Modding Minecraft with PHP - Buildings from Code!
Using PHP to Mod Minecraft: A Novel Approach
This article explores a unique method for creating Minecraft mods using PHP, bypassing the traditional reliance on Java. Leveraging asynchronous PHP libraries and an event loop, this technique avoids the need for resource-intensive busy loops, enabling efficient mod development.
The challenge of learning Java for Minecraft modding is addressed by this innovative approach. This tutorial demonstrates how to build mods in PHP, overcoming the typical language barrier.
(A future post will detail a 3D JavaScript Minecraft editor for streamlined blueprint creation. Stay tuned!)
(The majority of the code for this tutorial is available on Github. Testing was conducted using the latest Chrome version and PHP 7.0. While cross-browser and PHP version compatibility isn't guaranteed, the core principles remain consistent.)
Setting the Stage
Communication between PHP and the Minecraft server is crucial. Instead of a traditional blocking while
loop:
while (true) { // listen for player requests // make changes to the game sleep(1); }
We utilize AMPHP, an asynchronous PHP library, offering features like event loops and HTTP clients.
First, install necessary libraries:
composer require amphp/amp composer require amphp/file
Then, create a non-blocking event loop:
require __DIR__ . "/vendor/autoload.php"; Amp\run(function() { Amp\repeat(function() { // listen for player requests // make changes to the game }, 1000); });
This non-blocking approach allows for concurrent operations while awaiting potentially blocking tasks.
Promises and Generators: A Powerful Combination
AMPHP's promise-based interface handles asynchronous operations. Promises represent data not yet available, such as results from file system operations or HTTP requests. Generators further enhance this by streamlining iteration over undefined array values.
Here's an example using generators:
use Amp\File\Driver; function getContents(Driver $files, $path, $previous) { $next = yield $files->mtime($path); if ($previous !== $next) { return yield $files->get($path); } return null; }
This function efficiently handles asynchronous file operations without blocking the main thread.
Monitoring Server Logs
The mod listens to server logs to detect player commands. This prevents duplicate command execution.
define("LOG_PATH", "/path/to/logs/latest.log"); $files = Amp\File\filesystem(); // ... (rest of the code remains largely the same)
(Remember to replace /path/to/logs/latest.log
with your server's log file path.)
The code monitors the log file for changes, processes new lines, and executes commands accordingly.
Blueprint Generation and Block Placement
A 3D JavaScript builder (detailed in a separate post) generates an array of block coordinates. This array is used by the PHP script to construct structures within Minecraft.
The executeCommand
function processes the build command and places blocks based on the generated coordinates.
Server Communication via RCON
The Minecraft server's RCON (Remote Console) allows communication with the PHP script. An RCON client library is used to send commands to the server.
while (true) { // listen for player requests // make changes to the game sleep(1); }
(Note: The theory/builder
library includes a Minecraft server for testing purposes.)
The server's server.properties
file needs the following configurations:
composer require amphp/amp composer require amphp/file
The executeCommand
function uses the RCON client to send /setblock
commands to place blocks.
Future Enhancements and Conclusion
This approach opens doors to advanced mod functionalities. Future improvements could include a JSON API for design submission and more complex block arrangements. The accompanying JavaScript post provides further details on the 3D builder. The possibilities are vast!
Frequently Asked Questions (FAQs) (This section remains largely unchanged from the input, as it provides valuable supplementary information.)
How can I start modding Minecraft with PHP?
...
What is the PHP-Minecraft-Query?
...
What is the difference between Minecraft Java and Bedrock editions?
...
Can I implement a Minecraft server in PHP?
...
Can I play Minecraft on Windows?
...
What is the PHP-Minecraft/Minecraft-Query package?
...
How can I use PHP to create buildings in Minecraft?
...
Can I mod Minecraft on consoles or mobile devices?
...
How can I learn PHP for Minecraft modding?
...
Can I share my PHP Minecraft mods with others?
...
The above is the detailed content of Modding Minecraft with PHP - Buildings from Code!. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
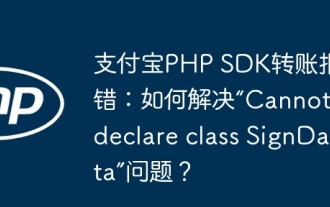
Alipay PHP...
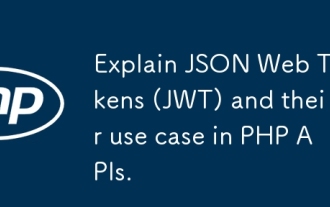
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
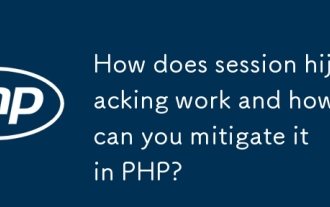
Session hijacking can be achieved through the following steps: 1. Obtain the session ID, 2. Use the session ID, 3. Keep the session active. The methods to prevent session hijacking in PHP include: 1. Use the session_regenerate_id() function to regenerate the session ID, 2. Store session data through the database, 3. Ensure that all session data is transmitted through HTTPS.
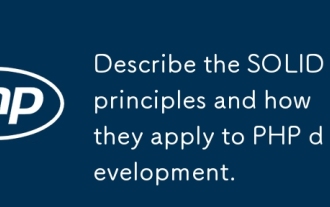
The application of SOLID principle in PHP development includes: 1. Single responsibility principle (SRP): Each class is responsible for only one function. 2. Open and close principle (OCP): Changes are achieved through extension rather than modification. 3. Lisch's Substitution Principle (LSP): Subclasses can replace base classes without affecting program accuracy. 4. Interface isolation principle (ISP): Use fine-grained interfaces to avoid dependencies and unused methods. 5. Dependency inversion principle (DIP): High and low-level modules rely on abstraction and are implemented through dependency injection.
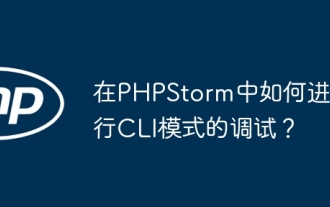
How to debug CLI mode in PHPStorm? When developing with PHPStorm, sometimes we need to debug PHP in command line interface (CLI) mode...
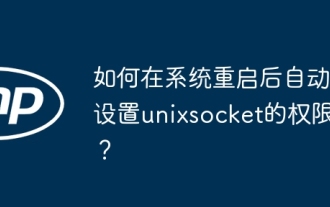
How to automatically set the permissions of unixsocket after the system restarts. Every time the system restarts, we need to execute the following command to modify the permissions of unixsocket: sudo...
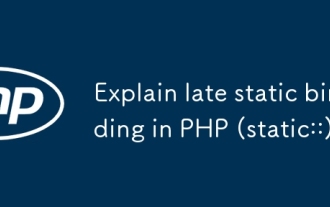
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.
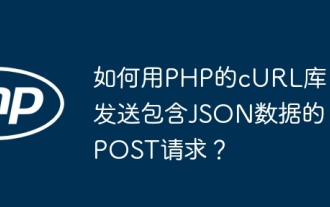
Sending JSON data using PHP's cURL library In PHP development, it is often necessary to interact with external APIs. One of the common ways is to use cURL library to send POST�...
