How to Design a Thread-Safe Blocking Queue in .NET?
In multi -threaded environment, when multiple threads operate the same queue, efficient queue management is essential. This article discusses how to create a blocking queue in the .NET to meet this demand.
The limitations of existing implementation
The code fragment provided in the question shows the basic implementation of a blocking queue. However, it lacks appropriate synchronization mechanisms and may lead to thread security issues.
Improvement method
In order to solve the limitations of the original implementation, please consider the following improved version:
In this improved version, the
class SizeQueue<T> { private readonly Queue<T> queue = new Queue<T>(); private readonly int maxSize; private bool closing; // 添加关闭标志 public SizeQueue(int maxSize) { this.maxSize = maxSize; } public void Enqueue(T item) { lock (queue) { while (queue.Count >= maxSize && !closing) // 检查关闭标志 { Monitor.Wait(queue); } if(closing) return; // 如果队列已关闭,则直接返回 queue.Enqueue(item); if (queue.Count == 1) { Monitor.PulseAll(queue); } } } public T Dequeue() { lock (queue) { while (queue.Count == 0 && !closing) // 检查关闭标志 { Monitor.Wait(queue); } if(closing && queue.Count == 0) throw new InvalidOperationException("Queue is closed and empty."); // 队列关闭且为空 T item = queue.Dequeue(); if (queue.Count == maxSize - 1) { Monitor.PulseAll(queue); } return item; } } public void Close() { lock (queue) { closing = true; Monitor.PulseAll(queue); } } public bool TryDequeue(out T value) { lock (queue) { while (queue.Count == 0) { if (closing) { value = default(T); return false; } Monitor.Wait(queue); } value = queue.Dequeue(); if (queue.Count == maxSize - 1) { Monitor.PulseAll(queue); } return true; } } }
Class is used to block and lift the blocking thread according to the status of the queue. In addition, the logo and lock
methods are added to the elegant closure of the queue, and the Monitor
method is used to handle the queue as empty. closing
Close()
further consideration TryDequeue()
These enhanced functions allow the reading thread in an orderly manner when the queue is closed. The improved code increases the check of the queue closure, and processes the situation of the queue closure and empty, avoiding abnormalities.
The above is the detailed content of How to Design a Thread-Safe Blocking Queue in .NET?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


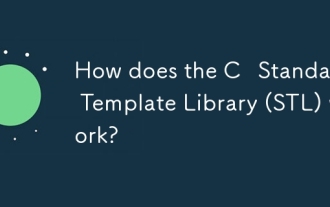
This article explains the C Standard Template Library (STL), focusing on its core components: containers, iterators, algorithms, and functors. It details how these interact to enable generic programming, improving code efficiency and readability t
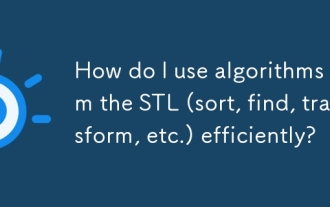
This article details efficient STL algorithm usage in C . It emphasizes data structure choice (vectors vs. lists), algorithm complexity analysis (e.g., std::sort vs. std::partial_sort), iterator usage, and parallel execution. Common pitfalls like
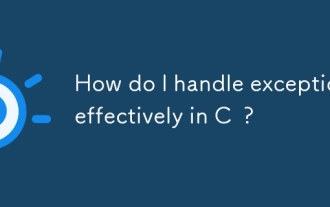
This article details effective exception handling in C , covering try, catch, and throw mechanics. It emphasizes best practices like RAII, avoiding unnecessary catch blocks, and logging exceptions for robust code. The article also addresses perf
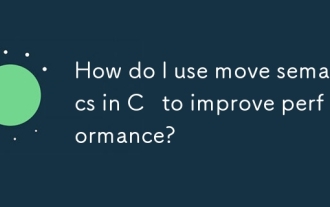
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
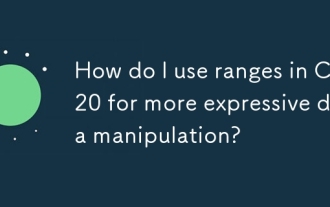
C 20 ranges enhance data manipulation with expressiveness, composability, and efficiency. They simplify complex transformations and integrate into existing codebases for better performance and maintainability.
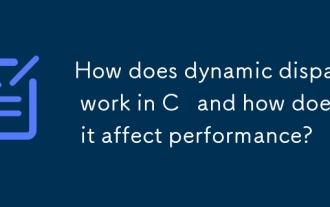
The article discusses dynamic dispatch in C , its performance costs, and optimization strategies. It highlights scenarios where dynamic dispatch impacts performance and compares it with static dispatch, emphasizing trade-offs between performance and
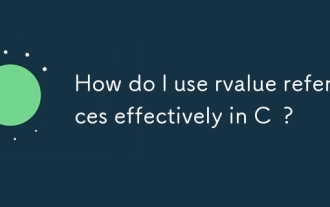
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)
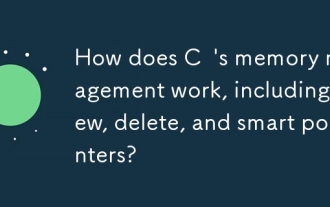
C memory management uses new, delete, and smart pointers. The article discusses manual vs. automated management and how smart pointers prevent memory leaks.
