How to Create Interactive Animations Using React Spring
React Spring: Elevate Your React Animations with Physics-Based Motion
This article explores React Spring, a JavaScript animation library for creating natural-looking, physics-based animations within React applications. We'll cover its key features, hooks, and practical implementation.
Frontend animation in React has evolved significantly. While CSS transitions and animations sufficed initially, the complexity of modern React applications demanded more robust solutions. JavaScript animation libraries like Framer Motion, Remotion, and React Spring emerged to meet this need, each offering unique strengths. React Spring distinguishes itself with its physics-based approach, resulting in smoother, more realistic animations.
Key Concepts:
- Physics-Based Animations: React Spring uses spring physics to simulate real-world motion, creating more engaging user experiences.
-
Versatile Hooks: It provides several hooks for diverse animation needs:
useSpring
(single spring animations),useTransition
(list item animations),useTrail
(sequential animations),useChain
(animation sequences), anduseSprings
(multiple simultaneous animations). - Easy Setup: Installation via npm or yarn is straightforward, followed by importing necessary components and hooks.
-
useSpring
: This core hook defines animation start and end points, managing the transition smoothly using spring physics. -
useTransition
: Ideal for animating list items as they enter, exit, or change within the DOM, creating fluid list updates and modal transitions.
Prerequisites:
This tutorial assumes familiarity with:
- React and React Hooks
- JavaScript and JSX
- Node.js and npm (or yarn)
- A code editor (e.g., Visual Studio Code)
Introducing React Spring
React Spring empowers developers to craft interactive animations that enhance user engagement. Unlike purely CSS-based methods or other animation libraries, its physics engine provides a more natural feel to the animations. Animations can be applied to any component property (position, scale, opacity, etc.).
Setting up React Spring
-
Installation: Use npm or yarn:
npm install react-spring # or yarn add react-spring
Copy after loginCopy after login -
Import: Import required components and hooks:
import { animated, useSpring } from 'react-spring'; // Import other hooks as needed
Copy after loginCopy after loginanimated
wraps standard HTML elements, enabling animation via React Spring's hooks.
React Spring Hooks: A Deep Dive
React Spring's power lies in its specialized hooks:
useSpring
: Creates single spring animations, transitioning data from an initial state to a target state.useTransition
: Animates array elements as they are added, removed, or reordered in the DOM. Excellent for dynamic lists and modals.useTrail
: Generates sequential animations, creating a "trail" effect where animations follow one another.useChain
: Orchestrates animation sequences, defining the order of execution for multiple animations.useSprings
: Manages multiple spring animations concurrently.
Animating with useSpring
useSpring
uses spring physics to create animations. You define the animation's start and end points, and React Spring handles the smooth transition.
Method 1: Object Literal
npm install react-spring # or yarn add react-spring
This directly sets the animation properties.
Method 2: Function Parameter
import { animated, useSpring } from 'react-spring'; // Import other hooks as needed
This offers more dynamic control based on state or props.
Animating Lists with useTransition
useTransition
animates items entering, leaving, or updating within an array. It manages the animation lifecycle, ensuring smooth transitions.
Sequential Animations with useTrail
useTrail
creates a "trail" effect, animating elements sequentially with configurable delays.
Chaining Animations with useChain
useChain
links multiple animations together, controlling their execution order and timing.
Multiple Animations with useSprings
useSprings
handles multiple independent spring animations simultaneously.
Conclusion
React Spring provides a powerful and intuitive way to create sophisticated, physics-based animations in React. Its diverse hooks cater to various animation needs, resulting in more engaging and natural-feeling user interfaces. Mastering these techniques will significantly enhance your React development capabilities.
The above is the detailed content of How to Create Interactive Animations Using React Spring. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
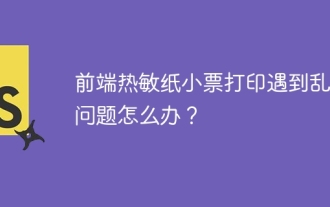
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
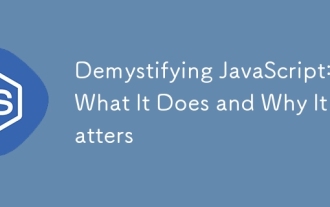
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
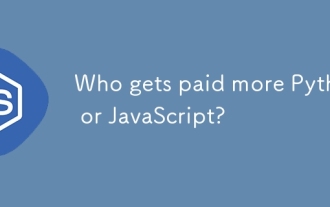
There is no absolute salary for Python and JavaScript developers, depending on skills and industry needs. 1. Python may be paid more in data science and machine learning. 2. JavaScript has great demand in front-end and full-stack development, and its salary is also considerable. 3. Influencing factors include experience, geographical location, company size and specific skills.
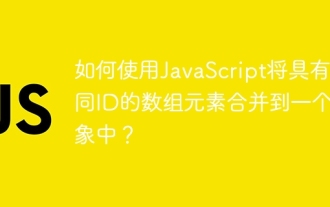
How to merge array elements with the same ID into one object in JavaScript? When processing data, we often encounter the need to have the same ID...
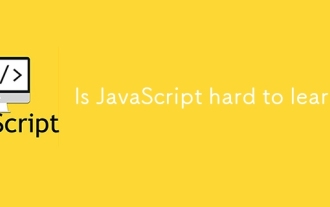
Learning JavaScript is not difficult, but it is challenging. 1) Understand basic concepts such as variables, data types, functions, etc. 2) Master asynchronous programming and implement it through event loops. 3) Use DOM operations and Promise to handle asynchronous requests. 4) Avoid common mistakes and use debugging techniques. 5) Optimize performance and follow best practices.
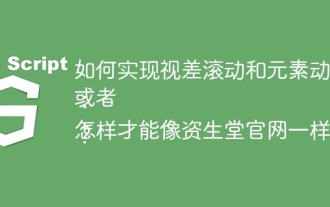
Discussion on the realization of parallax scrolling and element animation effects in this article will explore how to achieve similar to Shiseido official website (https://www.shiseido.co.jp/sb/wonderland/)...
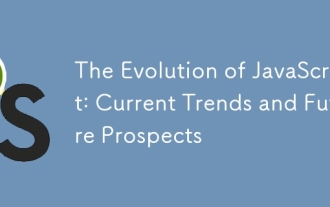
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
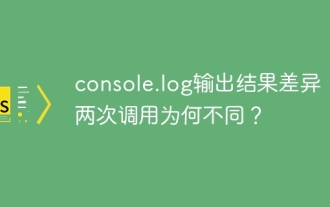
In-depth discussion of the root causes of the difference in console.log output. This article will analyze the differences in the output results of console.log function in a piece of code and explain the reasons behind it. �...
