Upgrading Sylius the TDD Way: Exploring PhpSpec
This article demonstrates extending Sylius's core functionality using Test-Driven Development (TDD) to improve inventory management. We'll add color-coded low-stock indicators to the product listing. This will be a backend implementation; visual testing with Behat will be covered in a future article. Assume you have a working Sylius instance.
Sylius offers robust inventory management, but we can enhance it. Currently, the admin product list lacks stock information. While variant details show stock levels and tracking, adding this to the product list improves usability. We'll also introduce a tiered warning system (e.g., green for ample stock, yellow for low stock, red for out of stock).
Extending ProductVariant
and Product
Models
To add stock availability information, we'll extend Sylius's ProductVariant
and Product
models.
1. Create a Bundle
Create src/AppBundle/AppBundle.php
:
1 2 3 4 5 6 7 8 |
|
Register it in app/AppKernel.php
:
1 2 3 4 5 6 7 8 9 |
|
Update composer.json
's autoload
section:
1 2 3 4 5 6 7 8 9 10 |
|
Run composer dump-autoload
.
2. SpecBDD Tests
We'll use PhpSpec for behavior-driven development. In phpspec.yml.dist
, add:
1 |
|
Clear the cache: php bin/console cache:clear
.
Generate specifications using:
1 2 |
|
Create interfaces: ProductInterface
and ProductVariantInterface
extending their Sylius counterparts. Then create Product.php
and ProductVariant.php
extending the Sylius classes and implementing the new interfaces.
Add a $reorderLevel
property to ProductVariant.php
:
1 2 3 4 5 6 7 8 9 |
|
3. Overriding Sylius Classes
Configure Sylius to use our extended classes in app/config/config.yml
:
1 2 3 4 5 6 7 8 |
|
4. Database Update
Generate and run migrations:
1 2 |
|
(Or use php bin/console doctrine:schema:update --force
if necessary). Create ProductVariant.orm.yml
to define the reorderLevel
column in the database. Create an empty Product.orm.yml
as we're not modifying the sylius_product
table.
5. More SpecBDD Tests
Write PhpSpec tests for ProductVariant
and Product
, implementing methods like getReorderLevel()
, setReorderLevel()
, isReorderable()
, getOnHand()
, and isTracked()
as needed, ensuring all tests pass. The tests should cover various scenarios, including different stock levels and reorder levels. Remember to update the interfaces with the necessary methods.
6. Conclusion
This TDD approach ensures robust code. The next article will cover Behat testing for visual verification.
(FAQs section omitted for brevity, as it's largely unrelated to the core code example and would significantly increase the response length. The provided FAQs are well-written and can be easily included separately.)
The above is the detailed content of Upgrading Sylius the TDD Way: Exploring PhpSpec. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
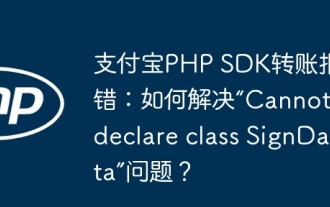
Alipay PHP...
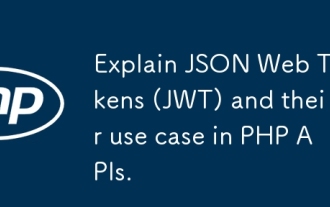
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
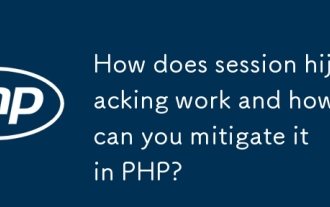
Session hijacking can be achieved through the following steps: 1. Obtain the session ID, 2. Use the session ID, 3. Keep the session active. The methods to prevent session hijacking in PHP include: 1. Use the session_regenerate_id() function to regenerate the session ID, 2. Store session data through the database, 3. Ensure that all session data is transmitted through HTTPS.

The enumeration function in PHP8.1 enhances the clarity and type safety of the code by defining named constants. 1) Enumerations can be integers, strings or objects, improving code readability and type safety. 2) Enumeration is based on class and supports object-oriented features such as traversal and reflection. 3) Enumeration can be used for comparison and assignment to ensure type safety. 4) Enumeration supports adding methods to implement complex logic. 5) Strict type checking and error handling can avoid common errors. 6) Enumeration reduces magic value and improves maintainability, but pay attention to performance optimization.
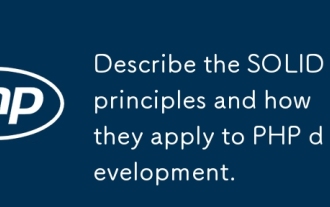
The application of SOLID principle in PHP development includes: 1. Single responsibility principle (SRP): Each class is responsible for only one function. 2. Open and close principle (OCP): Changes are achieved through extension rather than modification. 3. Lisch's Substitution Principle (LSP): Subclasses can replace base classes without affecting program accuracy. 4. Interface isolation principle (ISP): Use fine-grained interfaces to avoid dependencies and unused methods. 5. Dependency inversion principle (DIP): High and low-level modules rely on abstraction and are implemented through dependency injection.
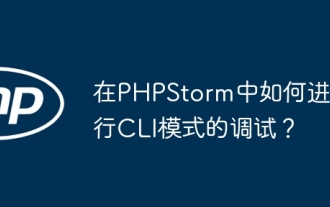
How to debug CLI mode in PHPStorm? When developing with PHPStorm, sometimes we need to debug PHP in command line interface (CLI) mode...
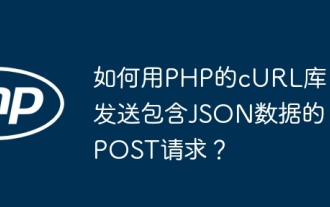
Sending JSON data using PHP's cURL library In PHP development, it is often necessary to interact with external APIs. One of the common ways is to use cURL library to send POST�...
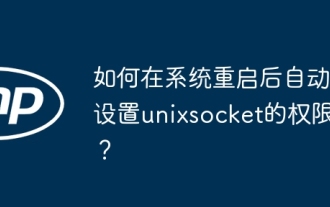
How to automatically set the permissions of unixsocket after the system restarts. Every time the system restarts, we need to execute the following command to modify the permissions of unixsocket: sudo...
