How Can I Perform Multiple Asynchronous WCF Calls Concurrently in C#?
Efficiently Handling Multiple Asynchronous WCF Calls with TPL Dataflow
When dealing with multiple asynchronous WCF calls in C#, Parallel.ForEach
isn't ideal because it doesn't directly support await
. A more robust solution is to leverage the Task Parallel Library's Dataflow (TPL Dataflow) which excels at managing asynchronous operations.
This example demonstrates how to rewrite code using TPL Dataflow for concurrent asynchronous WCF calls:
// Create a TransformBlock to asynchronously fetch Customer data. var getCustomerBlock = new TransformBlock<string, Customer>( async id => { ICustomerRepo repo = new CustomerRepo(); return await repo.GetCustomer(id); }, new ExecutionDataflowBlockOptions { MaxDegreeOfParallelism = DataflowBlockOptions.Unbounded // Adjust as needed }); // Create an ActionBlock to process each retrieved Customer. var writeCustomerBlock = new ActionBlock<Customer>(c => Console.WriteLine(c.ID)); // Link the blocks; PropagateCompletion ensures the pipeline finishes. getCustomerBlock.LinkTo(writeCustomerBlock, new DataflowLinkOptions { PropagateCompletion = true }); // Post IDs to the TransformBlock. foreach (var id in ids) { getCustomerBlock.Post(id); } // Signal completion and wait for all processing to finish. getCustomerBlock.Complete(); writeCustomerBlock.Completion.Wait();
Key Improvements:
-
Asynchronous Processing: TPL Dataflow inherently handles asynchronous operations (
await
) within theTransformBlock
. -
Controlled Parallelism:
MaxDegreeOfParallelism
allows you to control the level of concurrency, preventing resource exhaustion with large datasets.DataflowBlockOptions.Unbounded
allows for maximum parallelism, but consider setting a limit for better resource management in production environments. - Improved Structure: The code is more modular and readable, separating data retrieval and processing into distinct blocks.
- Streaming Output: Results are processed and written to the console as they become available, rather than waiting for all operations to complete.
- Error Handling: (Not shown, but easily added) TPL Dataflow provides mechanisms for handling exceptions that may occur during asynchronous operations.
This approach offers a more efficient and manageable way to handle numerous concurrent asynchronous WCF calls compared to using Parallel.ForEach
with nested await
calls. Remember to adjust MaxDegreeOfParallelism
based on your system's resources and the size of your ids
collection.
The above is the detailed content of How Can I Perform Multiple Asynchronous WCF Calls Concurrently in C#?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


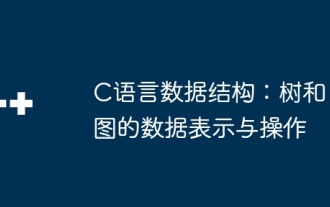
C language data structure: The data representation of the tree and graph is a hierarchical data structure consisting of nodes. Each node contains a data element and a pointer to its child nodes. The binary tree is a special type of tree. Each node has at most two child nodes. The data represents structTreeNode{intdata;structTreeNode*left;structTreeNode*right;}; Operation creates a tree traversal tree (predecision, in-order, and later order) search tree insertion node deletes node graph is a collection of data structures, where elements are vertices, and they can be connected together through edges with right or unrighted data representing neighbors.
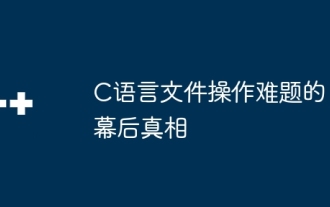
The truth about file operation problems: file opening failed: insufficient permissions, wrong paths, and file occupied. Data writing failed: the buffer is full, the file is not writable, and the disk space is insufficient. Other FAQs: slow file traversal, incorrect text file encoding, and binary file reading errors.
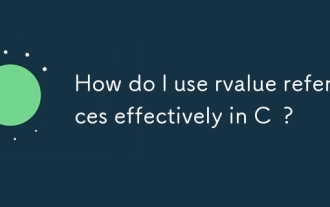
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)
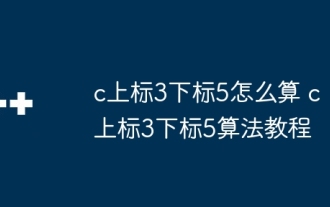
The calculation of C35 is essentially combinatorial mathematics, representing the number of combinations selected from 3 of 5 elements. The calculation formula is C53 = 5! / (3! * 2!), which can be directly calculated by loops to improve efficiency and avoid overflow. In addition, understanding the nature of combinations and mastering efficient calculation methods is crucial to solving many problems in the fields of probability statistics, cryptography, algorithm design, etc.
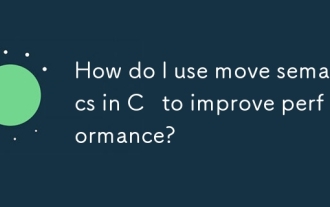
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
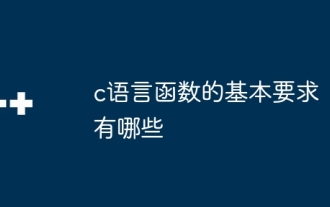
C language functions are the basis for code modularization and program building. They consist of declarations (function headers) and definitions (function bodies). C language uses values to pass parameters by default, but external variables can also be modified using address pass. Functions can have or have no return value, and the return value type must be consistent with the declaration. Function naming should be clear and easy to understand, using camel or underscore nomenclature. Follow the single responsibility principle and keep the function simplicity to improve maintainability and readability.
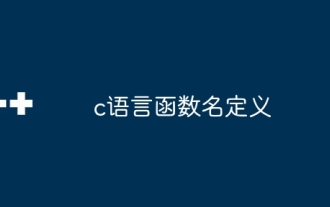
The C language function name definition includes: return value type, function name, parameter list and function body. Function names should be clear, concise and unified in style to avoid conflicts with keywords. Function names have scopes and can be used after declaration. Function pointers allow functions to be passed or assigned as arguments. Common errors include naming conflicts, mismatch of parameter types, and undeclared functions. Performance optimization focuses on function design and implementation, while clear and easy-to-read code is crucial.
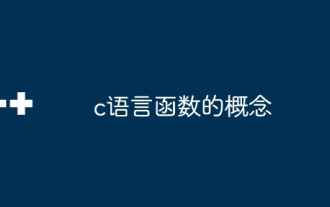
C language functions are reusable code blocks. They receive input, perform operations, and return results, which modularly improves reusability and reduces complexity. The internal mechanism of the function includes parameter passing, function execution, and return values. The entire process involves optimization such as function inline. A good function is written following the principle of single responsibility, small number of parameters, naming specifications, and error handling. Pointers combined with functions can achieve more powerful functions, such as modifying external variable values. Function pointers pass functions as parameters or store addresses, and are used to implement dynamic calls to functions. Understanding function features and techniques is the key to writing efficient, maintainable, and easy to understand C programs.
