How to Properly Deploy Web Apps via SFTP with Git
Deploying Web Applications via SFTP with Git: A Secure and Efficient Workflow
This article explores using PHPSECLIB and Git for streamlined and secure SFTP deployments. We'll cover key features, authentication methods, and automation techniques to optimize your deployment process.
Traditional SFTP methods using desktop clients are often cumbersome and inefficient, requiring full project uploads even for minor changes. PHPSECLIB offers a robust solution, leveraging optional PHP extensions or falling back on internal implementation for flexibility. Integrating Git further enhances efficiency by transferring only modified files, saving time and bandwidth.
Key Advantages:
- Secure Transfers: SFTP's encrypted communication ensures data security.
- Efficient Updates: Git's version control allows for uploading only changed files.
- Automated Deployments: Scripting enables automated deployment processes.
- Versatile Functionality: PHPSECLIB supports file uploads, deletions, permission management, and remote command execution.
PHPSECLIB Installation and Authentication:
Install PHPSECLIB using Composer:
1 |
|
PHPSECLIB supports various authentication methods:
- RSA Key: The most secure option, using a private key for authentication.
- Password Protected RSA Key: Supports RSA keys with password protection.
- Username and Password: Less secure and generally discouraged.
Example using RSA Key Authentication:
1 2 3 4 5 6 7 8 9 10 11 12 |
|
File Management (Uploads and Deletions):
Uploading files:
1 2 |
|
Deleting files and directories:
1 2 |
|
Automating Deployment with Git:
Leveraging Git's capabilities minimizes transferred data. A custom Git class can be created to manage Git interactions, such as identifying changed files:
1 2 3 4 5 6 7 8 |
|
The deployment script then uses this Git class and PHPSECLIB to upload/delete files accordingly:
1 2 3 4 5 6 7 8 9 |
|
Remote Command Execution and Permission Management:
PHPSECLIB allows executing commands on the remote server:
1 |
|
Managing file permissions:
1 |
|
Alternatives:
Several alternatives exist for automated SFTP deployments:
git-deploy-php
PHPloy
-
Deploy-Tantra
(commercial)
Conclusion:
Combining PHPSECLIB and Git provides a robust, secure, and efficient solution for SFTP deployments. Automating this process significantly streamlines workflows and reduces manual intervention. Consider the security implications of each authentication method and choose the most appropriate one for your environment. Remember to thoroughly test your deployment scripts before implementing them in a production environment.
(Note: The provided code snippets are simplified examples and may require adjustments based on your specific project setup and environment. Error handling and more robust logic should be added for production use.)
The above is the detailed content of How to Properly Deploy Web Apps via SFTP with Git. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
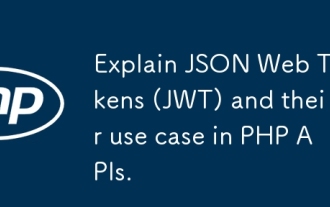
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,

The enumeration function in PHP8.1 enhances the clarity and type safety of the code by defining named constants. 1) Enumerations can be integers, strings or objects, improving code readability and type safety. 2) Enumeration is based on class and supports object-oriented features such as traversal and reflection. 3) Enumeration can be used for comparison and assignment to ensure type safety. 4) Enumeration supports adding methods to implement complex logic. 5) Strict type checking and error handling can avoid common errors. 6) Enumeration reduces magic value and improves maintainability, but pay attention to performance optimization.
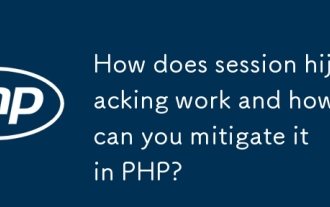
Session hijacking can be achieved through the following steps: 1. Obtain the session ID, 2. Use the session ID, 3. Keep the session active. The methods to prevent session hijacking in PHP include: 1. Use the session_regenerate_id() function to regenerate the session ID, 2. Store session data through the database, 3. Ensure that all session data is transmitted through HTTPS.
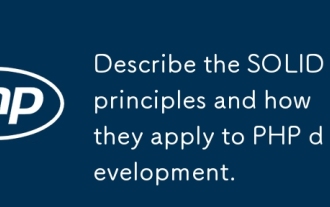
The application of SOLID principle in PHP development includes: 1. Single responsibility principle (SRP): Each class is responsible for only one function. 2. Open and close principle (OCP): Changes are achieved through extension rather than modification. 3. Lisch's Substitution Principle (LSP): Subclasses can replace base classes without affecting program accuracy. 4. Interface isolation principle (ISP): Use fine-grained interfaces to avoid dependencies and unused methods. 5. Dependency inversion principle (DIP): High and low-level modules rely on abstraction and are implemented through dependency injection.
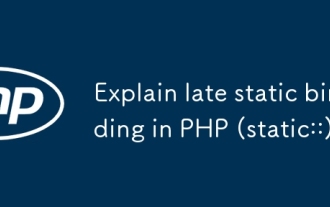
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.
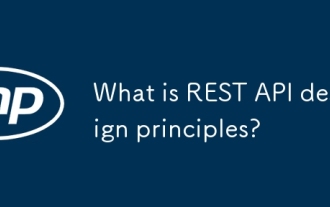
RESTAPI design principles include resource definition, URI design, HTTP method usage, status code usage, version control, and HATEOAS. 1. Resources should be represented by nouns and maintained at a hierarchy. 2. HTTP methods should conform to their semantics, such as GET is used to obtain resources. 3. The status code should be used correctly, such as 404 means that the resource does not exist. 4. Version control can be implemented through URI or header. 5. HATEOAS boots client operations through links in response.
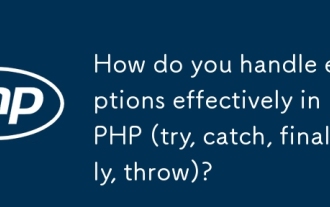
In PHP, exception handling is achieved through the try, catch, finally, and throw keywords. 1) The try block surrounds the code that may throw exceptions; 2) The catch block handles exceptions; 3) Finally block ensures that the code is always executed; 4) throw is used to manually throw exceptions. These mechanisms help improve the robustness and maintainability of your code.
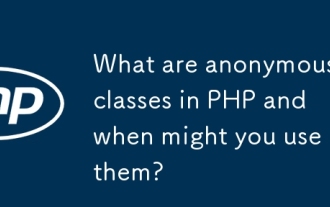
The main function of anonymous classes in PHP is to create one-time objects. 1. Anonymous classes allow classes without names to be directly defined in the code, which is suitable for temporary requirements. 2. They can inherit classes or implement interfaces to increase flexibility. 3. Pay attention to performance and code readability when using it, and avoid repeatedly defining the same anonymous classes.
