Quick Tip: How to Filter Data with PHP
Key Points
- Do not trust external input in the application. It is crucial to filter any data contained in your application to prevent attackers from injecting code.
- The two main types of data filtering in PHP are verification and cleaning. Verification ensures that external inputs meet expectations, while cleaning removes illegal or unsafe characters from external inputs.
- PHP provides a range of filters for verification and cleaning. These filters can be applied using the
filter_var()
andfilter_input()
functions to make your PHP applications safer and more reliable.
This article will explore why anything contained in a filter application is so important. In particular, we will look at how to validate and clean external data in PHP.
Absolutely don't trust external inputs in the app . This is one of the most important lessons that anyone developing a web application must learn.
External input can be anything - input data from $_GET
and $_POST
forms, certain elements in the HTTP request body, and even certain values in the $_SERVER
hyperglobal variable. Cookies, session values, and uploaded and downloaded document files are also considered external inputs.
Every time we process, output, include, or connect external data into our code, it is possible for an attacker to inject the code into our application (so-called injection attack). Therefore, we need to ensure that each piece of external data is properly filtered so that it can be safely integrated into the application.
In terms of filtering, there are two main types: verification and cleaning.
Verification
Verify that external inputs meet our expectations. For example, we might expect an email address, so we expect a format similar to name@domain.com
. To do this, we can use the FILTER_VALIDATE_EMAIL
filter. Or, if we expect a boolean, we can use PHP's FILTER_VALIDATE_BOOL
filter.
The most useful filters include FILTER_VALIDATE_BOOL
, FILTER_VALIDATE_INT
and FILTER_VALIDATE_FLOAT
for filtering basic types, and FILTER_VALIDATE_EMAIL
and FILTER_VALIDATE_DOMAIN
for filtering emails and domain names, respectively.
Another very important filter is FILTER_VALIDATE_REGEXP
, which allows us to filter according to regular expressions. With this filter, we can create a custom filter by changing the regular expressions we filter.
All filters available for verification in PHP can be found here.
Cleaning
Cleaning is the process of removing illegal or unsafe characters from external input.
The best example is that we clean up the database input before inserting it into the original SQL query.
Equally, some of the most useful cleaning filters include filters for cleaning basic types such as FILTER_SANITIZE_STRING
, FILTER_SANITIZE_CHARS
and FILTER_SANITIZE_INT
, as well as FILTER_SANITIZE_URL
and FILTER_SANITIZE_EMAIL
for cleaning URLs and electronics mail.
All PHP cleanup filters can be found here.
filter_var()
and filter_input()
Now that we know that PHP provides a variety of filters, we need to know how to use them.
The filter application is done through the filter_var()
and filter_input()
functions.
filter_var()
function applies the specified filter to the variable. It will get the value to filter, the filter to apply, and an optional array of options. For example, if we try to verify the email address, we can use the following code:
<?php $email = 'your.email@sitepoint.com'; if (filter_var($email, FILTER_VALIDATE_EMAIL)) { echo("This email is valid"); }
If the goal is to clean the string, we can use the following code:
<?php $string = "<h1 id="Hello-World">Hello World</h1>"; $sanitized_string = filter_var($string, FILTER_SANITIZE_STRING); echo $sanitized_string;
filter_input()
Function takes external input from form input and filters it.
It works similarly to the filter_var()
function, but it requires the input type (we can choose from GET, POST, COOKIE, SERVER, or ENV), the variables to filter, and the filter. It also selectively gets an array of options.
Similarly, if we want to check if the external input variable "email" is sent to our application via GET, we can use the following code:
<?php if (filter_input(INPUT_GET, "email", FILTER_VALIDATE_EMAIL)) { echo "The email is being sent and is valid."; }
Conclusion
These are the basics of data filtering in PHP. Other techniques can be used to filter external data, such as applying regular expressions, but the techniques we see in this article are sufficient for most use cases.
Make sure you understand the difference between validation and cleaning and how to use the filter function. With this knowledge, your PHP application will be more reliable and secure!
FAQs about PHP Data Filtering (FAQ)
What is the importance of PHP data filtering?
PHP data filtering is critical to ensuring the security and integrity of your web applications. It helps to verify and clean user input, a common source of security vulnerabilities such as SQL injection and cross-site scripting (XSS). By filtering data, you can prevent malicious or incorrect data from causing damage to your application or database. It also helps maintain data consistency and reliability, which is critical to the smooth operation of the application.
How does PHP data filtering work?
PHP data filtering works by applying specific filter functions to the data. These functions can verify the data (check whether the data meets a specific condition) or clean up the data (removing any illegal or unsafe characters from the data). PHP provides a variety of built-in filter functions that you can use, such as filter_var()
, filter_input()
, and filter_has_var()
.
What are some common PHP filter functions and how to use them?
Some common PHP filter functions include filter_var()
, filter_input()
, filter_has_var()
, and filter_id()
. The filter_var()
function uses the specified filter to filter variables. filter_input()
Functions take specific external variables by name and filter them optionally. The filter_has_var()
function checks whether the variable of the specified input type exists, while the filter_id()
function returns the filter ID belonging to the named filter.
How to use the filter_var()
function in PHP?
The filter_var()
function in PHP is used to filter variables using the specified filter. It accepts two parameters: the variable to be filtered and the type of filter to be applied. For example, to verify that a variable is an email, you can use the FILTER_VALIDATE_EMAIL
filter with the filter_var()
function as follows:
<?php $email = 'your.email@sitepoint.com'; if (filter_var($email, FILTER_VALIDATE_EMAIL)) { echo("This email is valid"); }
What is the difference between verifying and cleaning data in PHP?
Verifying data in PHP involves checking whether the data meets certain conditions. For example, you can verify your email address to make sure it is formatted correctly. On the other hand, cleaning up data involves deleting or replacing any illegal or unsafe characters from the data. This is often intended to prevent security vulnerabilities such as SQL injection and cross-site scripting (XSS).
How to clean up user input in PHP?
You can use the filter_input()
function and cleaning filter to clean user input in PHP. For example, to clean the username GET input, you can use the FILTER_SANITIZE_STRING
filter as follows:
<?php $string = "<h1 id="Hello-World">Hello World</h1>"; $sanitized_string = filter_var($string, FILTER_SANITIZE_STRING); echo $sanitized_string;
What are some common PHP filter flags and how to use them?
PHP filter flag is used to add additional constraints or modifiers to the filtering process. Some common signs include FILTER_FLAG_STRIP_LOW
, FILTER_FLAG_STRIP_HIGH
, FILTER_FLAG_ENCODE_LOW
and FILTER_FLAG_ENCODE_HIGH
. For example, the FILTER_FLAG_STRIP_LOW
flag removes characters with ASCII values below 32 from the input.
How to use filter flags in PHP?
You can use filter flags in PHP by passing them as third arguments to the filter_var()
or filter_input()
functions. For example, to filter IP addresses and allow only IPv4 addresses, you can use the FILTER_FLAG_IPV4
flag as follows:
<?php if (filter_input(INPUT_GET, "email", FILTER_VALIDATE_EMAIL)) { echo "The email is being sent and is valid."; }
What is the filter_has_var()
function in PHP?
The filter_has_var()
function in PHP is used to check whether a variable of the specified input type exists. It accepts two parameters: input type and variable name. Returns TRUE if the variable exists; otherwise, returns FALSE.
How to use the filter_has_var()
function in PHP?
You can use the filter_has_var()
function in PHP to check if a specific input variable exists. For example, to check if a POST variable named "username" exists, you can do this:
<?php $email = 'your.email@sitepoint.com'; if (filter_var($email, FILTER_VALIDATE_EMAIL)) { echo("This email is valid"); }
The above is the detailed content of Quick Tip: How to Filter Data with PHP. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
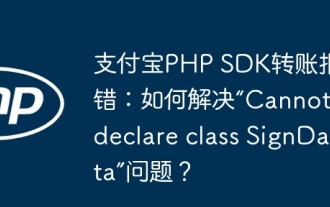
Alipay PHP...
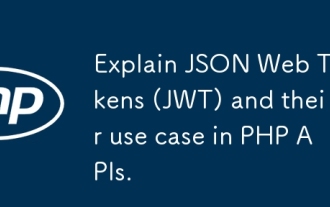
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
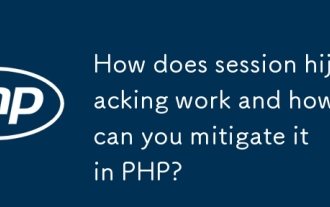
Session hijacking can be achieved through the following steps: 1. Obtain the session ID, 2. Use the session ID, 3. Keep the session active. The methods to prevent session hijacking in PHP include: 1. Use the session_regenerate_id() function to regenerate the session ID, 2. Store session data through the database, 3. Ensure that all session data is transmitted through HTTPS.
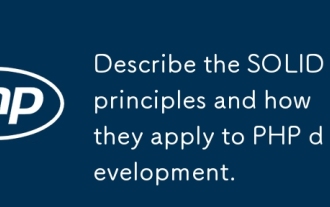
The application of SOLID principle in PHP development includes: 1. Single responsibility principle (SRP): Each class is responsible for only one function. 2. Open and close principle (OCP): Changes are achieved through extension rather than modification. 3. Lisch's Substitution Principle (LSP): Subclasses can replace base classes without affecting program accuracy. 4. Interface isolation principle (ISP): Use fine-grained interfaces to avoid dependencies and unused methods. 5. Dependency inversion principle (DIP): High and low-level modules rely on abstraction and are implemented through dependency injection.
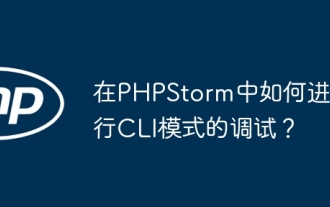
How to debug CLI mode in PHPStorm? When developing with PHPStorm, sometimes we need to debug PHP in command line interface (CLI) mode...
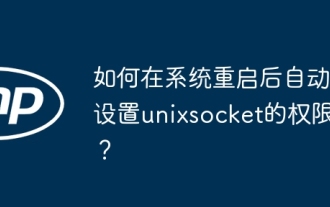
How to automatically set the permissions of unixsocket after the system restarts. Every time the system restarts, we need to execute the following command to modify the permissions of unixsocket: sudo...
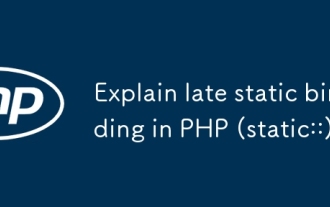
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.
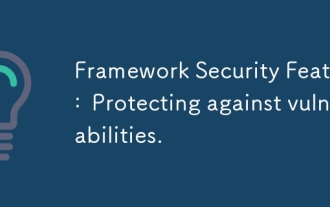
Article discusses essential security features in frameworks to protect against vulnerabilities, including input validation, authentication, and regular updates.
