Returning even numbers from a stack in java
Stack in Java
Stack is a last-in-first-out (LIFO) data structure. As shown below, the last book placed on the stack is the first one removed, and the first book placed on the stack is the last one removed.
In Java, you can create an integer stack by importing the java.util.Stack
package and calling the Stack()
constructor. The integer object can be pushed into the stack using the push()
method. The following code snippet provides an example.
Example
The following is a sample program:
import java.util.Stack; public class Testing { public static void main(String[] args) { Stack<Integer> numbers = new Stack<>(); // 使用Integer,而不是integer numbers.push(12); numbers.push(1); numbers.push(3); numbers.push(15); numbers.push(4); System.out.println(numbers); } }
The output of the above program is as follows:
<code>[12, 1, 3, 15, 4]</code>
Return even number from stack
You can use the get()
and pop()
methods to access elements of the stack in Java. The peek()
method can access it without removing the top element of the stack. We can use the pop()
method to access integers in the stack because it is more in line with the function of the stack as a LIFO data structure.
Example
The following is an example program for retrieving even numbers from the stack. In this program:
- Use the
empty()
method to test whether the stack is empty in awhile
loop. - If the stack is not empty, pop up the number from the top of the stack and store it as an integer.
- Use the modulo operator to check if the number is even.
- If the number is even, output it to the screen.
import java.util.Stack; public class Example { public static void main(String[] args) { Stack<Integer> numbers = new Stack<>(); // 使用Integer,而不是integer numbers.push(12); numbers.push(1); numbers.push(3); numbers.push(15); numbers.push(4); System.out.println("栈的内容: " + numbers); System.out.println("栈中的偶数: "); while(!numbers.empty()) { // 更简洁的写法 int number = numbers.pop(); if(number % 2 == 0){ System.out.println(number); } } } }
The output of the above program is as follows:
<code>栈的内容: [12, 1, 3, 15, 4] 栈中的偶数: 4 12</code>
Improvements: Correct integer
to Integer
, because integer
is not a valid type in Java, the Integer
should be used. At the same time, the code comments and statements have been adjusted to make them clearer and easier to understand.
The above is the detailed content of Returning even numbers from a stack in java. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


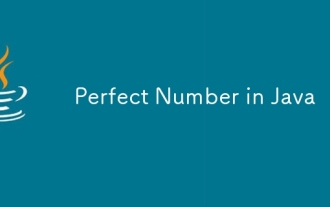
Guide to Perfect Number in Java. Here we discuss the Definition, How to check Perfect number in Java?, examples with code implementation.
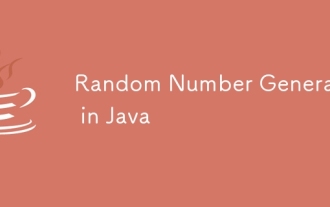
Guide to Random Number Generator in Java. Here we discuss Functions in Java with examples and two different Generators with ther examples.
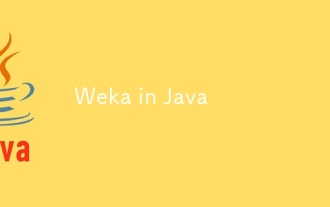
Guide to Weka in Java. Here we discuss the Introduction, how to use weka java, the type of platform, and advantages with examples.
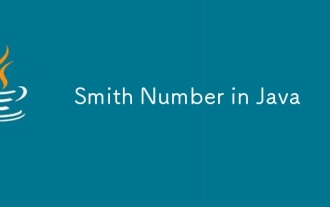
Guide to Smith Number in Java. Here we discuss the Definition, How to check smith number in Java? example with code implementation.

In this article, we have kept the most asked Java Spring Interview Questions with their detailed answers. So that you can crack the interview.
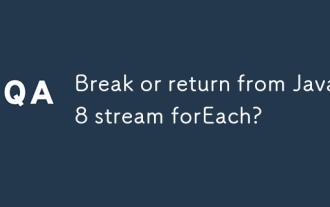
Java 8 introduces the Stream API, providing a powerful and expressive way to process data collections. However, a common question when using Stream is: How to break or return from a forEach operation? Traditional loops allow for early interruption or return, but Stream's forEach method does not directly support this method. This article will explain the reasons and explore alternative methods for implementing premature termination in Stream processing systems. Further reading: Java Stream API improvements Understand Stream forEach The forEach method is a terminal operation that performs one operation on each element in the Stream. Its design intention is
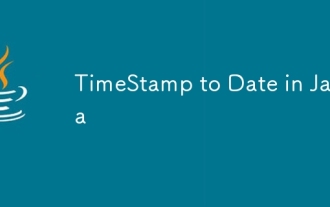
Guide to TimeStamp to Date in Java. Here we also discuss the introduction and how to convert timestamp to date in java along with examples.
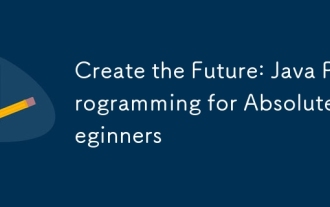
Java is a popular programming language that can be learned by both beginners and experienced developers. This tutorial starts with basic concepts and progresses through advanced topics. After installing the Java Development Kit, you can practice programming by creating a simple "Hello, World!" program. After you understand the code, use the command prompt to compile and run the program, and "Hello, World!" will be output on the console. Learning Java starts your programming journey, and as your mastery deepens, you can create more complex applications.
