A Deep Dive into Flask Templates
This article provides a comprehensive guide to Flask templating, covering its importance, benefits, and practical applications. We'll explore creating and rendering templates, utilizing template inheritance and layouts, working with variables and control structures, handling forms and user input, employing built-in and custom filters, managing static files and media, and implementing advanced template techniques. Whether you're a beginner or experienced Flask developer, this in-depth exploration will enhance your understanding and skills in building dynamic and visually appealing web interfaces. (Note: A basic understanding of Flask is assumed.)
Why Use Flask Templates?
Flask templates are crucial for well-structured, maintainable, and reusable code. By separating presentation (UI) from application logic, they simplify UI updates without altering backend code. This separation improves collaboration between developers and designers. Key benefits include:
- Code Reusability: Create reusable components (headers, footers, navigation) for consistent UI across multiple pages.
- Improved Readability: Clean separation of HTML and Python code enhances understanding and maintainability.
- Ease of Maintenance: Update logic or templates independently without affecting the other.
- Flexibility: Easily pass data to and from templates for dynamic content generation.
Creating and Rendering Templates
Flask templates reside in a templates
directory within your application's root directory. Flask uses the Jinja2 templating engine, supporting various extensions (.html
, .svg
, .csv
, etc.). We'll focus on .html
.
Example Application Structure:
<code>my_app/ ├── app.py └── templates/ └── index.html</code>
A simple index.html
template:
<!DOCTYPE html> <html> <head> <title>Index</title> </head> <body> <h1 id="Welcome">Welcome</h1> <p>This is the index page.</p> </body> </html>
Rendering with Flask's render_template()
function:
from flask import Flask, render_template app = Flask(__name__) @app.route('/') def index(): return render_template('index.html') if __name__ == '__main__': app.run()
Template Inheritance and Layouts
Jinja2's inheritance allows creating a base template with common elements (header, footer, navigation) and extending it in child templates.
Base Template (base.html
):
<!DOCTYPE html> <html> <head> <title>{% block title %}{% endblock %}</title> </head> <body> <nav></nav> <div class="content"> {% block content %}{% endblock %} </div> </body> </html>
Child Template (home.html
):
{% extends 'base.html' %} {% block title %}Home - My Website{% endblock %} {% block content %} <h1 id="Welcome-to-My-Website">Welcome to My Website</h1> <p>This is the home page content.</p> {% endblock %}
Template Variables and Control Structures
Pass data from Flask to templates using render_template()
's keyword arguments or a context dictionary. Access variables in templates using {{ variable_name }}
.
Passing variables:
return render_template('template.html', name="Alice", age=30)
Using variables in template.html
:
<code>my_app/ ├── app.py └── templates/ └── index.html</code>
Control Structures (if/else, for loops):
<!DOCTYPE html> <html> <head> <title>Index</title> </head> <body> <h1 id="Welcome">Welcome</h1> <p>This is the index page.</p> </body> </html>
Template Context and Global Variables
The template context contains variables available to the template. Flask provides request
, session
, config
, url_for()
, and g
(for global variables). Use g
to share data across requests:
from flask import Flask, render_template app = Flask(__name__) @app.route('/') def index(): return render_template('index.html') if __name__ == '__main__': app.run()
Template Forms and User Input
Use HTML forms or the WTForms library for robust form handling. WTForms provides validation and simplifies form creation.
Built-in and Custom Filters
Jinja2 offers built-in filters (e.g., upper
, lower
, capitalize
). Create custom filters to extend functionality:
<!DOCTYPE html> <html> <head> <title>{% block title %}{% endblock %}</title> </head> <body> <nav></nav> <div class="content"> {% block content %}{% endblock %} </div> </body> </html>
Working with Static Files and Media
Store static files (CSS, JS, images) in a static
directory. Use url_for('static', filename='...')
to generate URLs for these files in templates.
Advanced Template Techniques
-
Template Inclusion (
{% include 'partial.html' %}
): Reuse common components. -
Macros (
{% macro my_macro(arg) %}{% endmacro %}
): Create reusable code blocks within templates. -
Template Testing and Debugging: Use the
{% debug %}
tag (for development) and thorough testing to identify and fix issues.
Conclusion
Mastering Flask templating is key to building robust and maintainable web applications. By effectively utilizing the techniques discussed, you can create dynamic, user-friendly, and visually appealing web interfaces. Remember to consult the Flask and Jinja2 documentation for further details and advanced features.
The above is the detailed content of A Deep Dive into Flask Templates. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


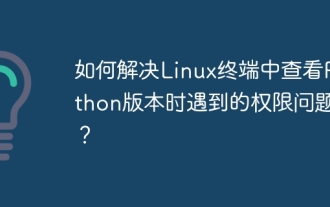
Solution to permission issues when viewing Python version in Linux terminal When you try to view Python version in Linux terminal, enter python...
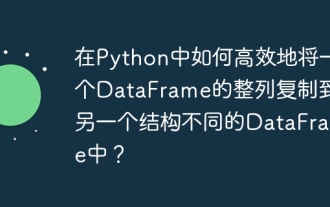
When using Python's pandas library, how to copy whole columns between two DataFrames with different structures is a common problem. Suppose we have two Dats...
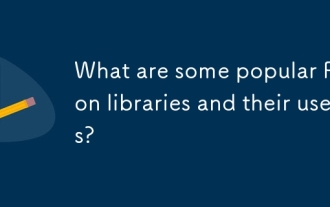
The article discusses popular Python libraries like NumPy, Pandas, Matplotlib, Scikit-learn, TensorFlow, Django, Flask, and Requests, detailing their uses in scientific computing, data analysis, visualization, machine learning, web development, and H
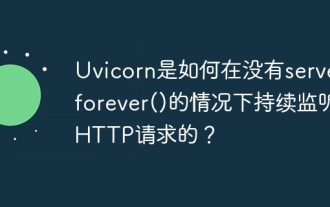
How does Uvicorn continuously listen for HTTP requests? Uvicorn is a lightweight web server based on ASGI. One of its core functions is to listen for HTTP requests and proceed...
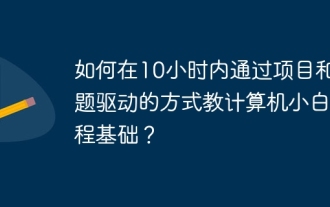
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
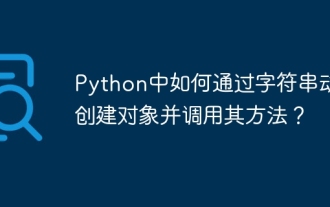
In Python, how to dynamically create an object through a string and call its methods? This is a common programming requirement, especially if it needs to be configured or run...
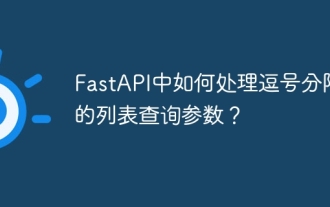
Fastapi ...
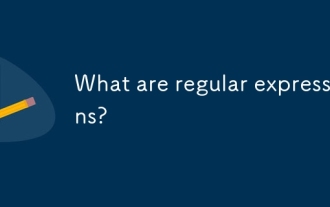
Regular expressions are powerful tools for pattern matching and text manipulation in programming, enhancing efficiency in text processing across various applications.
