Your First PHP Code
Quick review of PHP core concepts
- Server-side language: PHP code is executed on the server side, not on the browser side, which means that the browser only receives standard HTML, avoids browser compatibility issues and alleviates customers end load.
- Dynamic web page generation: PHP embeds HTML, giving web pages stronger dynamic interaction capabilities, surpassing the static rendering of pure HTML.
- Sentences and functions: PHP scripts are composed of a series of statements ending with semicolons. Functions can be called and parameters can be passed in to achieve diversified functions.
-
Delimiters:
<?php
and?>
mark the start and end of the PHP code block, and the server parses the code in this area and converts it to HTML. - Multi-language collaboration: PHP can seamlessly integrate with HTML, CSS, JavaScript, and SQL database technologies, and is an all-round web development tool.
A preliminary exploration of PHP: Write your first PHP script
After building a virtual server, let's start your first PHP scripting journey. As a server-side language, PHP may be different from client languages you are familiar with (such as HTML, CSS, JavaScript).
The server-side language is similar to JavaScript, allowing you to embed applets (scripts) into the HTML code of a web page. After executing these programs, you can gain more control over what is displayed in the browser window than using only HTML. The key difference between JavaScript and PHP is the web page loading phase that executes these embedded programs.
Client languages (such as JavaScript) are read and executed by the web browser after downloading web pages (including embedded programs) from the web server. Instead, a server-side language (such as PHP) is run by a Web server server before sending a web page to the browser. The client language allows you to control how the page behaves after the browser displays, while the server language allows you to dynamically generate custom pages before the page is sent to the browser.
After the web server executes the PHP code embedded in the web page, the result will replace the PHP code in the page. When receiving pages, all browsers see standard HTML code, so it is called a "server-side language". Let's look at a simple PHP example that generates a random number between 1 and 10 and displays it on the screen:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="utf-8"> <title>随机数</title> </head> <body> <p>生成1到10之间的随机数:</p> <?php echo rand(1, 10); ?> </body> </html>
Most of the code is pure HTML. Only the lines between <?php
and ?>
are PHP code. <?php
marks the beginning of an embedded PHP script, ?>
marks its end. The web server is asked to interpret everything between these two delimiters and convert it into regular HTML code before sending it to the web page that requests the browser. If you right-click in your browser and select "View Source Code" (the text may vary depending on the browser you are using), you can see that the browser displays the following:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="utf-8"> <title>随机数</title> </head> <body> <p>生成1到10之间的随机数:</p> <?php echo rand(1, 10); ?> </body> </html>
Please note that all traces of PHP code have disappeared. Instead, the output of the script is what it looks like standard HTML. This example demonstrates several advantages of server-side scripting...
- No browser compatibility issues. PHP scripts are interpreted only by the web server, so there is no need to worry about whether the language features you are using are supported by the visitor's browser.
- Access server-side resources. In the example above, we put the random numbers generated by the web server into the web page. If we insert numbers using JavaScript, the numbers will be generated in the browser and someone might modify the code to insert specific numbers. Of course, more impressive examples of server-side resource utilization include inserting content extracted from a MySQL database.
- Reduce client load. JavaScript can significantly delay the display of web pages (especially on mobile devices!) because the browser must run the script before the web page is displayed. With server-side code, this burden will be transferred to the web server, which you can make powerful based on the requirements of the application (and your wallet can afford).
- Select. When writing code that runs in a browser, the browser must understand how to run the given code. All modern browsers understand HTML, CSS, and JavaScript. To write certain code that runs in a browser, you must use one of these languages. By running code that generates HTML on the server, you can choose from multiple languages—one of which is PHP.
Basic grammar and statements
If you know JavaScript, C, C, C, C#, Objective-C, Java, Perl, or any other C-derived language, then PHP syntax will be very familiar. But if you are not familiar with these languages, or if you are new to programming, you don't need to worry.
PHP script consists of a series of commands or statements. Each statement is a directive that the web server must follow before continuing to execute the next directive. Like the statements in the above languages, PHP statements always end with a semicolon (;).
This is a typical PHP statement:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="utf-8"> <title>随机数</title> </head> <body> <p>生成1到10之间的随机数:</p> 7 </body> </html>
This is an echo statement used to generate content (usually HTML code) to send to the browser. The echo statement simply takes the given text and inserts it into the page HTML code containing its PHP script location.
In this case, we provide a text string to output: this is a test! . Note that the text string contains HTML tags (<code><strong>
and <code></strong>
), which is perfectly acceptable.
So if we put this statement into the full webpage, the generated code is as follows:
echo '这是一个<strong>测试</strong>!';
If you place this file on a web server and then request it using a web browser, your browser will receive this HTML code:
测试页面 <?php echo '这是一个<strong>测试</strong>!'; ?>
The random.php example we saw before contains a slightly more complex echo statement:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="utf-8"> <title>随机数</title> </head> <body> <p>生成1到10之间的随机数:</p> <?php echo rand(1, 10); ?> </body> </html>
You will notice that in the first example, PHP is given some direct print text, and in the second example, PHP is given a directive to follow. PHP tries to read anything that exists outside of quotes as directives it must follow. Anything inside quotes is treated as a string, which means PHP doesn't handle it at all, but just pass it to the command you call. Therefore, the following code passes the string "This is a test!" directly to the echo command:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="utf-8"> <title>随机数</title> </head> <body> <p>生成1到10之间的随机数:</p> 7 </body> </html>
Strings are represented by starting and ending quotes. PHP will see the first '
as the beginning of the string and find the next '
and use it as the end of the string.
Instead, the following code will first run the built-in function rand to generate a random number, and then pass the result to the echo command:
echo '这是一个<strong>测试</strong>!';
You can think of built-in functions as tasks that PHP can perform without you specifying. PHP has many built-in functions that allow you to perform everything from sending emails to using information stored in various types of databases.
PHP does not try to understand strings. They can contain any characters, arranged in any order. But the code—essentially a series of instructions—must follow a strict structure in order for the computer to understand it.
When you call a function in PHP—i.e., when you ask it to do its work—you are said to be calling the function. Most functions return values when called; then PHP behaves like you're actually just typing that return value into your code. In the echo 'rand(1, 10)';
example, our echo statement contains a call to the rand function that returns a random number as a text string. Then, the echo statement outputs the value returned by the function call.
Each function in PHP can have one or more parameters that allow you to make the function run in a slightly different way. The rand function takes two parameters: the minimum random number and the maximum random number. By changing the value passed to the function, you can change how it works. For example, if you want a random number between 1 and 50, you can use the following code:
测试页面 <?php echo '这是一个<strong>测试</strong>!'; ?>
You may be wondering why we need to enclose the parameters in brackets ((1, 50)). Brackets have two functions. First, they mean that rand is a function you want to call. Second, they mark the beginning and end of the parameter list—the PHP statement you wish to provide—to tell the function what you want it to do. For rand function you need to provide the minimum and maximum values. These values are separated by commas.
Later, we will look at functions that take different types of parameters. We will also consider functions that do not take any parameters at all. Even if there is nothing between them, these functions still require parentheses.
(The following is the FAQ part. Due to space limitations, I will only keep the question and a brief summary of the answers. Please refer to the original text for the complete FAQ answer.)
PHP Code FAQ (FAQ)
-
What is the meaning of PHP delimiter?
<?php
and?>
are used to mark the beginning and end of the PHP code block, and the server parses the PHP code accordingly. -
How to write my first PHP program? Create a
.php
file and write PHP code inside<?php ?>
, such asecho "Hello, World!";
. -
What is the role of PHP in web development? PHP is used to create dynamic interactive web pages, process databases, user sessions, forms, etc.
-
How does PHP interact with HTML? PHP code is embedded in HTML
<?php ?>
, and the server outputs the result as HTML after processing. -
What are the common mistakes that beginners in PHP code make? Forgot to close strings or brackets, improper use of semicolons, wrong function syntax, etc.
-
How to debug PHP code? Use
echo
orprint
to output variable values, or use debugging tools such as Xdebug. -
How to protect PHP code security? Verify and clean user input, use secure hashing algorithm to store passwords, update PHP version, use HTTPS connections, etc.
-
Can PHP be used with other programming languages? Can be, for example, with HTML, CSS, JavaScript and SQL databases.
-
How to improve the performance of PHP code? Use efficient algorithms and data structures, minimize database queries, use caching technology, etc.
-
What are the resources to learn PHP? PHP official website (php.net), online courses, books and tutorials, etc.
The above is the detailed content of Your First PHP Code. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics




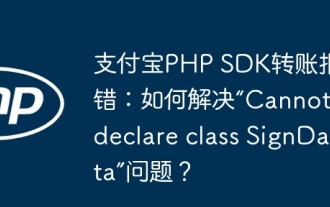
Alipay PHP...
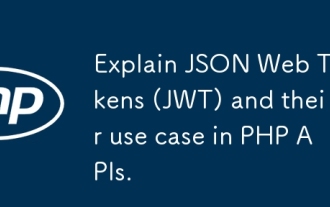
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
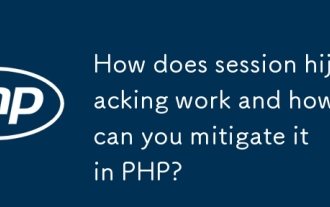
Session hijacking can be achieved through the following steps: 1. Obtain the session ID, 2. Use the session ID, 3. Keep the session active. The methods to prevent session hijacking in PHP include: 1. Use the session_regenerate_id() function to regenerate the session ID, 2. Store session data through the database, 3. Ensure that all session data is transmitted through HTTPS.
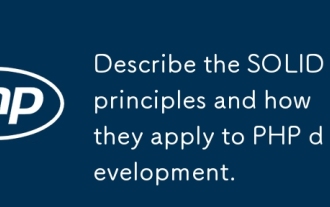
The application of SOLID principle in PHP development includes: 1. Single responsibility principle (SRP): Each class is responsible for only one function. 2. Open and close principle (OCP): Changes are achieved through extension rather than modification. 3. Lisch's Substitution Principle (LSP): Subclasses can replace base classes without affecting program accuracy. 4. Interface isolation principle (ISP): Use fine-grained interfaces to avoid dependencies and unused methods. 5. Dependency inversion principle (DIP): High and low-level modules rely on abstraction and are implemented through dependency injection.
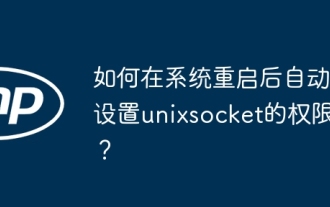
How to automatically set the permissions of unixsocket after the system restarts. Every time the system restarts, we need to execute the following command to modify the permissions of unixsocket: sudo...
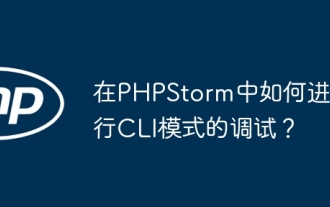
How to debug CLI mode in PHPStorm? When developing with PHPStorm, sometimes we need to debug PHP in command line interface (CLI) mode...
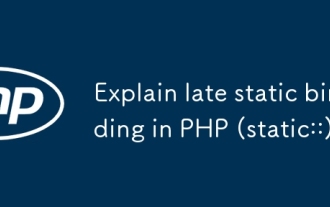
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.
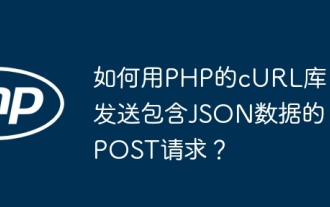
Sending JSON data using PHP's cURL library In PHP development, it is often necessary to interact with external APIs. One of the common ways is to use cURL library to send POST�...
