArgumentException("Salary must be greater than 0."); } } public void applyBonus(double percentage) { if (percentage > 0 && percentage <= 100) { this.salary *= (1 + percentage / 100); } else { throw new IllegalArgumentException("Bonus percentage must be between 0 and 100."); } } public void printDetails(){ System.out.println("Name: " + this.name); System.out.println("Salary: $" + this.salary); } } public class Main { public static void main(String[] args) { Employee emp = new Employee("Alice", 50000); System.out.println("Initial Salary:"); emp.printDetails(); emp.setSalary(55000); emp.applyBonus(10); System.out.println("\nUpdated Salary:"); emp.printDetails(); System.out.println("\nInvalid salary (-10000):"); try { emp.setSalary(-10000); } catch (IllegalArgumentException e) { System.out.println(e.getMessage()); } } }
Outputs:
Initial Salary: Name: Alice Salary: $50000.0
Updated Salary: Name: Alice Salary: $60500.0
Invalid salary (-10000): Salary must be greater than 0.
In summary, minimizing variable scope in Java enhances code readability, maintainability, and security by restricting access to only where needed. Java's inherent OOP nature, with its data encapsulation within classes, prevents unintended interactions and vulnerabilities, promoting efficient and secure programming practices.
References:
Carter, K. (2021, February 10). Effective Java: Minimize The Scope of Local Variables. DEV Community. https://dev.to/kylec32/effective-java-minimize-the-scope-of-local-variables-3e87
CMU — Software Engineering Institute (n.d.) DCL53-J. Minimize the scope of variables.
SEI CERT Oracle coding standard for Java. Carnegie Mellon University. Software Engineering Institute.
Mahrsee, R. (2024, May 13). Scope of variables in Java. GeeksforGeeks. https://www.geeksforgeeks.org/variable-scope-in-java/
Originally published at Alex.omegapy on Medium by Level UP Coding on November 22, 2024.
The above is the detailed content of Minimizing Variable Scope in Java: Best Practices for Secure and Efficient Code. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
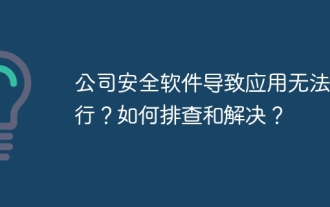
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
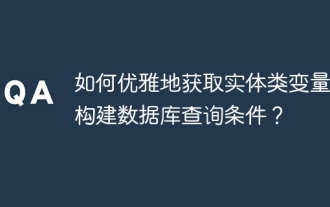
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
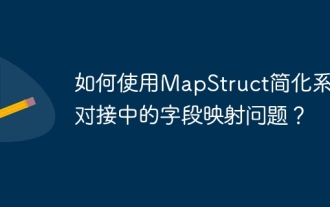
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
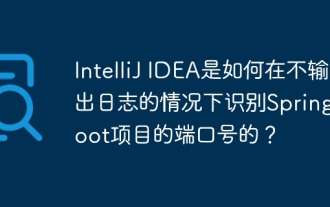
Start Spring using IntelliJIDEAUltimate version...
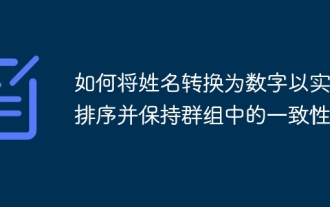
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
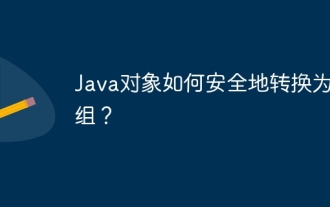
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
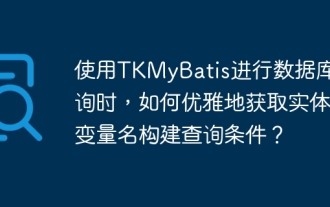
When using TKMyBatis for database queries, how to gracefully get entity class variable names to build query conditions is a common problem. This article will pin...
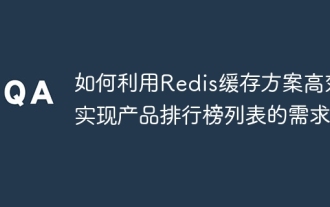
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
