Build a Superfast PHP Server in Minutes with Icicle
Event-driven programming presents a unique challenge for PHP developers accustomed to procedural coding. In PHP's procedural nature, events often boil down to simple function calls, with no inherent asynchronous behavior. All code execution remains blocking.
However, languages like JavaScript demonstrate the potential of event loops as a central component. This insight has led developers to integrate event loops and asynchronous capabilities into PHP HTTP servers. This article demonstrates building a high-performance PHP HTTP server leveraging the Icicle library, and integrating it with Apache for optimized static file serving. The example code is available at https://www.php.cn/link/ac2ef27277eab81da1d8dea067dd80c1.
Key Advantages
- Icicle's Asynchronous Power: Icicle's asynchronous programming capabilities enable the creation of a remarkably fast PHP server. Concurrent request handling significantly boosts performance.
- Apache Integration for Static Files: Efficiently offloads static file serving to Apache, leaving the PHP Icicle server to handle dynamic requests.
- Routing and Templating Enhancements: Extending the basic server with a router (like LeagueRoute) allows for sophisticated request handling and routing. A template engine (such as LeaguePlates) adds features like layouts and template inheritance for complex applications.
Apache Configuration for Optimized Static File Serving
To avoid unnecessary PHP processing for static files, configure Apache to serve them directly:
RewriteEngine on RewriteCond %{REQUEST_FILENAME} !-f RewriteCond %{REQUEST_FILENAME} !-d RewriteRule ^(.*) http://%{SERVER_NAME}:9001%{REQUEST_URI} [P]
This mod_rewrite
configuration directs Apache to forward requests for non-existent files to a different port (e.g., 9001), where the PHP Icicle server will handle them.
A Basic Icicle HTTP Server
Begin by installing Icicle:
composer require icicleio/http
A simple Icicle HTTP server example:
// server.php require __DIR__ . "/vendor/autoload.php"; use Icicle\Http\Message\RequestInterface; use Icicle\Http\Message\Response; use Icicle\Http\Server\Server; use Icicle\Loop; use Icicle\Socket\Client\ClientInterface; $server = new Server(function (RequestInterface $request, ClientInterface $client) { $response = (new Response(200))->withHeader("Content-Type", "text/plain"); yield $response->getBody()->end("hello world"); yield $response; }); $server->listen(9001); Loop\run();
Advanced Routing with LeagueRoute
For more robust routing, integrate LeagueRoute:
composer require league/route
Enhanced server.php
with routing:
// server.php // ... (previous imports) ... use League\Route\Http\Exception\MethodNotAllowedException; use League\Route\Http\Exception\NotFoundException; use League\Route\RouteCollection; use League\Route\Strategy\UriStrategy; // ... (Server creation) ... $router = new RouteCollection(); $router->setStrategy(new UriStrategy()); require __DIR__ . "/routes.php"; $dispatcher = $router->getDispatcher(); try { $result = $dispatcher->dispatch($request->getMethod(), $request->getRequestTarget()); $status = 200; $content = $result->getContent(); } catch (NotFoundException $e) { $status = 404; $content = "not found"; } catch (MethodNotAllowedException $e) { $status = 405; $content = "method not allowed"; } // ... (Response creation and sending) ...
A sample routes.php
:
$router->addRoute("GET", "/home", function () { return "hello world"; });
Rendering Complex Views with LeaguePlates
For complex views, use LeaguePlates:
composer require league/plates
Implement templating (example snippets from templates/layout.php
and templates/home.php
, and updated routes.php
are omitted for brevity but follow the original example's structure).
Performance Benchmarks and Conclusion
The original article includes performance benchmarks demonstrating the server's capability to handle a significant number of concurrent requests. These benchmarks should be considered in the context of the specific hardware and conditions under which they were run. The key takeaway is the potential for high performance with Icicle's asynchronous model. The article concludes by encouraging experimentation and community discussion. The updated benchmarks provided by the Icicle author are also included. The FAQ section further clarifies various aspects of using Icicle for server development.
The above is the detailed content of Build a Superfast PHP Server in Minutes with Icicle. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics




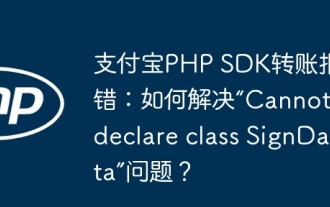
Alipay PHP...
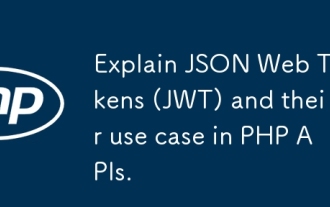
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
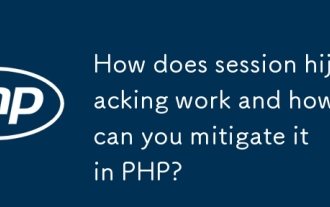
Session hijacking can be achieved through the following steps: 1. Obtain the session ID, 2. Use the session ID, 3. Keep the session active. The methods to prevent session hijacking in PHP include: 1. Use the session_regenerate_id() function to regenerate the session ID, 2. Store session data through the database, 3. Ensure that all session data is transmitted through HTTPS.
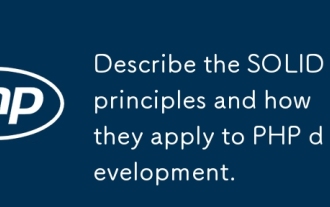
The application of SOLID principle in PHP development includes: 1. Single responsibility principle (SRP): Each class is responsible for only one function. 2. Open and close principle (OCP): Changes are achieved through extension rather than modification. 3. Lisch's Substitution Principle (LSP): Subclasses can replace base classes without affecting program accuracy. 4. Interface isolation principle (ISP): Use fine-grained interfaces to avoid dependencies and unused methods. 5. Dependency inversion principle (DIP): High and low-level modules rely on abstraction and are implemented through dependency injection.
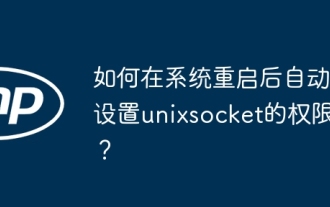
How to automatically set the permissions of unixsocket after the system restarts. Every time the system restarts, we need to execute the following command to modify the permissions of unixsocket: sudo...
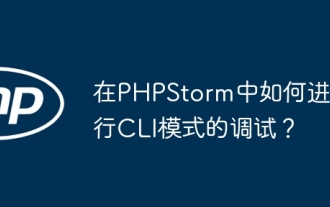
How to debug CLI mode in PHPStorm? When developing with PHPStorm, sometimes we need to debug PHP in command line interface (CLI) mode...
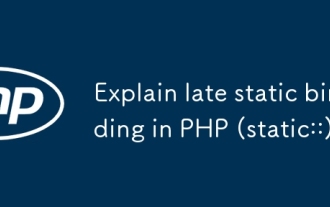
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.
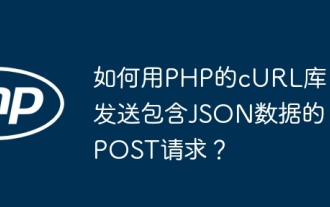
Sending JSON data using PHP's cURL library In PHP development, it is often necessary to interact with external APIs. One of the common ways is to use cURL library to send POST�...
