Building Microsoft's What-Dog AI in under 100 Lines of Code
This tutorial shows you how to build a dog breed identifier similar to Microsoft's What-Dog AI, but using Diffbot's Image API. The entire application is less than 100 lines of code and leverages Imgur for image hosting to minimize costs.
Key Features:
- Uses a simple image upload form and PHP for processing.
- Diffbot's Image API analyzes uploaded images and returns breed suggestions based on identified tags.
- While not perfect, the resulting application demonstrates the accessibility and potential of modern AI for image recognition.
Getting Started:
- Diffbot Account: Obtain a free 14-day API token from Diffbot.com.
-
Composer Setup: Use the following
composer.json
to install the necessary libraries:
{ "require": { "swader/diffbot-php-client": "^2", "php-http/guzzle6-adapter": "^1.0" }, "minimum-stability": "dev", "prefer-stable": true, "require-dev": { "symfony/var-dumper": "^3.0" } }
<code>Run `composer install`. The `minimum-stability` setting accommodates a beta dependency.</code>
- Imgur Account: Create an Imgur account and obtain a Client ID for anonymous image uploads.
Code Structure (index.php):
The core logic resides in index.php
. The code first handles image uploads via an HTML form (omitted for brevity, focusing on the PHP backend). Imgur is used for hosting, saving on server costs. The uploaded image URL is then sent to Diffbot's Image API.
<?php require 'vendor/autoload.php'; $token = 'YOUR_DIFFBOT_TOKEN'; // Replace with your Diffbot token $imgur_client = 'YOUR_IMGUR_CLIENT_ID'; // Replace with your Imgur Client ID if ($_SERVER['REQUEST_METHOD'] == 'POST') { // Handle image upload (using $_FILES) or URL submission (using $_POST['url']) // ... (Image upload to Imgur using Guzzle, obtaining the image URL) ... if (!isset($url) || empty($url)) { die("Image upload or URL submission failed."); } $diffbot = new Swader\Diffbot\Diffbot($token); $imageDetails = $diffbot->createImageAPI($url)->call(); $tags = $imageDetails->getTags(); echo "<img src=\"{$url}\" style="max-width:90%"500\" alt="Building Microsoft's What-Dog AI in under 100 Lines of Code" ></img>"; if (empty($tags)) { echo "<h4 id="No-breed-identified">No breed identified.</h4>"; } else { echo "<h4 id="Suggested-Breed-s">Suggested Breed(s):</h4>"; foreach ($tags as $tag) { echo "- <a href=\"https://www.bing.com/images/search?q=" . urlencode($tag['label']) . "\" target=\"_blank\">" . $tag['label'] . "</a><br>"; } } } ?> <!-- HTML form for image upload or URL input -->
Functions (Helper Functions):
The code uses helper functions (not shown above) to create links to Bing image search results for each suggested breed.
Testing and Results:
The tutorial includes several test images and their results, highlighting both successes and failures of the breed identification. The accuracy is comparable to Microsoft's What-Dog AI, demonstrating the feasibility of building a similar application with Diffbot.
Conclusion:
This tutorial showcases the ease of integrating AI-powered image analysis into a simple web application. While the accuracy isn't perfect, it highlights the potential of readily available APIs for building powerful image recognition features. Remember to replace placeholder tokens and IDs with your own.
The above is the detailed content of Building Microsoft's What-Dog AI in under 100 Lines of Code. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










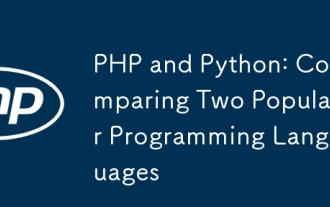
PHP and Python each have their own advantages, and choose according to project requirements. 1.PHP is suitable for web development, especially for rapid development and maintenance of websites. 2. Python is suitable for data science, machine learning and artificial intelligence, with concise syntax and suitable for beginners.
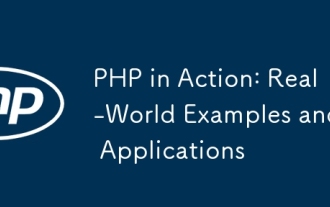
PHP is widely used in e-commerce, content management systems and API development. 1) E-commerce: used for shopping cart function and payment processing. 2) Content management system: used for dynamic content generation and user management. 3) API development: used for RESTful API development and API security. Through performance optimization and best practices, the efficiency and maintainability of PHP applications are improved.
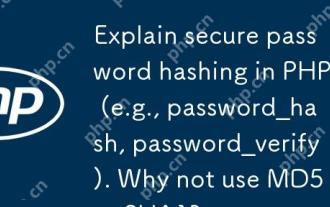
In PHP, password_hash and password_verify functions should be used to implement secure password hashing, and MD5 or SHA1 should not be used. 1) password_hash generates a hash containing salt values to enhance security. 2) Password_verify verify password and ensure security by comparing hash values. 3) MD5 and SHA1 are vulnerable and lack salt values, and are not suitable for modern password security.
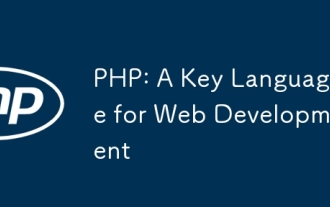
PHP is a scripting language widely used on the server side, especially suitable for web development. 1.PHP can embed HTML, process HTTP requests and responses, and supports a variety of databases. 2.PHP is used to generate dynamic web content, process form data, access databases, etc., with strong community support and open source resources. 3. PHP is an interpreted language, and the execution process includes lexical analysis, grammatical analysis, compilation and execution. 4.PHP can be combined with MySQL for advanced applications such as user registration systems. 5. When debugging PHP, you can use functions such as error_reporting() and var_dump(). 6. Optimize PHP code to use caching mechanisms, optimize database queries and use built-in functions. 7
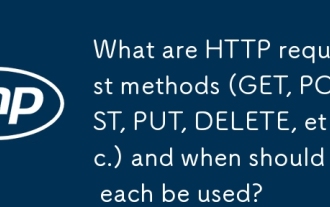
HTTP request methods include GET, POST, PUT and DELETE, which are used to obtain, submit, update and delete resources respectively. 1. The GET method is used to obtain resources and is suitable for read operations. 2. The POST method is used to submit data and is often used to create new resources. 3. The PUT method is used to update resources and is suitable for complete updates. 4. The DELETE method is used to delete resources and is suitable for deletion operations.
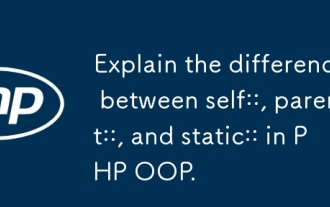
In PHPOOP, self:: refers to the current class, parent:: refers to the parent class, static:: is used for late static binding. 1.self:: is used for static method and constant calls, but does not support late static binding. 2.parent:: is used for subclasses to call parent class methods, and private methods cannot be accessed. 3.static:: supports late static binding, suitable for inheritance and polymorphism, but may affect the readability of the code.
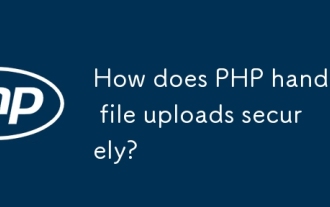
PHP handles file uploads through the $\_FILES variable. The methods to ensure security include: 1. Check upload errors, 2. Verify file type and size, 3. Prevent file overwriting, 4. Move files to a permanent storage location.
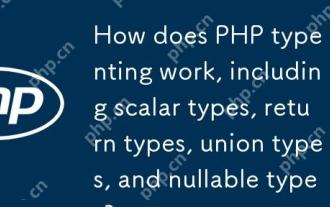
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.
