Build Your Own Dropbox Client with the Dropbox API
Dropbox: Build a custom Dropbox client with PHP and Laravel
Dropbox stands out among a wide range of file hosting solutions with its simplicity, automatic synchronization capabilities, cross-platform support, and other powerful features.
As a PHP developer, you can make the most of the Dropbox API to create applications to implement various operations of your Dropbox account. This tutorial will use Dropbox API v2. If you want to do it, you can clone the project from Github.
Core points
- Use Dropbox API v2 to build a custom Dropbox client in combination with PHP and Laravel to control user file operations.
- First register a new application on the Dropbox developer website and get the necessary credentials such as App Key and Secret, which are crucial for API authentication.
- Configure the Laravel environment to integrate Dropbox by setting the necessary routing and middleware to handle user authentication and file operations.
- Install and use Guzzle, Purl and Carbon libraries in Laravel to handle HTTP requests, URL operations, and date/time conversions respectively.
- Enable various functions such as file upload, download, search and version management through specific API endpoints, and use token-based access.
- Protect your application by setting the appropriate permissions (folder access or full Dropbox access) and using OAuth2 for user authentication and authorization.
- Explore advanced features such as webhooks for real-time file update notifications, as well as embedded tools such as Chooser and Saver for enhanced user interaction.
Create Dropbox app
First, visit the Dropbox developer website and create a new application.
Dropbox offers two APIs: the public-facing Dropbox API and the team-facing Business API. The two APIs are nearly identical, the only difference is that the Business API is specifically for enterprise accounts, so it includes team features by default such as access to team information, team member file access, and team member management. We will use the former.
After creating the application, you will see the application settings page:
Here, you can set the following:
- Development Users – This allows you to add Dropbox users to test your application. By default, the application status is "Development". This means that only you can test its functionality. If you allow any other user to access your app, they will not be able to use it.
- Permission Type – This is the setting you selected when creating the application. There are only two permission types: folder and full Dropbox. Folders means that your application can only access the folders you specify. Full Dropbox means that your app can access all users’ files.
- App Key and Secret – This is the only key that Dropbox uses to identify your application. We will need it later.
- OAuth2 Redirect URLs – Here you can set URLs, and your application can redirect to these URLs after the user authorizes the necessary permissions. Now leave blank first, you will add the value later. Now note that only the URL you specified here can be used for redirects.
- Allow implicit authorization – Whether to automatically generate an access token after the user grants the necessary permissions to your application. If you are using Dropbox on the client, you should set it to "Allow" so that you can get access tokens via JavaScript. For this project, you should set it to "Don't allow".
- Generated Access Token – You can use this token to generate an access token for your account. Access tokens can be used to make requests to the API.
- Chooser/Saver Domain Name – If you use embedded tools such as Chooser and Saver, you need to specify the domain name to embed these tools here.
- Webhooks – If you want the server to perform specific actions when a file in the user's Dropbox account changes, you can use Webhooks. In this tutorial, we will not cover Webhooks, so if you need this feature in your application, it is recommended that you check out the Webhooks documentation.
Build the application
Now you can start building the application. We will use Laravel.
Installing dependencies
composer create-project --prefer-dist laravel/laravel pinch
After the installation is complete, you also need to install Guzzle, Purl, and Carbon.
composer require nesbot/carbon jwage/purl guzzlehttp/guzzle
We will use Guzzle to issue HTTP requests to the Dropbox API, build the Dropbox login URL with Purl, and use Carbon to represent the file date in the user time zone.
Configuration
After installing Laravel, open the .env file in the project root directory and add the Dropbox configuration:
<code>DROPBOX_APP_KEY="YOUR DROPBOX APP KEY" DROPBOX_APP_SECRET="YOUR DROPBOX APP SECRET" DROPBOX_REDIRECT_URI="YOUR DROPBOX LOGIN REDIRECT URL"</code>
Use the App Key and App Secret you previously obtained from the Dropbox developer website as the values of DROPBOX_APP_KEY and DROPBOX_APP_SECRET. For DROPBOX_REDIRECT_URI, you have to specify an http URL, so if you are using a virtual host you need to use a tool like Ngrok to provide the service. Then, in your virtual host configuration, add the URL provided by Ngrok as ServerAlias.
<virtualhost *:80> ServerName pinch.dev ServerAlias xxxxxxx.ngrok.io ServerAdmin wern@localhost DocumentRoot /home/wern/www/pinch/public </virtualhost>
...(The rest of the parts are the same as the original text, and the length is too long, omitted here)....
The above is the detailed content of Build Your Own Dropbox Client with the Dropbox API. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics




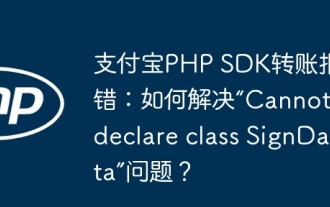
Alipay PHP...
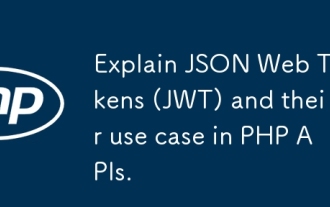
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
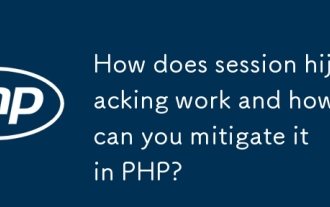
Session hijacking can be achieved through the following steps: 1. Obtain the session ID, 2. Use the session ID, 3. Keep the session active. The methods to prevent session hijacking in PHP include: 1. Use the session_regenerate_id() function to regenerate the session ID, 2. Store session data through the database, 3. Ensure that all session data is transmitted through HTTPS.
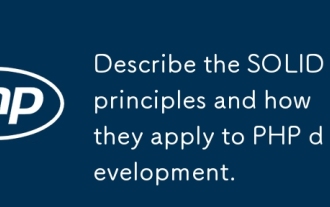
The application of SOLID principle in PHP development includes: 1. Single responsibility principle (SRP): Each class is responsible for only one function. 2. Open and close principle (OCP): Changes are achieved through extension rather than modification. 3. Lisch's Substitution Principle (LSP): Subclasses can replace base classes without affecting program accuracy. 4. Interface isolation principle (ISP): Use fine-grained interfaces to avoid dependencies and unused methods. 5. Dependency inversion principle (DIP): High and low-level modules rely on abstraction and are implemented through dependency injection.
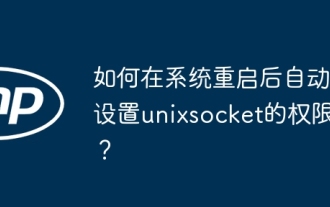
How to automatically set the permissions of unixsocket after the system restarts. Every time the system restarts, we need to execute the following command to modify the permissions of unixsocket: sudo...
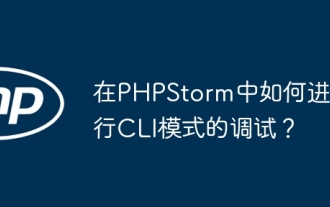
How to debug CLI mode in PHPStorm? When developing with PHPStorm, sometimes we need to debug PHP in command line interface (CLI) mode...
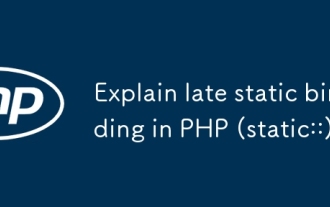
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.
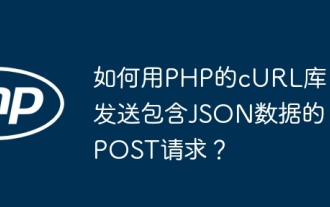
Sending JSON data using PHP's cURL library In PHP development, it is often necessary to interact with external APIs. One of the common ways is to use cURL library to send POST�...
