Laravel Doctrine - Best of Both Worlds?
Laravel Doctrine: A Powerful ORM Alternative to Eloquent
This article explores Laravel Doctrine, a robust Object-Relational Mapper (ORM) offering a compelling alternative to Eloquent in Laravel 5.X and beyond. We'll examine its advantages, installation, entity definition, CRUD operations, and seamless integration with core Laravel features.
Key Advantages of Laravel Doctrine:
- Data Mapper Pattern: Unlike Eloquent's Active Record pattern, Doctrine employs the Data Mapper pattern. This provides superior abstraction, separating business logic from database interactions, leading to improved flexibility and maintainability, especially in complex applications.
- Enhanced Testability: The decoupling inherent in the Data Mapper pattern significantly simplifies testing, as database dependencies are minimized.
- Clean Entity Definitions: Doctrine entities are straightforward PHP classes without base class extensions, using annotations for database mapping. This promotes cleaner, more focused code adhering to the Single Responsibility Principle.
-
Complete CRUD Functionality: The
EntityManager
facilitates effortless CRUD operations, maintaining a clear separation of concerns. - Seamless Laravel Integration: Doctrine integrates smoothly with Laravel's authentication, validation, pagination, and caching mechanisms.
- Advanced Features: Support for event handling and custom repository implementations provides extensive customization options.
Why Choose Doctrine over Eloquent?
Eloquent's Active Record approach, while convenient for smaller projects, can become cumbersome in larger, more complex applications. Its tight coupling between database operations and business logic hinders scalability and testability. Doctrine's Data Mapper pattern offers a more maintainable and robust solution for complex projects.
Installation and Setup:
-
Create a Laravel Project: Use Composer:
composer create-project laravel/laravel Project
-
Install Laravel Doctrine:
composer require "laravel-doctrine/orm:1.1.*"
-
Register Service Provider: Add
LaravelDoctrineORMDoctrineServiceProvider::class
to yourconfig/app.php
file'sproviders
array. -
Register Facades (Optional but Recommended): Add the following to your
config/app.php
file'saliases
array:'EntityManager' => LaravelDoctrine\ORM\Facades\EntityManager::class, 'Registry' => LaravelDoctrine\ORM\Facades\Registry::class, 'Doctrine' => LaravelDoctrine\ORM\Facades\Doctrine::class,
Copy after login - Publish Config File:
php artisan vendor:publish --tag="config"
Example Application: A Simple To-Do List
This section illustrates Doctrine's usage through a basic to-do list application.
Entities:
A Task
entity is defined as a plain PHP class with Doctrine annotations:
<?php namespace TodoList\Entities; use Doctrine\ORM\Mapping as ORM; /** * @ORM\Entity * @ORM\Table(name="tasks") */ class Task { // ... (Entity properties with ORM annotations) ... }
The annotations map class properties to database columns. After defining the entity, generate the database schema: php artisan doctrine:schema:create
EntityManager for CRUD Operations:
The EntityManager
handles persistence. To add a task:
$task = new Task('Task Name', 'Task Description'); EntityManager::persist($task); EntityManager::flush();
Retrieving a task:
$task = EntityManager::find(Task::class, 1);
The complete to-do list application, including adding, editing, deleting, and toggling task statuses, is detailed in the original article. This involves creating controllers, views, and routes, leveraging the EntityManager
and Repository
for database interactions. The example also demonstrates the implementation of user authentication and relationships between users and tasks.
Conclusion:
Laravel Doctrine provides a powerful and flexible alternative to Eloquent, particularly beneficial for complex applications requiring better abstraction, testability, and maintainability. Its seamless integration with Laravel's core features makes it a strong contender for projects demanding a robust ORM solution. The original article provides a comprehensive tutorial on building a full-fledged to-do list application using Laravel Doctrine, showcasing its capabilities and ease of use.
Frequently Asked Questions (FAQs):
The original article already includes a comprehensive FAQ section covering installation, entity definition, CRUD operations, integration with Laravel's authentication, validation, pagination, events, caching, and migrations systems. Refer to that section for detailed answers.
The above is the detailed content of Laravel Doctrine - Best of Both Worlds?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics




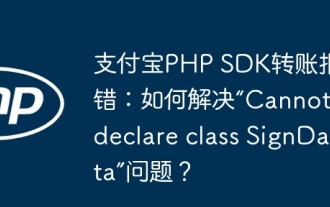
Alipay PHP...
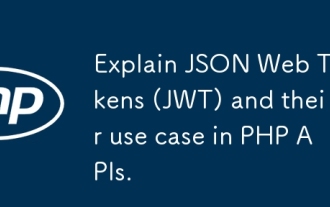
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
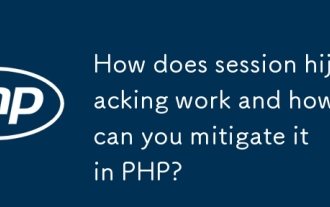
Session hijacking can be achieved through the following steps: 1. Obtain the session ID, 2. Use the session ID, 3. Keep the session active. The methods to prevent session hijacking in PHP include: 1. Use the session_regenerate_id() function to regenerate the session ID, 2. Store session data through the database, 3. Ensure that all session data is transmitted through HTTPS.
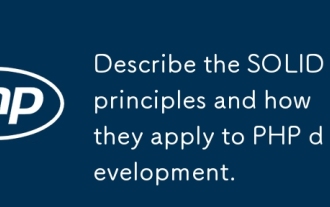
The application of SOLID principle in PHP development includes: 1. Single responsibility principle (SRP): Each class is responsible for only one function. 2. Open and close principle (OCP): Changes are achieved through extension rather than modification. 3. Lisch's Substitution Principle (LSP): Subclasses can replace base classes without affecting program accuracy. 4. Interface isolation principle (ISP): Use fine-grained interfaces to avoid dependencies and unused methods. 5. Dependency inversion principle (DIP): High and low-level modules rely on abstraction and are implemented through dependency injection.
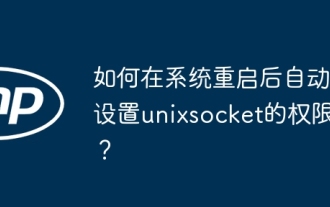
How to automatically set the permissions of unixsocket after the system restarts. Every time the system restarts, we need to execute the following command to modify the permissions of unixsocket: sudo...
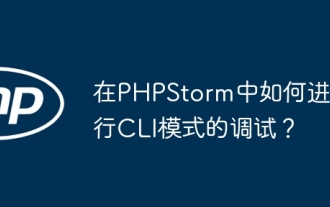
How to debug CLI mode in PHPStorm? When developing with PHPStorm, sometimes we need to debug PHP in command line interface (CLI) mode...
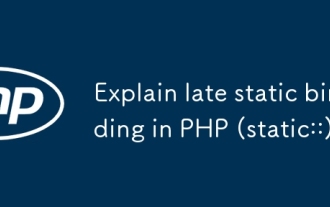
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.
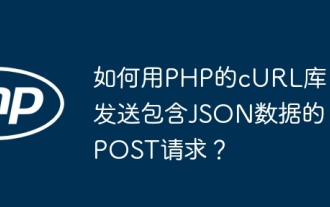
Sending JSON data using PHP's cURL library In PHP development, it is often necessary to interact with external APIs. One of the common ways is to use cURL library to send POST�...
