


How to Create a Disappearing Watermark in a WPF TextBox Using Attached Properties?
This code creates a disappearing watermark in a WPF TextBox using attached properties. Let's refactor and improve the code for clarity and maintainability. The original code has some redundancy and could be simplified.
Watermark in TextBox
This improved example demonstrates creating a placeholder text (watermark) in a TextBox that disappears when the user starts typing. We'll utilize attached properties for a clean and reusable solution.
1. Improved Attached Property and Watermark Service:
public static class WatermarkService { public static readonly DependencyProperty WatermarkProperty = DependencyProperty.RegisterAttached("Watermark", typeof(object), typeof(WatermarkService), new FrameworkPropertyMetadata(null, OnWatermarkChanged)); public static object GetWatermark(DependencyObject obj) => obj.GetValue(WatermarkProperty); public static void SetWatermark(DependencyObject obj, object value) => obj.SetValue(WatermarkProperty, value); private static void OnWatermarkChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { if (!(d is TextBox textBox)) return; textBox.Loaded += TextBox_Loaded; textBox.TextChanged += TextBox_TextChanged; textBox.GotFocus += TextBox_GotFocus; } private static void TextBox_Loaded(object sender, RoutedEventArgs e) => UpdateWatermarkVisibility((TextBox)sender); private static void TextBox_TextChanged(object sender, TextChangedEventArgs e) => UpdateWatermarkVisibility((TextBox)sender); private static void TextBox_GotFocus(object sender, RoutedEventArgs e) => UpdateWatermarkVisibility((TextBox)sender); private static void UpdateWatermarkVisibility(TextBox textBox) { RemoveWatermark(textBox); if (string.IsNullOrEmpty(textBox.Text)) { ShowWatermark(textBox); } } private static void RemoveWatermark(TextBox textBox) { var layer = AdornerLayer.GetAdornerLayer(textBox); if (layer != null) { var adorners = layer.GetAdorners(textBox); if (adorners != null) { foreach (var adorner in adorners.OfType<WatermarkAdorner>()) { layer.Remove(adorner); } } } } private static void ShowWatermark(TextBox textBox) { var layer = AdornerLayer.GetAdornerLayer(textBox); if (layer != null) { layer.Add(new WatermarkAdorner(textBox, GetWatermark(textBox))); } } }
2. WatermarkAdorner Class (Minor Improvements):
internal class WatermarkAdorner : Adorner { private readonly ContentPresenter contentPresenter; public WatermarkAdorner(UIElement adornedElement, object watermark) : base(adornedElement) { IsHitTestVisible = false; contentPresenter = new ContentPresenter { Content = watermark, Opacity = 0.5 }; // Removed unnecessary margin setting; let the watermark style handle positioning. } protected override int VisualChildrenCount => 1; protected override Visual GetVisualChild(int index) => contentPresenter; protected override Size MeasureOverride(Size constraint) { contentPresenter.Measure(AdornedElement.RenderSize); return AdornedElement.RenderSize; } protected override Size ArrangeOverride(Size finalSize) { contentPresenter.Arrange(new Rect(finalSize)); return finalSize; } }
3. XAML Usage:
<TextBox x:Name="SearchTextBox"> <WatermarkService.Watermark> <TextBlock>Type here to search text</TextBlock> </WatermarkService.Watermark> </TextBox>
Improvements:
-
Simplified Event Handling: The revised code uses only
Loaded
,TextChanged
, andGotFocus
events for the TextBox, making it more concise and easier to understand. TheUpdateWatermarkVisibility
method handles the logic for showing and hiding the watermark based on the TextBox's text. - Removed Redundancy: The original code had several duplicated code blocks. This version streamlines the logic.
-
Improved Type Safety: The code now explicitly checks for
TextBox
type, avoiding potential casting exceptions. -
LINQ Usage: Using
OfType<WatermarkAdorner>()
simplifies the removal of adorners. - Clearer Structure: The code is better organized into logical sections.
-
Removed Unnecessary Margin: The margin is better handled through styling the
TextBlock
within the watermark.
This improved version is more efficient, readable, and maintainable while achieving the same functionality. Remember to add appropriate styling to the TextBlock
within the Watermark
to control its appearance and positioning within the TextBox
.
The above is the detailed content of How to Create a Disappearing Watermark in a WPF TextBox Using Attached Properties?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


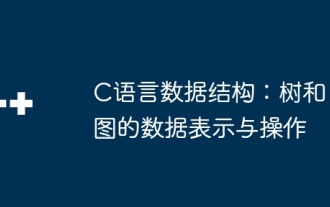
C language data structure: The data representation of the tree and graph is a hierarchical data structure consisting of nodes. Each node contains a data element and a pointer to its child nodes. The binary tree is a special type of tree. Each node has at most two child nodes. The data represents structTreeNode{intdata;structTreeNode*left;structTreeNode*right;}; Operation creates a tree traversal tree (predecision, in-order, and later order) search tree insertion node deletes node graph is a collection of data structures, where elements are vertices, and they can be connected together through edges with right or unrighted data representing neighbors.
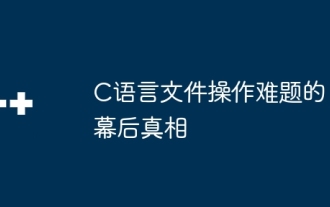
The truth about file operation problems: file opening failed: insufficient permissions, wrong paths, and file occupied. Data writing failed: the buffer is full, the file is not writable, and the disk space is insufficient. Other FAQs: slow file traversal, incorrect text file encoding, and binary file reading errors.
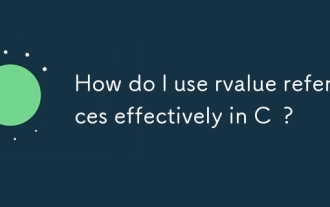
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)
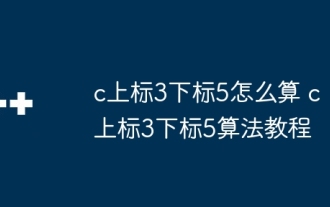
The calculation of C35 is essentially combinatorial mathematics, representing the number of combinations selected from 3 of 5 elements. The calculation formula is C53 = 5! / (3! * 2!), which can be directly calculated by loops to improve efficiency and avoid overflow. In addition, understanding the nature of combinations and mastering efficient calculation methods is crucial to solving many problems in the fields of probability statistics, cryptography, algorithm design, etc.
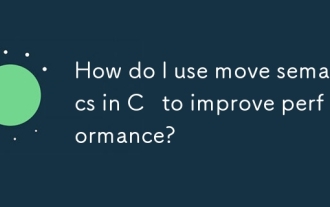
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
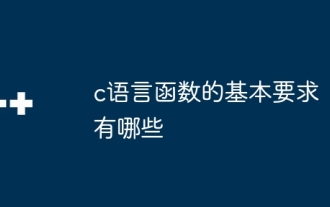
C language functions are the basis for code modularization and program building. They consist of declarations (function headers) and definitions (function bodies). C language uses values to pass parameters by default, but external variables can also be modified using address pass. Functions can have or have no return value, and the return value type must be consistent with the declaration. Function naming should be clear and easy to understand, using camel or underscore nomenclature. Follow the single responsibility principle and keep the function simplicity to improve maintainability and readability.
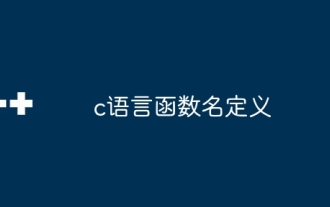
The C language function name definition includes: return value type, function name, parameter list and function body. Function names should be clear, concise and unified in style to avoid conflicts with keywords. Function names have scopes and can be used after declaration. Function pointers allow functions to be passed or assigned as arguments. Common errors include naming conflicts, mismatch of parameter types, and undeclared functions. Performance optimization focuses on function design and implementation, while clear and easy-to-read code is crucial.
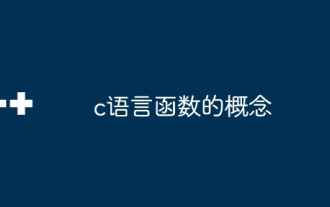
C language functions are reusable code blocks. They receive input, perform operations, and return results, which modularly improves reusability and reduces complexity. The internal mechanism of the function includes parameter passing, function execution, and return values. The entire process involves optimization such as function inline. A good function is written following the principle of single responsibility, small number of parameters, naming specifications, and error handling. Pointers combined with functions can achieve more powerful functions, such as modifying external variable values. Function pointers pass functions as parameters or store addresses, and are used to implement dynamic calls to functions. Understanding function features and techniques is the key to writing efficient, maintainable, and easy to understand C programs.
