ES6 Generators and Iterators: a Developer's Guide
ES6 has introduced many new features to the JavaScript language. Two of these features, generators and iterators, have greatly changed the way we write specific functions in more complex front-end code.
While they work well together, their actual functions can be a bit confusing, so let's take a closer look.
Key Points
- ES6 provides a cleaner way to write for loops, providing a more Python-like way to directly interact with elements in the dataset, making the code easier to read and write.
- The generator in ES6 is a function that remembers the state between each call. They can generate the next value in the sequence each time they are called, effectively creating custom iterations.
- The "yield" keyword in the generator function is similar to "return", but it keeps the state of the function, allowing it to continue execution from the interrupt on the next call.
- While Node and modern browsers support ES6 features, older browsers may require translators such as Babel to convert ES6 code to ECMAScript 5 code.
Iterator
Iteration is a common practice in programming, usually used to loop through a set of values, convert each value, or use or save it in some way for later use.
In JavaScript, we have always had such a for loop:
for (var i = 0; i < foo.length; i++) { // 对i执行某些操作 }
But ES6 gives us another option:
for (const i of foo) { // 对i执行某些操作 }
This is arguably more concise and easier to use, reminding me of languages like Python and Ruby. However, there is another very important thing to note about this new iteration: it allows you to interact directly with elements of the dataset.
Suppose we want to find out if each number in the array is a prime number. We can do this by creating a function that does this. It might look like this:
function isPrime(number) { if (number <= 1) { return false; } else if (number === 2) { return true; } for (var i = 2; i < number; i++) { if (number % i === 0) { return false; break; } } return true; }
Not the best in the world, but it works. The next step is to loop through our list of numbers and check if each number is prime using our shiny new function. It's very simple:
var possiblePrimes = [73, 6, 90, 19, 15]; var confirmedPrimes = []; for (var i = 0; i < possiblePrimes.length; i++) { if (isPrime(possiblePrimes[i])) { confirmedPrimes.push(possiblePrimes[i]); } } // confirmedPrimes现在是[73, 19]
Again, it works, but it's clumsy, and this clumsy depends largely on how JavaScript handles for loops. However, with ES6, we get an almost Python-like option in the new iterator. Therefore, the previous for loop can be written like this:
const possiblePrimes = [73, 6, 90, 19, 15]; const confirmedPrimes = []; for (const i of possiblePrimes){ if ( isPrime(i) ){ confirmedPrimes.push(i); } } // confirmedPrimes现在是[73, 19]
This is much cleaner, but the most striking thing is the for loop. The variable i now represents the actual item in an array named possiblePrimes. Therefore, we no longer need to call it by index. This means we don't have to call possiblePrimes[i] in the loop, but just call i.
Behind the scenes, this iteration takes advantage of ES6's shiny new Symbol.iterator() method. This method is responsible for describing iteration and when called, returns a JavaScript object containing the next value in the loop and a done key, which is true or false depending on whether the loop is completed.
If you are interested in this detail, you can read this wonderful blog post "Iterators gonna iterate" by Jake Archibald. As we dig into another part of this article: the generator, it also gives you a good idea of what’s going on behind the scenes.
Generator
The generator (also known as the "iter factory") is a new type of JavaScript function used to create specific iterations. They provide you with a special, custom way to loop through content.
Okay, so what does it mean? Let's look at an example. Suppose we want a function, and each time we call it, we will be given the next prime number:
for (var i = 0; i < foo.length; i++) { // 对i执行某些操作 }
If you're used to JavaScript, some of this stuff looks a bit like witchcraft, but it's not too bad in reality. We have that weird asterisk after the keyword function, but that just tells JavaScript that we are defining a generator.
Another strange part is the yield keyword. This is actually what the generator spits out when you call it. It roughly equivalent to return, but it retains the state of the function instead of rerunning everything every time it is called. It "remember" its location at runtime, so the next time you call it, it continues from the interrupt.
This means we can do this:
for (const i of foo) { // 对i执行某些操作 }
Then, whenever we want to get - you guessed it - the next prime number, we can call nextPrime:
function isPrime(number) { if (number <= 1) { return false; } else if (number === 2) { return true; } for (var i = 2; i < number; i++) { if (number % i === 0) { return false; break; } } return true; }
You can also just call nextPrime.next(), which is useful when your generator is not infinite, as it returns an object like this:
var possiblePrimes = [73, 6, 90, 19, 15]; var confirmedPrimes = []; for (var i = 0; i < possiblePrimes.length; i++) { if (isPrime(possiblePrimes[i])) { confirmedPrimes.push(possiblePrimes[i]); } } // confirmedPrimes现在是[73, 19]
Here, the done key tells you whether the function has completed its task. In our case, our function never ends, which theoretically gives us all prime numbers up to infinity (if we have that much computer memory).
It's cool, so can I use generators and iterators now?
Although ECMAScript 2015 has been completed and has been around for many years, browser support for its features (especially generators) is far from perfect. If you really want to use these and other modern features, you can look at translators like Babel and Traceur that will convert your ECMAScript 2015 code into equivalent (if possible) ECMAScript 5 code.
There are also many online editors that support ECMAScript 2015, or focus specifically on it, especially Facebook's Regenerator and JS Bin. If you just want to play and learn how JavaScript is written now, these are worth a look.
Conclusion
Generators and iterators provide quite a lot of new flexibility for our approach to JavaScript problems. Iterators allow us to write for loops in a more Python-like way, which means our code looks cleaner and easier to read.
Generator functions allow us to write functions that remember where they were last seen, and can continue execution from the interrupt. They can also be infinite in terms of what they actually remember, which is very convenient in some cases.
The support for these generators and iterators is good. They are supported in Node and in all modern browsers, except for Internet Explorer. If you need to support older browsers, the best way is to use a translator like Babel.
FAQs about ECMAScript 2015 Generators and Iterators (FAQ)
What is the difference between iterators and generators in ECMAScript 2015?
Iterators and generators are both features of ECMAScript 2015 and are used to process data flows. An iterator is an object that allows the programmer to iterate through all elements in a collection. It has a next() method that returns the next item in the sequence. On the other hand, the generator is a function that can stop halfway and then continue from the stop. In other words, the generator looks like a function, but it behaves like an iterator.
How to use yield keyword in ECMAScript 2015?
yield keyword is used for ECMAScript 2015 pause and restore generator functions (function* or legacy generator functions). yield can return a value from the generator function. This return value is usually an object with two properties: value and done. The value attribute is the result of calculating the yield expression, and done is a boolean value indicating whether the generator has generated its last value.
What is the purpose of the next() method in ECMAScript 2015?
The next() method is a key part of the iterator protocol in ECMAScript 2015. It returns an object with two properties: value and done. The value attribute is the next value in the iteration sequence, and done is a boolean value indicating whether the iteration is completed. If done is true, the iterator has exceeded the end of the iteration sequence.How to use generators for asynchronous programming in ECMAScript 2015?
The generator in ECMAScript 2015 can be used to simplify asynchronous programming. They can be used to block execution to wait for the asynchronous operation to complete without blocking the entire program. This can make the asynchronous code look and behave more like synchronous code, which is easier to understand and reason about.
What is the difference between for…of loop and for…in loop in ECMAScript 2015?
The for…of loop in ECMAScript 2015 is used to loop through iterable objects such as arrays, strings, maps, collections, etc. It calls custom iterative hooks using statements that will be executed for the value of each different attribute. On the other hand, the for…in loop is used to loop through the properties of an object. It returns a list of keys for the object being iterated.
How to create a custom iterator in ECMAScript 2015?
In ECMAScript 2015, you can create a custom iterator by defining an object with the next() method. This method should return an object with two properties: value and done. The value attribute is the next value in the iteration sequence, and done is a boolean value indicating whether the iteration is completed.
What is the role of Symbol.iterator in ECMAScript 2015?
Symbol.iterator is a special built-in symbol in ECMAScript 2015. It is used to specify the default iterator for the object. When an object needs to be iterated (for example at the beginning of a for…of loop), its @@iterator method will be called without any arguments, and the returned iterator will be used to get the value to iterate.
Can you provide an example of generator functions in ECMAScript 2015?
Of course, this is a simple example of generator functions in ECMAScript 2015:
for (var i = 0; i < foo.length; i++) { // 对i执行某些操作 }
In this example, the idMaker function is a generator that produces a sequence of numbers.
How to use throw() method and generator in ECMAScript 2015?
The throw() method in ECMAScript 2015 can be used in the generator to restore the execution of the generator function and throw an error from the yield expression. The throw() method can be used to handle errors that occur during the execution of the generator function.
What is the significance of the done attribute in the ECMAScript 2015 iterator?
Thedone property is a boolean value returned by the iterator in ECMAScript 2015. It indicates whether the iterator has more values to return. If done is true, the iterator has exceeded the end of the iteration sequence. If done is false, the iterator can still generate more values.
The above is the detailed content of ES6 Generators and Iterators: a Developer's Guide. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


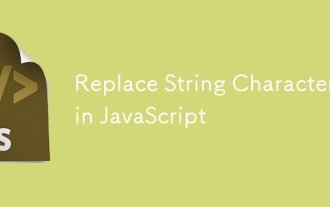
Detailed explanation of JavaScript string replacement method and FAQ This article will explore two ways to replace string characters in JavaScript: internal JavaScript code and internal HTML for web pages. Replace string inside JavaScript code The most direct way is to use the replace() method: str = str.replace("find","replace"); This method replaces only the first match. To replace all matches, use a regular expression and add the global flag g: str = str.replace(/fi
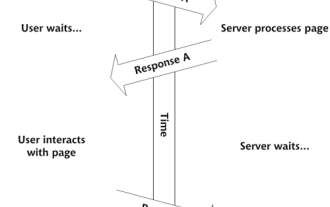
So here you are, ready to learn all about this thing called AJAX. But, what exactly is it? The term AJAX refers to a loose grouping of technologies that are used to create dynamic, interactive web content. The term AJAX, originally coined by Jesse J
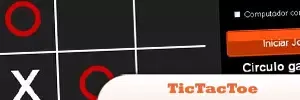
10 fun jQuery game plugins to make your website more attractive and enhance user stickiness! While Flash is still the best software for developing casual web games, jQuery can also create surprising effects, and while not comparable to pure action Flash games, in some cases you can also have unexpected fun in your browser. jQuery tic toe game The "Hello world" of game programming now has a jQuery version. Source code jQuery Crazy Word Composition Game This is a fill-in-the-blank game, and it can produce some weird results due to not knowing the context of the word. Source code jQuery mine sweeping game
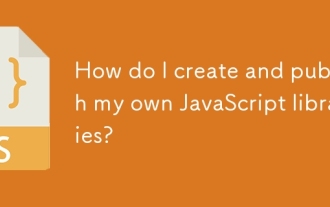
Article discusses creating, publishing, and maintaining JavaScript libraries, focusing on planning, development, testing, documentation, and promotion strategies.
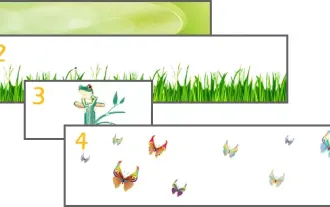
This tutorial demonstrates how to create a captivating parallax background effect using jQuery. We'll build a header banner with layered images that create a stunning visual depth. The updated plugin works with jQuery 1.6.4 and later. Download the
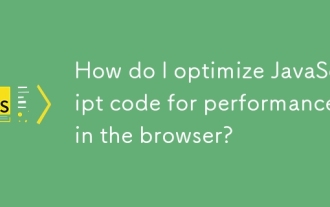
The article discusses strategies for optimizing JavaScript performance in browsers, focusing on reducing execution time and minimizing impact on page load speed.
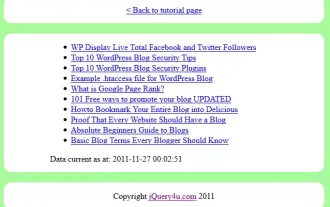
This article demonstrates how to automatically refresh a div's content every 5 seconds using jQuery and AJAX. The example fetches and displays the latest blog posts from an RSS feed, along with the last refresh timestamp. A loading image is optiona
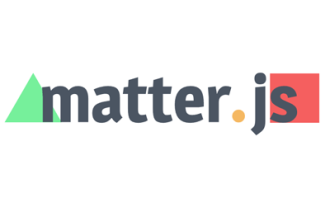
Matter.js is a 2D rigid body physics engine written in JavaScript. This library can help you easily simulate 2D physics in your browser. It provides many features, such as the ability to create rigid bodies and assign physical properties such as mass, area, or density. You can also simulate different types of collisions and forces, such as gravity friction. Matter.js supports all mainstream browsers. Additionally, it is suitable for mobile devices as it detects touches and is responsive. All of these features make it worth your time to learn how to use the engine, as this makes it easy to create a physics-based 2D game or simulation. In this tutorial, I will cover the basics of this library, including its installation and usage, and provide a
