How Can I Use LINQ to Query a DataTable Effectively?
Mastering LINQ Queries on DataTables
LINQ's versatility extends to various data sources, but querying DataTables requires a specific approach. Directly applying LINQ to a DataTable's rows often leads to errors. For instance:
var results = from myRow in myDataTable where results.Field("RowNo") == 1 select results;
This code will fail.
The AsEnumerable() Solution
The key is to use the AsEnumerable()
extension method. This converts the DataTable's Rows
collection into an IEnumerable<DataRow>
, enabling seamless LINQ integration. The correct approach is:
var results = from myRow in myDataTable.AsEnumerable() where myRow.Field<int>("RowNo") == 1 select myRow;
Important Notes:
- Ensure you've added a reference to
System.Data.DataSetExtensions
in your project to useAsEnumerable()
. - To convert the resulting
IEnumerable<DataRow>
back to a DataTable, employ theCopyToDataTable()
extension method.
Lambda Expressions: A More Concise Approach
Lambda expressions offer a more compact syntax:
var result = myDataTable .AsEnumerable() .Where(myRow => myRow.Field<int>("RowNo") == 1);
By employing these methods, developers can harness the power of LINQ for efficient data manipulation and filtering within their .NET applications when working with DataTables.
The above is the detailed content of How Can I Use LINQ to Query a DataTable Effectively?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


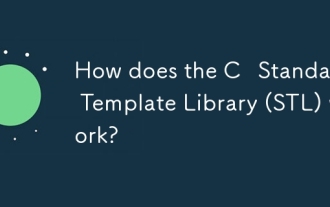
This article explains the C Standard Template Library (STL), focusing on its core components: containers, iterators, algorithms, and functors. It details how these interact to enable generic programming, improving code efficiency and readability t
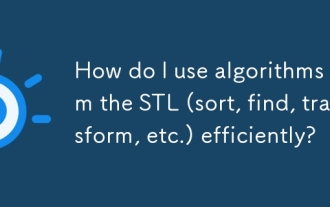
This article details efficient STL algorithm usage in C . It emphasizes data structure choice (vectors vs. lists), algorithm complexity analysis (e.g., std::sort vs. std::partial_sort), iterator usage, and parallel execution. Common pitfalls like
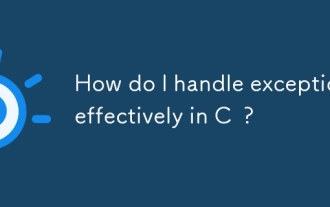
This article details effective exception handling in C , covering try, catch, and throw mechanics. It emphasizes best practices like RAII, avoiding unnecessary catch blocks, and logging exceptions for robust code. The article also addresses perf
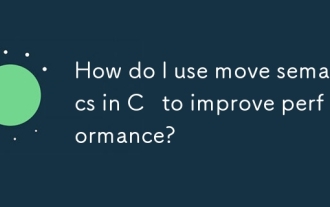
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
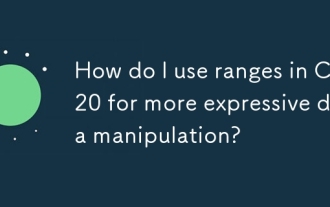
C 20 ranges enhance data manipulation with expressiveness, composability, and efficiency. They simplify complex transformations and integrate into existing codebases for better performance and maintainability.
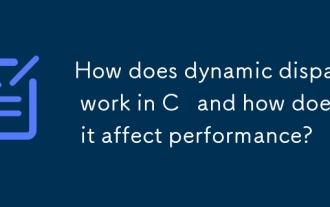
The article discusses dynamic dispatch in C , its performance costs, and optimization strategies. It highlights scenarios where dynamic dispatch impacts performance and compares it with static dispatch, emphasizing trade-offs between performance and
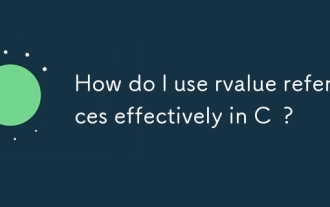
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)
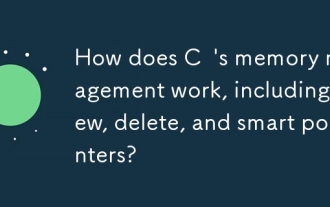
C memory management uses new, delete, and smart pointers. The article discusses manual vs. automated management and how smart pointers prevent memory leaks.
