Python Regular Expressions
Odoma processing and user input verification are key links in programming, especially when building applications that require user interaction. Python provides powerful tools to complete these tasks. The regular expression (Regex) is one of the most effective tools for pattern matching. This article will explore the world of Python abnormalities and regular expressions, explain their importance, provide practical examples, and ensure that your code is strong and efficient.
Understand Python abnormalities
Before studying in -depth research, we must understand the concepts of abnormal abnormalities in Python. Abnormal is an event that interrupts the normal process. These events are usually caused by errors, such as trying to remove documents that do not exist with zero or access.
Basic abnormal treatment
Python provides TRY-EXCEPT blocks to handle abnormalities. This is a simple example:
In this example, if the user tries to remove the non -integer with zero or input, the program will capture abnormalities and handle it elegantly.
try: 分子 = int(input("请输入分子:")) 分母 = int(input("请输入分母:")) 结果 = 分子 / 分母 print(f"结果是 {结果}") except ZeroDivisionError: print("错误:不能除以零!") except ValueError: print("错误:请输入有效的整数!")
The importance of abnormal treatment
In software development, the correct abnormal processing is critical because it can prevent the procedure from accidentally collapse. It also provides a way to convey errors to users to help them understand what problems and how to solve it.
Deeper regular expression
regular expression, usually called "regex", is a character sequence defined by the search mode. They are very useful for verification and operation of string in Python.
Why use regular expressions?
Consider a scene that needs to verify the email address. If there is no regular expression, you may eventually write a large number of code to check the existence of the "@" symbol and period ("."). However, even this may not be enough to ensure that the email address is effective. Regular expressions allow you to write simple and powerful verification rules.
Basic regular expression example
Let's start with a simple regular expression example to verify the email address:
In this example, R "^w @w .w $" is a regular expression mode. It matches the basic email structure:
import re 邮箱 = input("您的邮箱地址是?").strip() if re.search(r"^\w+@\w+\.\w+$", 邮箱): print("有效的邮箱地址") else: print("无效的邮箱地址")
^ Make sure the mode starts from the beginning of the string. W matchs one or more word characters (letters, numbers, and lower lines). @ "@" Symbol. . Matching the period ("."). The $ ensures the end of the pattern at the end of the string.
Advanced email verification
The basic regular expression above may not capture all invalid email formats. For example, it allows multiple "@" symbols, which is not allowed in an effective email address. This is an improved version:
This regular expression mode is more robust:
import re 邮箱 = input("您的邮箱地址是?").strip() if re.search(r"^[^@]+@[^@]+\.\w{2,}$", 邮箱): print("有效的邮箱地址") else: print("无效的邮箱地址")
- [^@] Make sure that the content before and after the "@" symbol does not contain another "@".
- W {2,} ensure that there are at least two characters in the domain name part (after the period).
Treatment of common traps
The regular expression function is powerful, but they may be tricky. For example, the period in regular expressions (.) Match any characters other than changing the character. To match the actual period in the string, you need to use the back slope (.) To make a righteousness. In addition, the regular expression mode distinguishes the case, but you can use the RE.IGNORCASE logo to deal with this problem.
<小> Scholarship of the case
try: 分子 = int(input("请输入分子:")) 分母 = int(input("请输入分母:")) 结果 = 分子 / 分母 print(f"结果是 {结果}") except ZeroDivisionError: print("错误:不能除以零!") except ValueError: print("错误:请输入有效的整数!")
Extract user information <提>
regular expression is not only used to verify; it is also used to extract the specific parts of the string. Suppose you want to extract the user name from Twitter URL:
This code extract Twitter username by matching the relevant part of the URL. (W) capture the username, it is the second match group (Group (2)).
import re 邮箱 = input("您的邮箱地址是?").strip() if re.search(r"^\w+@\w+\.\w+$", 邮箱): print("有效的邮箱地址") else: print("无效的邮箱地址")
Optimize the code with regular expression
Use the original string
When processing regular expressions, it is best to use the original string (R "). The primitive string looks at the back slope as the text character to prevent accidental transition sequences. For example:
Avoid common errors
import re 邮箱 = input("您的邮箱地址是?").strip() if re.search(r"^[^@]+@[^@]+\.\w{2,}$", 邮箱): print("有效的邮箱地址") else: print("无效的邮箱地址")
Make the mode too complicated: starting from simple, only increase complexity when necessary.
- ignore performance: regular expressions may be slow when using complex mode or large text. By minimizing the retrospective optimization.
- Readability: Maintain readability by decomposing complex regular expression patterns by using annotations or detailed patterns to maintain readability.
- Actual application: Clean up the user input
Users usually enter data in unexpected formats. Regular expressions can help standardize these inputs. Consider a program for a format name:
This code will be re -sorted as "named surname" in the name "Surname, Name" format.
import re 邮箱 = input("您的邮箱地址是?").strip() if re.search(r"^\w+@\w+\.\w+$", 邮箱, re.IGNORECASE): print("有效的邮箱地址") else: print("无效的邮箱地址")
SEO optimization and actual use
Regular expression can also play a role in SEO. For example, they can be used for network capture, extract meta tags or specific content from HTML to ensure that the network content is optimized for search engines.
This example is the description of the element from the HTML mark, which is essential for SEO.
import re 网址 = input("请输入您的 Twitter 个人资料网址:").strip() 匹配 = re.search(r"^https?://(www\.)?twitter\.com/(\w+)", 网址) if 匹配: 用户名 = 匹配.group(2) print(f"用户名:{用户名}") else: print("无效的网址")
Conclusion
Understand and master the regular expression in Python to open a world full of possibilities, from simple verification to complex text processing tasks. Combined with the correct abnormal treatment, you can create a strong, efficient and user -friendly application. Continue to try different regular expression patterns. Over time, you will find that they are indispensable tools in programming tool packs.
By mastering these concepts, you can not only write more concise and more efficient code, but also obtain the advantages of developing applications that can handle real -world input elegantly.The above is the detailed content of Python Regular Expressions. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
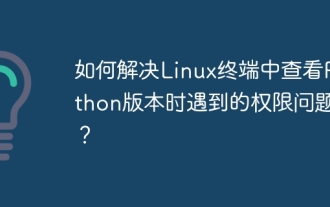
Solution to permission issues when viewing Python version in Linux terminal When you try to view Python version in Linux terminal, enter python...
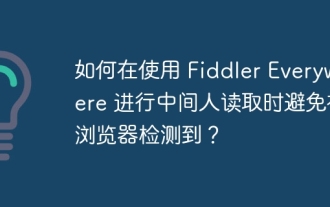
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...
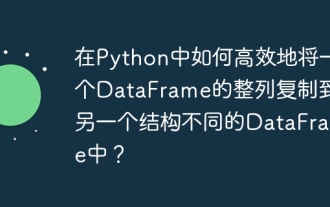
When using Python's pandas library, how to copy whole columns between two DataFrames with different structures is a common problem. Suppose we have two Dats...
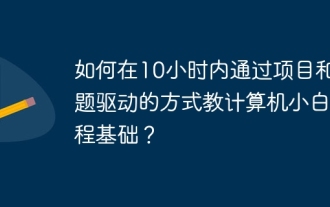
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
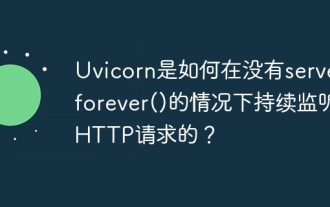
How does Uvicorn continuously listen for HTTP requests? Uvicorn is a lightweight web server based on ASGI. One of its core functions is to listen for HTTP requests and proceed...
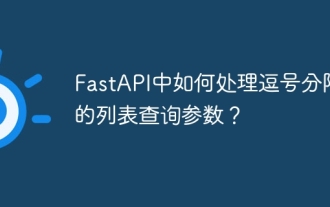
Fastapi ...
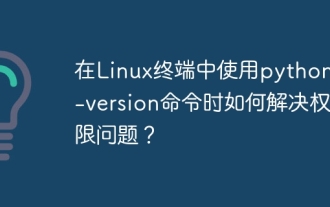
Using python in Linux terminal...
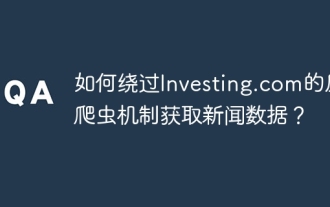
Understanding the anti-crawling strategy of Investing.com Many people often try to crawl news data from Investing.com (https://cn.investing.com/news/latest-news)...
