Building a WordPress Plugin with Vue
This tutorial shows how to build a modern WordPress UI using Vue.js, a progressive JavaScript framework. We'll create a simple plugin with a Vue interface interacting with the WordPress REST API via the Fetch API.
Key Concepts:
- This guide covers creating a WordPress plugin that registers a shortcode, integrating Vue.js, and building a Vue app that interacts with the
/wp-json/wp/v2/posts?filter[orderby]=date
endpoint to display recent posts. - We'll demonstrate creating a Vue instance, using lifecycle hooks like
mounted()
, and fetching data. Real-time updates usingsetInterval()
will also be shown. - The tutorial assumes basic familiarity with Vue.js.
Building the WordPress Plugin:
-
Create Plugin Directory: Create a folder (e.g.,
vueplugin
) within your WordPresswp-content/plugins
directory. -
vueplugin.php
: Inside the folder, createvueplugin.php
with the following initial content:<?php /* Plugin Name: Latest Posts Description: Latest posts shortcode with Vue.js Version: 1.0 */
Copy after login -
Register Shortcode: Add this code to
vueplugin.php
to register a shortcode namedlatestPosts
:function handle_shortcode() { return '<div id="mount"></div>'; } add_shortcode('latestPosts', 'handle_shortcode'); function enqueue_scripts() { global $post; if (has_shortcode($post->post_content, "latestPosts")) { wp_enqueue_script('vue', 'https://cdn.jsdelivr.net/npm/vue@2.5.17/dist/vue.js', [], '2.5.17'); wp_enqueue_script('latest-posts', plugin_dir_url(__FILE__) . 'latest-posts.js', [], '1.0', true); } } add_action('wp_enqueue_scripts', 'enqueue_scripts');
Copy after loginThis enqueues the Vue.js library and a custom JavaScript file (
latest-posts.js
). -
Activate Plugin: Activate the plugin via your WordPress admin dashboard.
-
Test Shortcode: Add
[latestPosts]
to a post or page to test the shortcode.
Integrating Vue.js:
-
latest-posts.js
: Createlatest-posts.js
in your plugin directory with this code:(function() { var vm = new Vue({ el: '#mount', data: { posts: [] }, mounted: function() { this.fetchPosts(); setInterval(this.fetchPosts.bind(this), 5000); }, methods: { fetchPosts: function() { fetch('/wp-json/wp/v2/posts?filter[orderby]=date') .then(response => response.json()) .then(data => this.posts = data); } }, template: ` <div> <h1 id="My-Latest-Posts">My Latest Posts</h1> <div v-if="posts.length > 0"> <ul> <li v-for="post in posts"> <a :href="https://www.php.cn/link/f417d05af72b37f956c906aea42d1511">{{ post.title.rendered }}</a> </li> </ul> </div> <div v-else> <p>Loading posts...</p> </div> </div> ` }); })();
Copy after login
This Vue instance fetches posts, displays them in a list, and updates every 5 seconds.
-
Verification: Check your browser's developer console for "Component is mounted" and the fetched posts. The
latest-posts.js
script should also be included in your page source.
Conclusion:
This enhanced tutorial provides a complete, working example of integrating Vue.js into a WordPress plugin for a dynamic, real-time user experience. Remember to adjust paths and styling as needed for your specific theme. The FAQs from the original input have been omitted as they are adequately covered within the revised and expanded tutorial.
The above is the detailed content of Building a WordPress Plugin with Vue. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
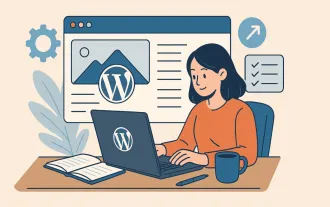
Blogs are the ideal platform for people to express their opinions, opinions and opinions online. Many newbies are eager to build their own website but are hesitant to worry about technical barriers or cost issues. However, as the platform continues to evolve to meet the capabilities and needs of beginners, it is now starting to become easier than ever. This article will guide you step by step how to build a WordPress blog, from theme selection to using plugins to improve security and performance, helping you create your own website easily. Choose a blog topic and direction Before purchasing a domain name or registering a host, it is best to identify the topics you plan to cover. Personal websites can revolve around travel, cooking, product reviews, music or any hobby that sparks your interests. Focusing on areas you are truly interested in can encourage continuous writing

WordPress is easy for beginners to get started. 1. After logging into the background, the user interface is intuitive and the simple dashboard provides all the necessary function links. 2. Basic operations include creating and editing content. The WYSIWYG editor simplifies content creation. 3. Beginners can expand website functions through plug-ins and themes, and the learning curve exists but can be mastered through practice.
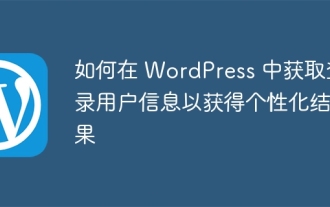
Recently, we showed you how to create a personalized experience for users by allowing users to save their favorite posts in a personalized library. You can take personalized results to another level by using their names in some places (i.e., welcome screens). Fortunately, WordPress makes it very easy to get information about logged in users. In this article, we will show you how to retrieve information related to the currently logged in user. We will use the get_currentuserinfo(); function. This can be used anywhere in the theme (header, footer, sidebar, page template, etc.). In order for it to work, the user must be logged in. So we need to use

Can learn WordPress within three days. 1. Master basic knowledge, such as themes, plug-ins, etc. 2. Understand the core functions, including installation and working principles. 3. Learn basic and advanced usage through examples. 4. Understand debugging techniques and performance optimization suggestions.

WordPressisgoodforvirtuallyanywebprojectduetoitsversatilityasaCMS.Itexcelsin:1)user-friendliness,allowingeasywebsitesetup;2)flexibilityandcustomizationwithnumerousthemesandplugins;3)SEOoptimization;and4)strongcommunitysupport,thoughusersmustmanageper
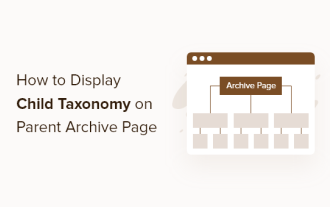
Do you want to know how to display child categories on the parent category archive page? When you customize a classification archive page, you may need to do this to make it more useful to your visitors. In this article, we will show you how to easily display child categories on the parent category archive page. Why do subcategories appear on parent category archive page? By displaying all child categories on the parent category archive page, you can make them less generic and more useful to visitors. For example, if you run a WordPress blog about books and have a taxonomy called "Theme", you can add sub-taxonomy such as "novel", "non-fiction" so that your readers can

Wix is suitable for users who have no programming experience, and WordPress is suitable for users who want more control and expansion capabilities. 1) Wix provides drag-and-drop editors and rich templates, making it easy to quickly build a website. 2) As an open source CMS, WordPress has a huge community and plug-in ecosystem, supporting in-depth customization and expansion.
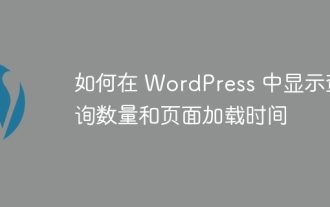
One of our users asked other websites how to display the number of queries and page loading time in the footer. You often see this in the footer of your website, and it may display something like: "64 queries in 1.248 seconds". In this article, we will show you how to display the number of queries and page loading time in WordPress. Just paste the following code anywhere you like in the theme file (e.g. footer.php). queriesin
