A Beginner's Guide to Setting Up a Project in Laravel
This guide provides a foundational understanding of Laravel and walks you through setting up a small project. Laravel, a popular PHP framework, is known for its elegant design and powerful features, making it suitable for projects of all sizes.
Prerequisites: Setting Up Your Laravel Environment
Before starting, ensure you have the necessary tools:
-
PHP: Verify PHP is installed by running
php -v
in your terminal. If not, download the latest version from the official PHP website or use Laravel Homestead for a pre-configured environment. Homestead offers a streamlined setup, especially for beginners. - Composer: Composer is the PHP dependency manager. It's essential for managing Laravel's dependencies.
-
Laravel Installer: Globally install the Laravel installer using Composer:
composer global require laravel/installer
. Remember to add Composer's global bin directory to your system's PATH environment variable. Alternatives like Laravel Herd (a Docker-based solution) offer lightweight alternatives to Homestead.
With PHP, Composer, and the Laravel installer (or Homestead/Herd) in place, you're ready to build your Laravel application.
Creating a New Laravel Project
Use the following command to create a new project:
composer create-project --prefer-dist laravel/laravel my-project
Replace my-project
with your desired project name. This command downloads Laravel and sets up the project directory.
Understanding the Laravel Project Directory Structure
Laravel uses a well-organized directory structure:
app
: Contains your application's core logic (controllers, models, etc.).bootstrap
: Bootstrapping and configuration files.config
: Configuration files for database connections, services, and more.database
: Database migrations and seeders.public
: Publicly accessible assets (CSS, JavaScript, images,index.php
).resources
: Uncompiled assets (Blade templates, Sass, JavaScript).routes
: Routing configuration.storage
: Temporary files, cache, and logs.vendor
: Composer-managed dependencies.
Database Configuration and Environment Variables
Configure your database connection in the .env
file (located in the project root). This file contains environment-specific settings like database credentials. For security, use environment variables to store sensitive information. Example .env
entries:
composer create-project --prefer-dist laravel/laravel my-project
Access these variables in your configuration files using the env()
function:
<code>DB_CONNECTION=mysql DB_HOST=127.0.0.1 DB_PORT=3306 DB_DATABASE=my_database DB_USERNAME=my_username DB_PASSWORD=my_password</code>
Routing, Controllers, and Views
Laravel uses a MVC (Model-View-Controller) architecture.
-
Routing (
routes/web.php
): Define routes to map URLs to controller actions. Example:Route::get('/welcome', [WelcomeController::class, 'index']);
-
Controllers (
app/Http/Controllers
): Handle requests and interact with models. -
Views (
resources/views
): Present data to the user using Blade templating.
Database Migrations and Seeding
-
Migrations: Version-controlled database schema changes. Create migrations using
php artisan make:migration create_books_table
. -
Seeders: Populate your database with sample data. Create seeders using
php artisan make:seeder BooksTableSeeder
.
Eloquent ORM (Object-Relational Mapping)
Eloquent simplifies database interactions. Create models using php artisan make:model Book
.
Building a Simple CRUD Application (Book Registration)
This section outlines creating a basic book registration application to demonstrate CRUD (Create, Read, Update, Delete) operations. This example focuses on the initial setup; completing the full CRUD functionality is left as an exercise.
-
Migration: Create a migration for the
books
table:php artisan make:migration create_books_table
. Define the table structure (id, title, author, timestamps) within the migration file. Run the migration:php artisan migrate
. -
Seeder: Create a seeder:
php artisan make:seeder BooksTableSeeder
. Populate thebooks
table with sample data. Run the seeder:php artisan db:seed --class=BooksTableSeeder
. -
Controller: Create a
BookController
. Implement methods for index (listing books), create (displaying the creation form), store (saving new books), etc. -
Views: Create Blade views (e.g.,
resources/views/books/index.blade.php
,resources/views/books/create.blade.php
) to display and manage book data.
Conclusion
This guide covered the fundamentals of Laravel project setup and a basic CRUD application. Refer to the official Laravel documentation for more advanced topics and best practices. Consider exploring Laravel boilerplates for pre-configured project structures.
The above is the detailed content of A Beginner's Guide to Setting Up a Project in Laravel. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
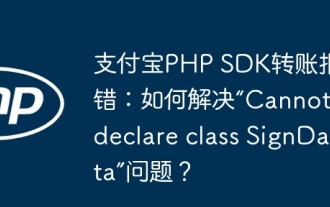
Alipay PHP...
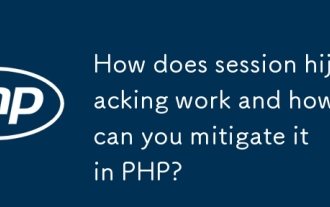
Session hijacking can be achieved through the following steps: 1. Obtain the session ID, 2. Use the session ID, 3. Keep the session active. The methods to prevent session hijacking in PHP include: 1. Use the session_regenerate_id() function to regenerate the session ID, 2. Store session data through the database, 3. Ensure that all session data is transmitted through HTTPS.
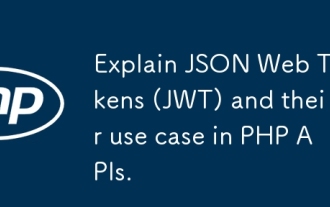
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
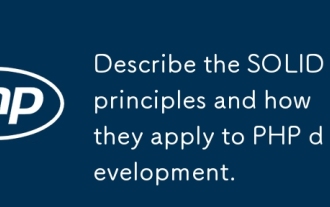
The application of SOLID principle in PHP development includes: 1. Single responsibility principle (SRP): Each class is responsible for only one function. 2. Open and close principle (OCP): Changes are achieved through extension rather than modification. 3. Lisch's Substitution Principle (LSP): Subclasses can replace base classes without affecting program accuracy. 4. Interface isolation principle (ISP): Use fine-grained interfaces to avoid dependencies and unused methods. 5. Dependency inversion principle (DIP): High and low-level modules rely on abstraction and are implemented through dependency injection.
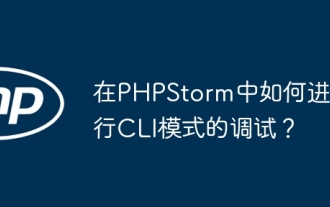
How to debug CLI mode in PHPStorm? When developing with PHPStorm, sometimes we need to debug PHP in command line interface (CLI) mode...
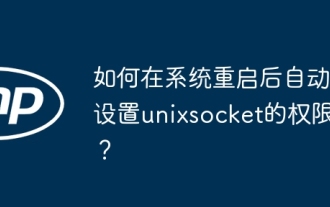
How to automatically set the permissions of unixsocket after the system restarts. Every time the system restarts, we need to execute the following command to modify the permissions of unixsocket: sudo...
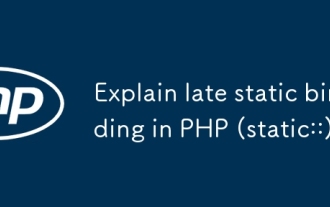
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.
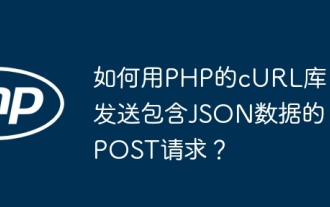
Sending JSON data using PHP's cURL library In PHP development, it is often necessary to interact with external APIs. One of the common ways is to use cURL library to send POST�...
