PHP program to find the perimeter of rectangle
Problem Description
Given the length and width of a rectangle, we need to implement a PHP program to calculate its perimeter. A rectangle is an enclosed two-dimensional figure with 4 sides. The opposite sides are equal and parallel, and the angle formed by adjacent sides is equal to 90 degrees. The perimeter is the sum of all sides; in other words, the perimeter is twice the sum of length and width. In this tutorial, we will learn how to find the perimeter of a given rectangle in PHP using different methods.
Rectangle perimeter formula
The formula for the circumference of the rectangle is as follows:
$${perimeter = 2 × (length and width)}$$
Of:
- Long - Horizontal distance.
- Width - Vertical distance.
Example
Let's understand the problem description with some input and output examples.
Enter
<code>长 = 10 单位 宽 = 5 单位</code>
Output
<code>30 单位</code>
Explanation
Calculate the circumference using the formula: 2 × (length and width).
<code>周长 = 2 × (10 + 5) = 2 × (15) = 30 单位</code>
Enter
<code>长 = 20 单位 宽 = 0 单位</code>
Output
<code>0</code>
Explanation
If the length or width of any dimension is zero, the two-dimensional figure does not exist. Therefore, a non-existent rectangular figure has no perimeter.
Enter
<code>长 = 15 单位 宽 = 10 单位</code>
Output
<code>50 单位</code>
Explanation
Calculate the circumference using formula: 2 × (length and width)
<code>2 × (15 + 10) 2 × (25) 50 单位</code>
Method 1: Direct formula method
We use the direct formula method to calculate the perimeter of a rectangle using PHP. The formula for calculating the circumference of a rectangle is: length, width, length, width
Formula for rectangle perimeter = 2 × (length and width). Return the result after calculating the perimeter.
Implementation steps
Accounting the following steps:
- Prefer length and width as input parameters.
- Calculate the circumference of a rectangle using formula: 2 × (length and width)
- After the calculation is completed, print the result.
Example
The following example is an implementation in PHP following the implementation steps discussed above.
<?php $length = 10; $width = 5; // 使用公式计算周长 $perimeter = 2 * ($length + $width); // 输出 echo "长为 $length,宽为 $width 的矩形的周长是:$perimeter<br>"; ?>
Output
<code>长为 10,宽为 5 的矩形的周长是:30</code>
Time complexity: O(1), constant time Space complexity: O(1), constant space.
Method 2: Use the function
We will use a function to calculate the perimeter of the rectangle. The logic and formula for calculating the circumference of a rectangle are the same. We will use a function so that the code can be reused later by simply changing the value.
Implementation steps
Accounting the following steps:
- We create a function to calculate the perimeter, which uses the formula ${2 * ($length $width)}$ to calculate the perimeter of the rectangle.
- We pass the input value length and width.
- Finally, we output the result.
Example
The following example is an implementation in PHP following the implementation steps discussed above.
<code>长 = 10 单位 宽 = 5 单位</code>
Output
<code>30 单位</code>
Time complexity: O(1), a single function call. Spatial complexity: O(1), because the above function only uses some variables.
The above is the detailed content of PHP program to find the perimeter of rectangle. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


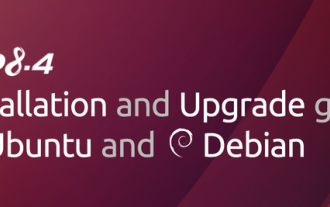
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
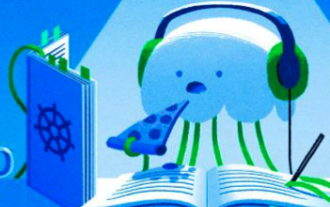
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
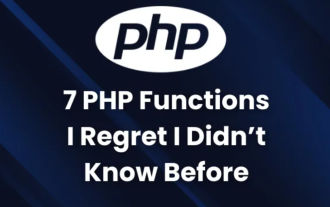
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
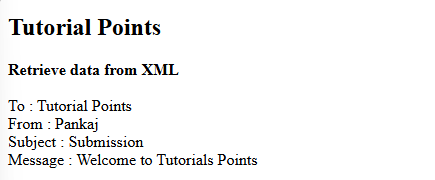
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
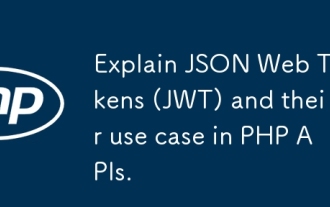
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
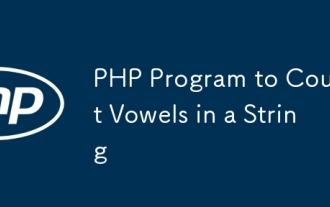
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
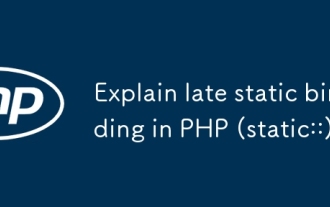
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
