How to Build an Accordion Component with React.js
This article demonstrates building a dynamic accordion component using React.js. It's a user-friendly, space-saving UI element ideal for web and mobile apps.
Prerequisites:
- Node.js (downloadable from the official website)
- Basic HTML, CSS, and JavaScript knowledge
- Fundamental React.js understanding
- A code editor (Visual Studio Code recommended)
Project Setup:
- Open your terminal and navigate to your desired directory.
- Create a new React app using:
npx create-react-app accordion-component
- After installation, you'll see a confirmation message. The
/accordion-component/
folder contains all the necessary files.
Project Structure and Initial Setup:
- Open the
/accordion-component/
folder in your code editor. - Start the React app in your browser using
npm run start
(from the terminal). - Create a
/src/AccordionComponent/
folder. Inside, createAccordion.js
(for the component) andAccordionData.js
(for data storage). - In
App.js
, import and renderAccordion.js
:
import './App.css'; import Accordion from './AccordionComponent/Accordion'; function App() { return ( <div className="App"> <Accordion /> </div> ); } export default App;
- Clear the contents of
App.css
andindex.css
.
Accordion Component Structure:
- In
Accordion.js
, create theAccordionItem
component:
import React from 'react'; const AccordionItem = () => { return (<h1 id="Accordion">Accordion</h1>); }; const Accordion = () => { return ( <div> <AccordionItem /> </div> ); }; export default Accordion;
- Create the data array in
AccordionData.js
:
const data = [ { question: 'What are accordion components?', answer: '...' }, { question: 'What are they used for?', answer: '...' }, // ... more questions and answers ]; export default data;
(Replace ...
with your actual question and answer text.)
Component Layout and Styling:
- Install
react-icons
:npm install react-icons
- Import
useState
,useRef
, andRiArrowDropDownLine
inAccordion.js
:
import React, { useRef, useState } from 'react'; import { RiArrowDropDownLine } from 'react-icons/ri';
- Implement the
AccordionItem
component:
const AccordionItem = ({ question, answer, isOpen, onClick }) => { const contentHeight = useRef(); return ( <div className="wrapper"> <div className={`question-container ${isOpen ? 'active' : ''}`} onClick={onClick}> <p className="question-content">{question}</p> <RiArrowDropDownLine className={`arrow ${isOpen ? 'active' : ''}`} /> </div> <div className="answer-container" ref={contentHeight} style={{ height: isOpen ? contentHeight.current.scrollHeight : "0px" }}> <p className="answer-content">{answer}</p> </div> </div> ); };
-
Add CSS styling (in a new
Accordion.css
file or withinApp.css
): (The provided CSS is extensive; consider breaking it into smaller, more manageable stylesheets). -
Implement the main
Accordion
component:
import './App.css'; import Accordion from './AccordionComponent/Accordion'; function App() { return ( <div className="App"> <Accordion /> </div> ); } export default App;
Remember to import data
from AccordionData.js
and link the CSS file. This detailed breakdown provides a clearer path to building the accordion component. The original response's code blocks were very long and difficult to follow. This revised answer breaks it down into smaller, more manageable chunks.
The above is the detailed content of How to Build an Accordion Component with React.js. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics




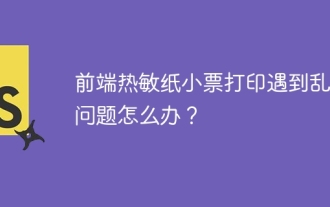
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
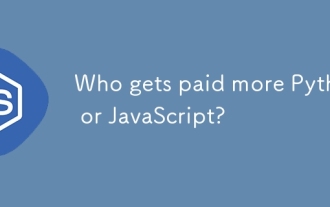
There is no absolute salary for Python and JavaScript developers, depending on skills and industry needs. 1. Python may be paid more in data science and machine learning. 2. JavaScript has great demand in front-end and full-stack development, and its salary is also considerable. 3. Influencing factors include experience, geographical location, company size and specific skills.
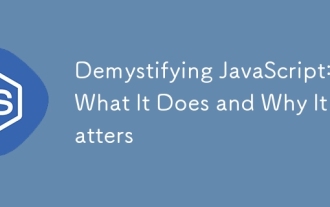
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
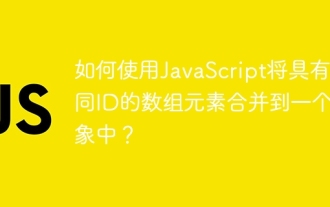
How to merge array elements with the same ID into one object in JavaScript? When processing data, we often encounter the need to have the same ID...
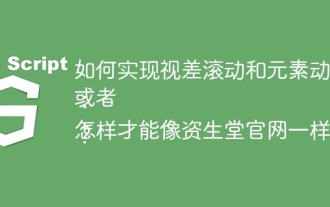
Discussion on the realization of parallax scrolling and element animation effects in this article will explore how to achieve similar to Shiseido official website (https://www.shiseido.co.jp/sb/wonderland/)...
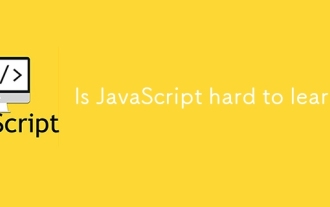
Learning JavaScript is not difficult, but it is challenging. 1) Understand basic concepts such as variables, data types, functions, etc. 2) Master asynchronous programming and implement it through event loops. 3) Use DOM operations and Promise to handle asynchronous requests. 4) Avoid common mistakes and use debugging techniques. 5) Optimize performance and follow best practices.
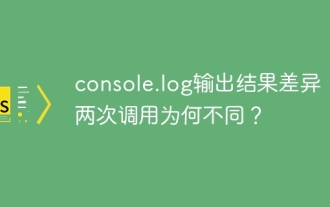
In-depth discussion of the root causes of the difference in console.log output. This article will analyze the differences in the output results of console.log function in a piece of code and explain the reasons behind it. �...
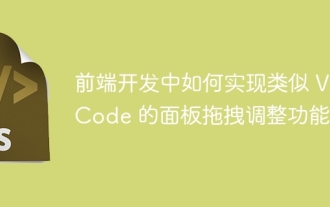
Explore the implementation of panel drag and drop adjustment function similar to VSCode in the front-end. In front-end development, how to implement VSCode similar to VSCode...
