Quick Tip: How To Check if a Variable Is Set in PHP
This quick PHP tip guide will explain how to check if a variable is set and if it is set, what its value is - this is a very common task in programming.
When checking whether a variable is set in PHP, our first reaction may be to use the isset()
function. While this works in most cases, if we look at the definition of the isset()
function, we will see the following:
isset()
: Determines whether a variable has been declared and is not NULL.
However, in PHP we can set the variable to NULL:
<?php $variable = NULL; var_dump(isset($variable));
This assignment will return false - even if we have explicitly set the variable to NULL! This is a situation that may cause some confusion in PHP. In the following we will look at the isset()
method and when to use it, and what other methods we can use when isset()
does not work.
empty()
and is_null()
appear
While the isset()
method can help us determine if a variable has some value set, it will not help us if the variable is set to NULL. To handle this situation, we need to use the empty()
or is_null()
functions. The empty()
function will determine whether the variable is empty. It will be valid for the following situations (i.e. return true):
- "" (empty string)
- 0 (integer 0)
- 0.0 (floating point number 0)
- "0" (String 0)
- NULL
- FALSE
- array() (empty array)
- $var; (variable declared but without value)
This means we can use empty()
to determine whether the variable is empty. In this case, both NULL and false are considered empty:
<?php $variable = NULL; var_dump(empty($variable));
We can also use the is_null()
function. This function determines whether the variable is null.
The following is an example of how to use is_null()
to determine if a variable is null:
<?php $variable = NULL; var_dump(is_null($variable));
The most important thing when dealing with PHP variables is to understand the difference between isset()
, is_null()
and empty()
. All three functions can be used to determine the state of variables, but they behave differently. The following table can be used to highlight the differences between these functions.
"" "foo" NULL FALSE 0 Undefined Trueempty()
True False True True True True True Trueis_null()
False False False True False Falseisset()
False True (Error)
True True True
Is there a single function that we can use?
Using get_defined_vars()
will return an associative array with the key as the variable name and the value as the variable value. We still can't use isset(get_defined_vars()['variable'])
here because the key may exist and the value is still null, so we have to use array_key_exists('variable', get_defined_vars())
.
Example:
<?php $variable = NULL; var_dump(isset($variable));
Using get_defined_vars()
this way, we can 100% determine whether we are checking whether the variable is set.
Conclusion
In this short article, we discuss how to check if a variable is set in PHP. We also looked at the differences between set, empty and null, as well as key considerations to keep in mind when using common functions isset()
, is_null()
and empty()
. So the next time you need to check the status of the PHP variable, you will have all the information you need so that you can choose the right method and disambiguate all the ambiguity in your code.
FAQ for PHP variable checking (FAQ)
What is the purpose of the function in PHP? isset()
The function in PHP is used to determine whether the variable is set and not NULL. If the variable exists and is not NULL, this function returns true, otherwise false. This is a useful function to prevent errors when you try to access variables that may not exist or may have NULL values. isset()
How is the difference between the
function in isset()
in PHP? empty()
Although both and isset()
functions are used to check the values of variables, they work slightly differently. The empty()
function checks whether the variable is set and not NULL. On the other hand, the isset()
function checks whether the variable is empty. If the variable does not exist or its value is equal to FALSE, the variable is considered empty. Therefore, if the variable is not set or its value is 0, 0.0, "", "0", NULL, FALSE, array(), or unset variable, empty()
will return true. empty()
function to check if the array key exists? isset()
Yes, you can use the function to check if there is a specific key in the array. If the key exists and its value is not NULL, isset()
will return true. If the key does not exist or its value is NULL, isset()
will return false. isset()
You can check if multiple variables are set by passing multiple variables to the
function. This function returns true only if all specified variables are set and not NULL. isset()
for unsetted variables? isset()
If you use for unset variables, it will return false. This is because the isset()
function in PHP is used to destroy the specified variable, so after the variable is unset, it is no longer set and its value is considered NULL. unset()
Can I use isset()
to check if the variable is set to 0?
Yes, you can use isset()
to check if the variable is set to 0. The isset()
function only checks whether the variable is set and not NULL. It does not check the value of the variable. Therefore, if the variable is set to 0, isset()
will return true.
How to use isset()
to avoid undefined variable errors?
You can use isset()
to check if the variable is set before trying to use it, thus avoiding undefined variable errors. If isset()
returns false, it means the variable is not set and you can avoid using it, thus preventing undefined variable errors.
Can I use isset()
with object properties in PHP?
Yes, you can use isset()
with object properties in PHP. The isset()
function can be used to check whether the properties exist in the object. If the property exists and its value is not NULL, isset()
will return true.
What is the difference between isset()
and is_null()
in
?
The isset()
is_null()
function checks whether the variable is set and not NULL, while the isset()
function checks whether the variable is NULL. So if the variable is set to NULL, is_null()
will return false and
isset()
Can I use
to check if the form field has been filled in?
isset()
Yes, you can use isset()
to check if the form field has been filled in. When a form is submitted, the form field is sent to the server as a variable. You can use
The above is the detailed content of Quick Tip: How To Check if a Variable Is Set in PHP. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
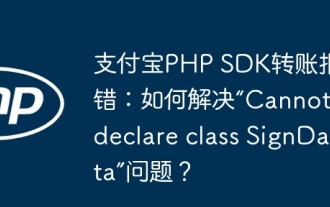
Alipay PHP...
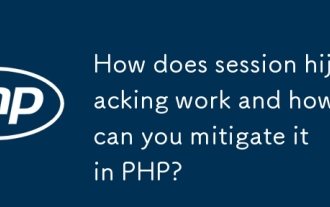
Session hijacking can be achieved through the following steps: 1. Obtain the session ID, 2. Use the session ID, 3. Keep the session active. The methods to prevent session hijacking in PHP include: 1. Use the session_regenerate_id() function to regenerate the session ID, 2. Store session data through the database, 3. Ensure that all session data is transmitted through HTTPS.
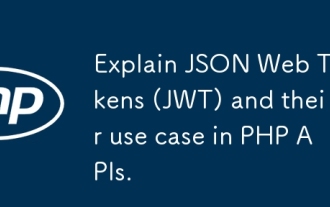
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
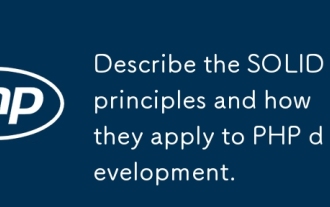
The application of SOLID principle in PHP development includes: 1. Single responsibility principle (SRP): Each class is responsible for only one function. 2. Open and close principle (OCP): Changes are achieved through extension rather than modification. 3. Lisch's Substitution Principle (LSP): Subclasses can replace base classes without affecting program accuracy. 4. Interface isolation principle (ISP): Use fine-grained interfaces to avoid dependencies and unused methods. 5. Dependency inversion principle (DIP): High and low-level modules rely on abstraction and are implemented through dependency injection.
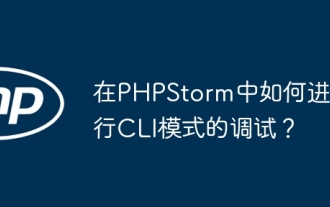
How to debug CLI mode in PHPStorm? When developing with PHPStorm, sometimes we need to debug PHP in command line interface (CLI) mode...
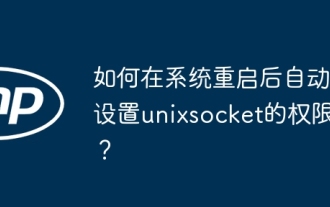
How to automatically set the permissions of unixsocket after the system restarts. Every time the system restarts, we need to execute the following command to modify the permissions of unixsocket: sudo...
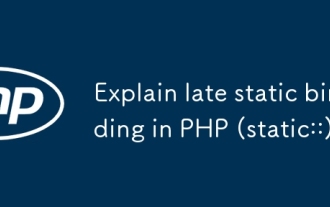
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.
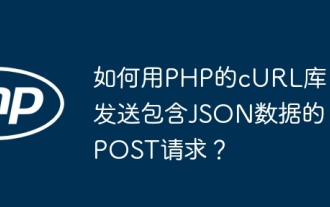
Sending JSON data using PHP's cURL library In PHP development, it is often necessary to interact with external APIs. One of the common ways is to use cURL library to send POST�...
