How to Enrich Data with MongoDB Stitch
This tutorial demonstrates enriching MongoDB documents with data from an external API using MongoDB Stitch. We'll add movie details from the OMDB API to a MongoDB document after initial insertion.
Goal: This tutorial shows how to:
- Insert a document into MongoDB using a MongoDB Stitch HTTP POST service. The initial document will contain only an
_id
and aTitle
. - Create a Stitch trigger that activates upon new document insertion.
- Use the trigger to call the OMDB API with the movie title.
- Update the original MongoDB document with the fetched movie details.
Prerequisites:
You'll need a free MongoDB Atlas cluster. A video tutorial outlining the setup process is available (link presumably provided in the original). Then, link a MongoDB Stitch application to your Atlas cluster:
- Navigate to "Stitch Apps" in the left panel.
- Click "Create New Application."
- Name your application.
- Link it to your MongoDB Atlas cluster.
Setting up the HTTP POST Service:
- In the left panel, go to "Services," then "Add a Service."
- Name the service "IMDB" (or choose another name; remember to update the code accordingly).
- Add an Incoming Webhook and note the following configuration (screenshot provided in original).
The following function code will handle the initial document insertion:
exports = function(payload, response) { const mongodb = context.services.get("mongodb-atlas"); const movies = mongodb.db("stitch").collection("movies"); var body = EJSON.parse(payload.body.text()); movies.insertOne(body) .then(result => { response.setStatusCode(201); }); };
Save the function. Test it using a curl
command (or Postman) like this, replacing the placeholder URL and secret:
curl -H "Content-Type: application/json" -d '{"Title":"Guardians of the Galaxy"}' https://webhooks.mongodb-stitch.com/api/client/v2.0/app/stitchtapp-abcde/service/IMDB/incoming_webhook/post_movie_title?secret=test
Verify the insertion in your MongoDB Atlas cluster.
Creating the Trigger and Enrichment Function:
- In the left panel, go to "Triggers," then "Add a Database Trigger."
- Configure the trigger as shown in the original (screenshot provided).
- Use the following function code to fetch and add movie details from the OMDB API:
exports = function(changeEvent) { var docId = changeEvent.documentKey._id; var title = encodeURIComponent(changeEvent.fullDocument.Title.trim()); var movies = context.services.get("mongodb-atlas").db("stitch").collection("movies"); var imdb_url = "http://www.omdbapi.com/?apikey=[YOUR_OMDB_API_KEY]&t=" + title; const http = context.services.get("IMDB"); return http .get({ url: imdb_url }) .then(resp => { var doc = EJSON.parse(resp.body.text()); movies.updateOne({"_id":docId}, {$set: doc}); // Use $set to update only the new fields }); };
Remember to replace [YOUR_OMDB_API_KEY]
with your actual OMDB API key (obtain one from https://www.php.cn/link/fcf70ea0bbeb4edca72cc304e75f4c98). The $set
operator is used to prevent overwriting existing fields.
Test the trigger by sending another curl
request. The updated document should now contain the enriched movie data.
Summary:
This process demonstrates a powerful way to integrate external APIs with your MongoDB data using MongoDB Stitch's serverless capabilities. The event-driven architecture allows for efficient data enrichment without complex server-side logic.
Further Reading:
- MongoDB Stitch Billing: (link presumably provided in the original)
- Querying MongoDB Atlas with MongoDB Stitch: (link presumably provided in the original)
The above is the detailed content of How to Enrich Data with MongoDB Stitch. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










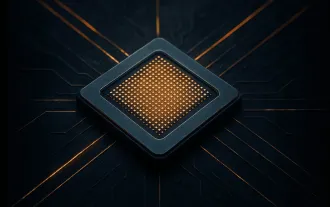
This pilot program, a collaboration between the CNCF (Cloud Native Computing Foundation), Ampere Computing, Equinix Metal, and Actuated, streamlines arm64 CI/CD for CNCF GitHub projects. The initiative addresses security concerns and performance lim
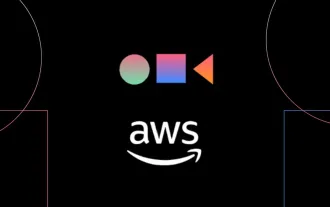
This tutorial guides you through building a serverless image processing pipeline using AWS services. We'll create a Next.js frontend deployed on an ECS Fargate cluster, interacting with an API Gateway, Lambda functions, S3 buckets, and DynamoDB. Th
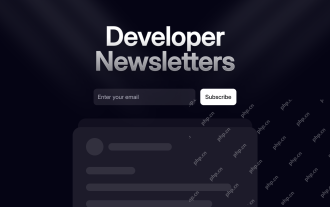
Stay informed about the latest tech trends with these top developer newsletters! This curated list offers something for everyone, from AI enthusiasts to seasoned backend and frontend developers. Choose your favorites and save time searching for rel
