Comparing Streams to Loops in Java
Java Streams offer a concise and efficient way to process collections of data. This article compares Streams to traditional loops, highlighting their strengths and weaknesses. Streams employ a pipeline architecture, chaining operations like filter()
, map()
, forEach()
, and collect()
for data manipulation. A stream's lifecycle begins with a data source, proceeds through a pipeline of operations, and concludes with a terminal operation.
Performance Comparison:
Benchmarking reveals performance variations depending on the specific operation. While simple loops might sometimes outperform streams in certain scenarios (as shown below), streams often provide more readable and maintainable code, especially for complex data transformations.
<code>Benchmark Is Here Mode Cnt Score Error Units ForLoopPerformanceTest.usingForEachLoop thrpt 20 259.008 ± 17.888 ops/s ForLoopPerformanceTest.usingIterator thrpt 20 256.016 ± 10.342 ops/s ForLoopPerformanceTest.usingSimpleForLoop thrpt 20 495.308 ± 12.866 ops/s ForLoopPerformanceTest.usingStream thrpt 20 257.174 ± 15.880 ops/s</code>
Algorithmic Approach:
Comparing streams and loops involves analyzing the steps involved in data processing. Both approaches generally follow these steps:
- Initialization: Define the data source (e.g., array, list).
- Iteration/Processing: Iterate through the data (loop) or create a stream and apply operations (stream).
- Transformation (Optional): Modify data elements (e.g., filtering, mapping).
- Aggregation (Optional): Collect results (e.g., summing, collecting to a list).
- Termination: End the process and return results.
Illustrative Syntax:
Streams provide a declarative style, while loops are imperative. Here's a comparison:
Stream Example:
List<Person> persons = List.of(...); // Sample Person class with name, age, gender List<String> namesOfAdults = persons.stream() .filter(p -> p.getAge() > 18) .map(Person::getName) .collect(Collectors.toList());
Loop Example (Enhanced for loop):
List<Person> persons = List.of(...); List<String> namesOfAdults = new ArrayList<>(); for (Person p : persons) { if (p.getAge() > 18) { namesOfAdults.add(p.getName()); } }
Exception Handling:
When working with streams (especially those involving file I/O), robust exception handling is crucial. The try-catch-finally
block ensures resources are released properly, even if exceptions occur.
Example with finally
block:
try { // Stream operations here... } catch (IOException e) { // Handle exception } finally { // Close resources }
Conclusion:
Java Streams offer a functional approach to data processing, often leading to more concise and readable code. While performance may vary depending on the specific task, streams generally provide a superior approach for complex data manipulations, enhancing code maintainability and readability. Careful consideration of exception handling is vital when working with streams, especially when dealing with external resources.
The above is the detailed content of Comparing Streams to Loops in Java. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics




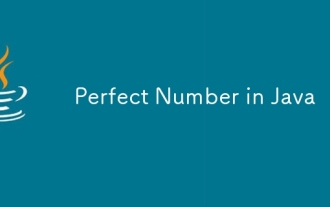
Guide to Perfect Number in Java. Here we discuss the Definition, How to check Perfect number in Java?, examples with code implementation.
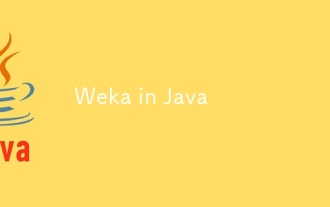
Guide to Weka in Java. Here we discuss the Introduction, how to use weka java, the type of platform, and advantages with examples.
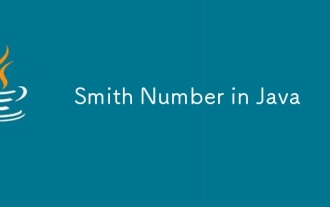
Guide to Smith Number in Java. Here we discuss the Definition, How to check smith number in Java? example with code implementation.

In this article, we have kept the most asked Java Spring Interview Questions with their detailed answers. So that you can crack the interview.
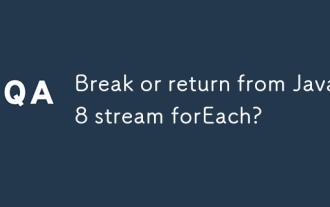
Java 8 introduces the Stream API, providing a powerful and expressive way to process data collections. However, a common question when using Stream is: How to break or return from a forEach operation? Traditional loops allow for early interruption or return, but Stream's forEach method does not directly support this method. This article will explain the reasons and explore alternative methods for implementing premature termination in Stream processing systems. Further reading: Java Stream API improvements Understand Stream forEach The forEach method is a terminal operation that performs one operation on each element in the Stream. Its design intention is
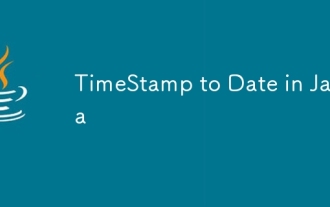
Guide to TimeStamp to Date in Java. Here we also discuss the introduction and how to convert timestamp to date in java along with examples.
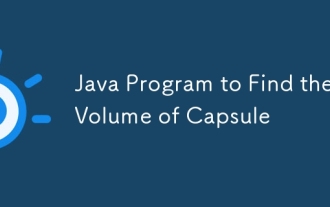
Capsules are three-dimensional geometric figures, composed of a cylinder and a hemisphere at both ends. The volume of the capsule can be calculated by adding the volume of the cylinder and the volume of the hemisphere at both ends. This tutorial will discuss how to calculate the volume of a given capsule in Java using different methods. Capsule volume formula The formula for capsule volume is as follows: Capsule volume = Cylindrical volume Volume Two hemisphere volume in, r: The radius of the hemisphere. h: The height of the cylinder (excluding the hemisphere). Example 1 enter Radius = 5 units Height = 10 units Output Volume = 1570.8 cubic units explain Calculate volume using formula: Volume = π × r2 × h (4
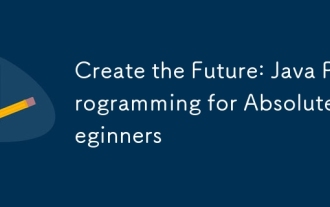
Java is a popular programming language that can be learned by both beginners and experienced developers. This tutorial starts with basic concepts and progresses through advanced topics. After installing the Java Development Kit, you can practice programming by creating a simple "Hello, World!" program. After you understand the code, use the command prompt to compile and run the program, and "Hello, World!" will be output on the console. Learning Java starts your programming journey, and as your mastery deepens, you can create more complex applications.
