Converting Your Typographic Units with Sass
Sass: Streamlining Typographic Unit Conversions
This article explores how Sass simplifies typographic unit conversions, eliminating the need for manual calculations. We'll build a Sass function that handles conversions between pixels, ems, percentages, and points.
This article is an updated version of a piece originally published on March 5, 2015.
Historically, web developers often relied on fixed pixel-based layouts. Responsive design has ushered in a more flexible approach, but converting between typographic units (pixels, ems, percentages) remains a common challenge. This often involves tedious manual conversions or consulting conversion charts.
This tutorial demonstrates a Sass function to automate these conversions, saving time and reducing errors.
Prerequisites:
A default font-size must be defined in your CSS (typically 16px). This tutorial assumes a 16px default.
The function will support pixels (px), ems (em), percentages (%), and points (pt).
The Sass Function:
The convert
function takes three arguments:
-
$value
: The numerical value to convert. -
$currentUnit
: The current unit of the value (px, em, %, pt). -
$convertUnit
: The desired unit (px, em, %, pt).
@function convert($value, $currentUnit, $convertUnit) { @if $currentUnit == px { @if $convertUnit == em { @return $value / 16 + 0em; } @else if $convertUnit == % { @return percentage($value / 16); } @else if $convertUnit == pt { @return $value * 1.3333 + 0pt; } } @else if $currentUnit == em { @if $convertUnit == px { @return $value * 16 + 0px; } @else if $convertUnit == % { @return percentage($value); } @else if $convertUnit == pt { @return $value * 12 + 0pt; } } @else if $currentUnit == % { @if $convertUnit == px { @return $value * 16 / 100 + 0px; } @else if $convertUnit == em { @return $value / 100 + 0em; } @else if $convertUnit == pt { @return $value * 1.3333 * 16 / 100 + 0pt; } } @else if $currentUnit == pt { @if $convertUnit == px { @return $value * 1.3333 + 0px; } @else if $convertUnit == em { @return $value / 12 + 0em; } @else if $convertUnit == % { @return percentage($value / 12); } } }
Usage:
.foo { font-size: convert(16, px, em); // Returns 1em } .bar { font-size: convert(100, %, px); // Returns 16px }
Extending the Function:
This function can be further enhanced by adding:
- Support for rem units.
- Error handling for invalid inputs.
- Default unit settings.
Frequently Asked Questions (FAQs):
This section addresses common questions regarding CSS, Sass, and typographic unit conversions. The answers are similar to the original, but rephrased for clarity and conciseness.
- CSS vs. Sass: CSS is a style sheet language; Sass is a preprocessor that compiles to CSS, offering features like variables and nesting for improved code organization and maintainability.
- Converting CSS to Sass: Online tools or manual conversion can translate CSS to Sass.
- Using Sass in existing CSS projects: Sass is compatible with CSS and can be gradually integrated.
-
Compiling Sass to CSS: A Sass compiler (like Dart Sass) is needed to compile
.scss
files to.css
. - Benefits of Sass over CSS: Sass offers variables, nesting, mixins, and functions for better code organization, reusability, and maintainability.
-
Typographic units in Sass: These include
px
,em
,rem
,pt
, and%
. - Converting typographic units in Sass: Use built-in Sass functions or create custom functions like the one shown above.
- Using CSS functions in Sass: Sass supports all CSS functions and adds its own.
-
Using variables in Sass: Declare variables using
$variable-name: value;
. -
Mixins in Sass: Reusable blocks of styles defined with
@mixin
and included with@include
.
This improved response provides a more concise and well-structured explanation of the Sass function, while retaining the key information and addressing the FAQs. The image is included as requested.
The above is the detailed content of Converting Your Typographic Units with Sass. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


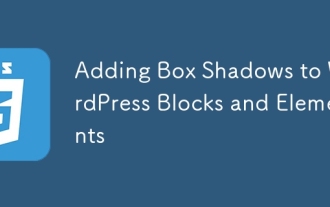
The CSS box-shadow and outline properties gained theme.json support in WordPress 6.1. Let's look at a few examples of how it works in real themes, and what options we have to apply these styles to WordPress blocks and elements.
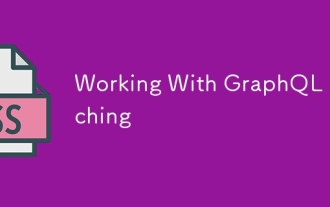
If you’ve recently started working with GraphQL, or reviewed its pros and cons, you’ve no doubt heard things like “GraphQL doesn’t support caching” or
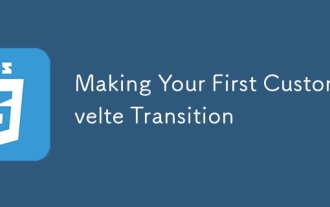
The Svelte transition API provides a way to animate components when they enter or leave the document, including custom Svelte transitions.
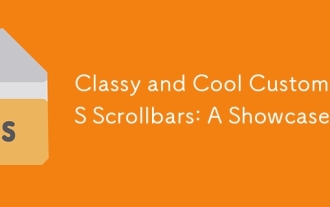
In this article we will be diving into the world of scrollbars. I know, it doesn’t sound too glamorous, but trust me, a well-designed page goes hand-in-hand
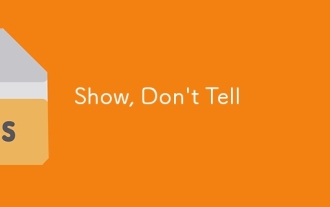
How much time do you spend designing the content presentation for your websites? When you write a new blog post or create a new page, are you thinking about
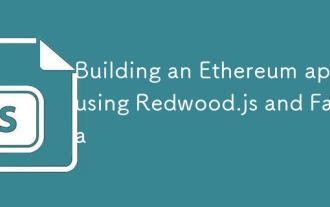
With the recent climb of Bitcoin’s price over 20k $USD, and to it recently breaking 30k, I thought it’s worth taking a deep dive back into creating Ethereum
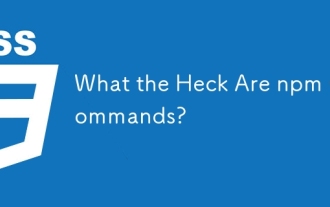
npm commands run various tasks for you, either as a one-off or a continuously running process for things like starting a server or compiling code.
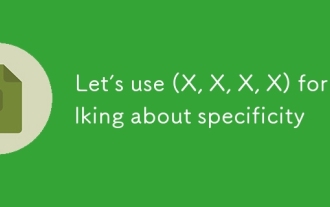
I was just chatting with Eric Meyer the other day and I remembered an Eric Meyer story from my formative years. I wrote a blog post about CSS specificity, and
