Writing Better JavaScript with Flow
Flow: Static type checker to improve the quality of JavaScript code
Flow, launched by Facebook, is a static type checker for JavaScript, designed to efficiently detect type errors in JavaScript code, usually without modifying the code, thereby minimizing programmers' workload.
Core advantages:
-
Optional type checking: You can selectively check specific files, just add a
/*@flow*/
comment on the top of the file to enable Flow's type checking. - Type inference and annotation: Flow supports explicitly specifying types through annotations, and also supports type inference through code context, which is flexible and efficient.
-
Strict null value processing: Flow will not ignore the
null
value, effectively preventing application crashes caused bynull
values, which is different from other types of systems. - Library definition support: Library definition files (libdefs), Flow can avoid type errors when using third-party libraries. The libdef file contains a function or method declaration provided by third-party code.
The nemesis of common JavaScript errors
Have you ever spent a lot of time tracking bugs in your code and eventually found that the error was just simple and avoidable? For example, the function parameter order is wrong, or the wrong data type is passed? The weak type system and implicit type conversion characteristics of JavaScript often lead to errors that do not exist in statically typed languages. Flow is a powerful tool to solve such problems.
March 30, 2017: The article has been updated to reflect changes to the Flow library.
Installation and configuration
Flow supports Mac OS X, Linux (64-bit) and Windows (64-bit). The easiest way to install it is through npm:
npm install --save-dev flow-bin
and add in the package.json
section of the project scripts
file:
"scripts": { "flow": "flow" }
Next, create a .flowconfig
file in the project root directory (you can use npm run flow init
to create an empty configuration file).
Run the Flow check
You can use the npm run flow check
command to check the project code, but this is not the most efficient way, as it rechecks the entire project every time. It is recommended to use a Flow server, which will incrementally check the code and only check for changed parts. Start the server: npm run flow
; stop the server: npm run flow stop
.
Optional type checking and type inference
Flow type checking is optional. You can select the file you want to check and add a /*@flow*/
comment at the top of the file.
Flow mainly performs type checking in two ways:
- Type Annotation: Explicitly specify the expected type in the code.
- Type inference: Flow infers the type based on the context of the use of the variable.
Type inference reduces the workload of code modification, while type annotations provide more precise type checking.
Null value processing and type annotation
Flow is different from other type systems, and it does not ignore the null
value, which helps prevent errors caused by the null
value.
type annotation uses the colon :
prefix, which can be used for function parameters, return values, and variable declarations.
Example type annotation for functions, arrays, classes, and object literals:
-
Function:
function add(x : number, y : number) : number { ... }
-
Array:
var foo : Array<number> = [1, 2, 3];</number>
-
Category:
class Bar { x: string; y: string | number; ... }
-
Object literal:
var obj : { a: string, b: number, ... } = { ... };
-
Nullable type:
var foo : ?string = null;
Library definition (libdefs)
To avoid type errors when using third-party libraries, you can use library definition files (libdefs). You can use the flow-typed
tool to install predefined libdefs, or create custom libdefs yourself.
Remove type annotations
Use the flow-remove-types
tool to remove type annotations from the code to run in the browser.
Summary
Flow is a powerful static type checker that helps you write more robust and easier to maintain JavaScript code. Its optional type checking, type inference and strict null value processing make it an effective tool to improve the quality of JavaScript code.
FAQ
Here are some FAQs about writing better JavaScript code using Flow:
-
What is Flow and why should I use it? Flow is a JavaScript static type checker developed by Facebook, which helps to detect errors before code runs, improving code robustness and maintainability.
-
How to install and set Flow in JavaScript project? Install Flow globally with
npm install -g flow-bin
, then initialize the project withflow init
and add/*@flow*/
comments to the file that requires type checking. -
How to check types in JavaScript code using Flow? Type checking by adding type annotations to variables, function parameters, and return values, and then run the
flow
command. -
The advantages of other types of checkers such as Flow and TypeScript? Flow's type system is more flexible and integrates better with existing JavaScript code, but TypeScript's tools and community support are more complete.
-
How to handle
null
andundefined
values in Flow? Flow does not allow values that may benull
orundefined
when not checked. You can useif (value != null)
for checking. -
Can Flow be used with React and other JavaScript libraries? Yes, Flow has built-in support for React and can support other libraries via
flow-typed
or custom libdefs. -
How to migrate an existing JavaScript project to Flow? You can gradually migrate, first enable Flow in some files, and then gradually add type annotations.
I hope the above information will be helpful to you!
The above is the detailed content of Writing Better JavaScript with Flow. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
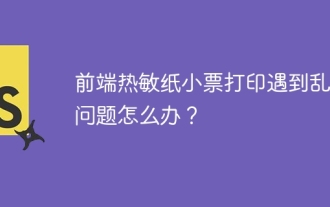
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
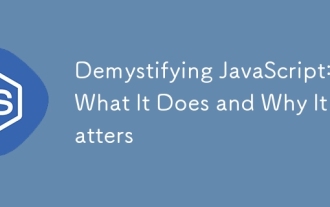
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
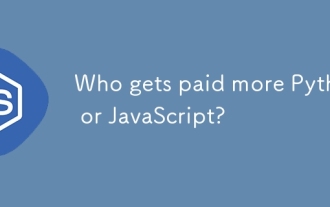
There is no absolute salary for Python and JavaScript developers, depending on skills and industry needs. 1. Python may be paid more in data science and machine learning. 2. JavaScript has great demand in front-end and full-stack development, and its salary is also considerable. 3. Influencing factors include experience, geographical location, company size and specific skills.
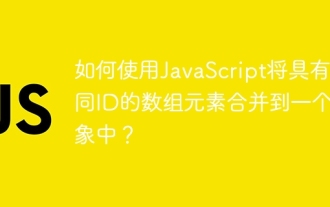
How to merge array elements with the same ID into one object in JavaScript? When processing data, we often encounter the need to have the same ID...
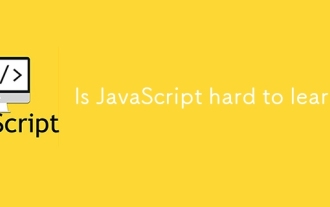
Learning JavaScript is not difficult, but it is challenging. 1) Understand basic concepts such as variables, data types, functions, etc. 2) Master asynchronous programming and implement it through event loops. 3) Use DOM operations and Promise to handle asynchronous requests. 4) Avoid common mistakes and use debugging techniques. 5) Optimize performance and follow best practices.
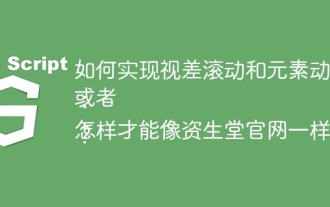
Discussion on the realization of parallax scrolling and element animation effects in this article will explore how to achieve similar to Shiseido official website (https://www.shiseido.co.jp/sb/wonderland/)...
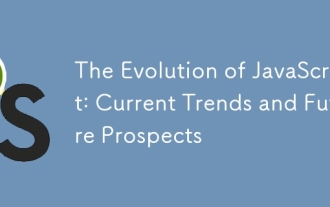
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
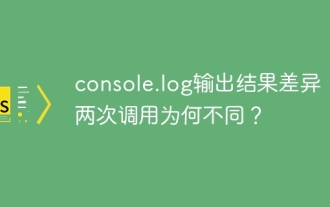
In-depth discussion of the root causes of the difference in console.log output. This article will analyze the differences in the output results of console.log function in a piece of code and explain the reasons behind it. �...
