This comprehensive guide explores the intricacies of undefined behavior in C , going beyond typical textbook explanations. Ideal for experienced C programmers, this deep dive into the subject's most obscure aspects is the culmination of a six-month project originally planned as an eleven-part series, but expanded to twelve. Authored by Dmitry Sviridkin and edited by Andrey Karpov, this work is slated for future print publication.
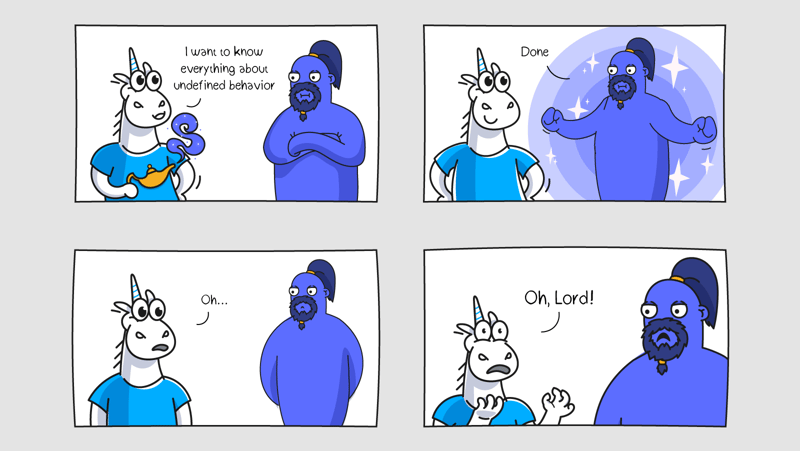
The book delves into a wide range of topics, including:
-
Introduction: Defining undefined behavior and its consequences; exploring narrowing conversions and implicit type conversions.
-
Integer and Floating-Point Issues: Examining signed integer overflow, floating-point peculiarities, integer promotion, and the nuances of
char
and sign extension.
-
Memory Management and References: Addressing dangling references,
string_view
, range-based for loops, self-references, and std::vector
reference invalidation.
-
Lambda Functions and Mutability: Analyzing lambda function capture lists, tuples, unexpected mutability, implicit references, use-after-move, and lifetime extension.
-
Parsing and Move Semantics: Tackling the Most Vexing Parse, non-constant constants, move semantics,
std::enable_if_t
vs. std::void_t
, and forgotten return
statements.
-
Operators and I/O: Covering ellipsis and functions,
operator[]
, the challenges of iostreams
debugging, the comma operator, function-try-blocks, and zero-sized types.
-
Strings and Smart Pointers: Discussing null-terminated strings,
std::shared_ptr
, explicit type conversion, and safe standard function passing.
-
Loops, Recursion, and Buffers: Exploring infinite loops, the halting problem, recursion,
noexcept
handling, and buffer overflows.
-
RAII, Pointers, and Initialization: Comparing (N)RVO and RAII, examining null pointer dereferencing, the static initialization order fiasco, static inline functions, ODR violations, and reserved names.
-
Types, Variables, and Ranges: Delving into trivial types and ABI, uninitialized variables, C 20 unbounded ranges, non-virtual yet virtual functions, and VLAs.
-
Pointers, Concurrency, and Signals: Addressing invalid pointers, placement new for arrays, data races, mutex deadlocks, signal safety, and best practices for deadlock avoidance.
-
Vectors, Alignment, and Static Analysis: Analyzing
std::vector::reserve
and std::vector::resize
, unaligned references, object lifetimes, static analysis and undefined behavior, and concluding remarks.
Licensing and Usage:
This work is available for reference, with proper attribution. Copying or reproduction requires the author's permission (dmisvrl1@gmail.com). Commercial use or fee-based instruction is prohibited.
About the Authors:
-
Dmitry Sviridkin: A software engineer at AWS with extensive experience in high-performance software development and a background in teaching C and Linux system programming.
-
Andrey Karpov: A co-founder of the PVS-Studio project with over 15 years of experience in static code analysis and software quality, and a Microsoft MVP award recipient.
The above is the detailed content of C programmers guide to undefined behavior. For more information, please follow other related articles on the PHP Chinese website!