A Beginner's Guide to Babel
Babel: Your Gateway to Modern JavaScript Development
This article explores Babel, a powerful JavaScript compiler that bridges the gap between cutting-edge JavaScript features and older browser compatibility. Frustration often arises when developing web applications due to inconsistencies in browser support for newer JavaScript features. Babel acts as a transpiler, converting modern JavaScript (ES2015 ) into compatible code for older browsers, allowing developers to focus on writing clean, efficient code without compatibility concerns.
Key Advantages:
- Modern JavaScript Today: Utilize the latest JavaScript features immediately, regardless of browser limitations.
- Simplified Development: Concentrate on writing optimal code without being bogged down by browser compatibility checks.
- Extensive Ecosystem: Leverage a rich collection of presets and plugins to extend Babel's functionality and incorporate non-standard features.
- Future-Proofing: Utilize features not yet standardized in the language, ensuring your code remains adaptable to future JavaScript updates.
Getting Started with Babel (CLI):
Setting up Babel via the command line interface (CLI) is straightforward. These steps assume a functional Node.js environment:
-
Project Setup: Create a project directory and initialize an npm project:
mkdir babel-project cd babel-project npm init -y
Copy after loginCopy after login -
Install Babel: Install the Babel CLI as a development dependency:
npm install --save-dev @babel/cli @babel/core
Copy after loginCopy after login -
Configure Babel (
.babelrc
): Create a.babelrc
file in your project's root directory to specify presets and plugins. The@babel/preset-env
preset automatically selects necessary plugins based on your target browsers:{ "presets": ["@babel/preset-env"] }
Copy after loginCopy after login -
Install Preset: Install the
@babel/preset-env
preset:npm install --save-dev @babel/preset-env
Copy after login -
Add Build Script (package.json): Add a build script to your
package.json
file:"scripts": { "build": "babel src -d dist" }
Copy after loginThis script processes files in the
src
directory and outputs the transpiled code to thedist
directory. -
Create Directories: Create the
src
anddist
directories:mkdir src dist
Copy after login -
Write Your Code: Create a JavaScript file (e.g.,
src/main.js
) using modern JavaScript syntax. -
Run Babel: Execute the build script:
npm run build
Copy after loginThis will transpile your code.
Targeting Specific Browsers:
Fine-tune browser compatibility by specifying target browsers within the .babelrc
file:
mkdir babel-project cd babel-project npm init -y
Babel Ecosystem:
Babel's strength lies in its extensive ecosystem of presets and plugins. Presets are collections of plugins, simplifying configuration. Plugins add specific transformations or features. Key presets include @babel/preset-env
(for ES2015 features), @babel/preset-react
(for JSX), and @babel/preset-typescript
(for TypeScript).
Babel Polyfill:
For features that require runtime polyfills (e.g., Promises), use the Babel polyfill, which includes core-js
and the Regenerator runtime.
Advanced Usage:
Babel can also handle features in the proposal stage, such as class fields. To use class fields, install @babel/plugin-proposal-class-properties
:
npm install --save-dev @babel/cli @babel/core
Update your .babelrc
to include the plugin:
{ "presets": ["@babel/preset-env"] }
Alternatives:
While Babel is a powerful tool, other options exist, such as TypeScript, which adds static typing to JavaScript.
Conclusion:
Babel empowers developers to write modern, maintainable JavaScript code while ensuring broad browser compatibility. Its flexibility and extensive ecosystem make it an indispensable tool for modern web development. The FAQs section below addresses common questions about Babel's usage and configuration.
Frequently Asked Questions (FAQs):
(The FAQs section from the original input has been retained and slightly reformatted for better readability.)
What is the purpose of Babel in JavaScript development? Babel is a JavaScript compiler that transforms syntax, enabling developers to use next-generation JavaScript features while maintaining compatibility with older browsers. It converts ECMAScript 2015 code into a backwards-compatible version.
How do I install and set up Babel? Install using Node.js and npm: npm install --save-dev @babel/core @babel/cli
. Create a .babelrc
file to specify presets.
What are Babel presets and plugins? Presets are collections of plugins. Plugins add specific features or transformations. @babel/preset-env
is a commonly used preset.
How do I use Babel with Webpack? Use babel-loader
to transpile JavaScript files within your Webpack configuration.
How does Babel handle polyfills? Babel uses core-js
for polyfills, automatically importing necessary polyfills for unsupported features.
Can I use Babel with React? Yes, use @babel/preset-react
to transform JSX.
How do I debug Babel issues? Check the transpiled code and use source maps to debug.
How do I configure Babel for browser compatibility? Use @babel/preset-env
and specify target browsers.
Can I use Babel with TypeScript? Yes, use @babel/preset-typescript
.
How do I keep Babel up to date? Regularly run npm update
to update Babel and its dependencies.
The above is the detailed content of A Beginner's Guide to Babel. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
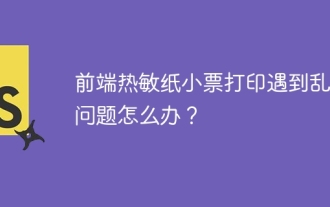
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
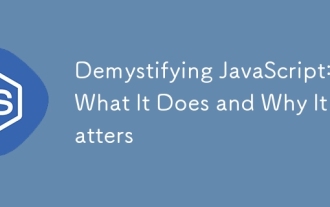
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
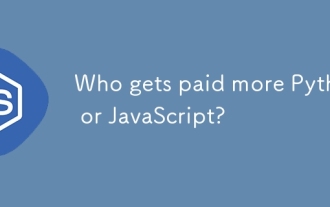
There is no absolute salary for Python and JavaScript developers, depending on skills and industry needs. 1. Python may be paid more in data science and machine learning. 2. JavaScript has great demand in front-end and full-stack development, and its salary is also considerable. 3. Influencing factors include experience, geographical location, company size and specific skills.
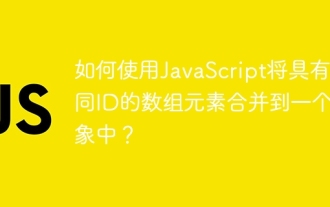
How to merge array elements with the same ID into one object in JavaScript? When processing data, we often encounter the need to have the same ID...
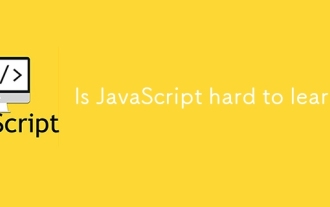
Learning JavaScript is not difficult, but it is challenging. 1) Understand basic concepts such as variables, data types, functions, etc. 2) Master asynchronous programming and implement it through event loops. 3) Use DOM operations and Promise to handle asynchronous requests. 4) Avoid common mistakes and use debugging techniques. 5) Optimize performance and follow best practices.
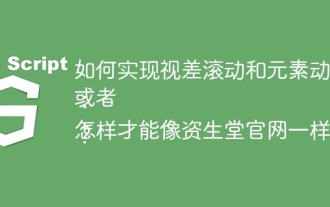
Discussion on the realization of parallax scrolling and element animation effects in this article will explore how to achieve similar to Shiseido official website (https://www.shiseido.co.jp/sb/wonderland/)...
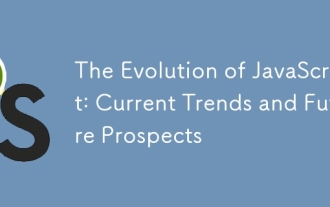
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
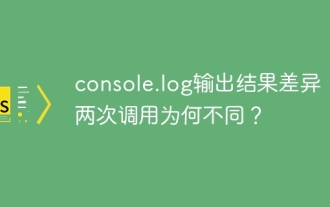
In-depth discussion of the root causes of the difference in console.log output. This article will analyze the differences in the output results of console.log function in a piece of code and explain the reasons behind it. �...
