Build a React App with User Authentication in 15 Minutes
This article demonstrates integrating Okta's Sign-In Widget into a React application for user authentication. The widget, a Javascript tool, simplifies the process by leveraging Okta's infrastructure.
The tutorial starts with a basic React project, adding authentication via the Okta Sign-In Widget (installed using npm). It covers creating a LoginPage
component, configuring an OpenID Connect application in Okta, and integrating the widget.
The process includes rendering the widget, verifying user login status, and adding a login link to the navigation bar. The final LoginPage
component manages login, logout, and conditional rendering based on login status.
Setting Up the Project:
-
Clone the Seed Project: Begin by cloning a simple React seed project using Git:
git clone https://github.com/leebrandt/simple-react-seed.git okta-react-widget-sample cd okta-react-widget-sample
Copy after login -
Install the Widget: Install the Okta Sign-In Widget via npm:
npm install @okta/okta-signin-widget@2.3.0 --save
Copy after login -
Add Styles: Include the widget's CSS from the Okta CDN within your
index.html
file's<head>
section:<link href="https://ok1static.oktacdn.com/assets/js/sdk/okta-signin-widget/2.3.0/css/okta-sign-in.min.css" type="text/css" rel="stylesheet"/> <link href="https://ok1static.oktacdn.com/assets/js/sdk/okta-signin-widget/2.3.0/css/okta-theme.css" type="text/css" rel="stylesheet"/>
Copy after login
Creating the LoginPage Component:
-
Create the Component: Create a
LoginPage.js
file within./src/components/auth/
. Initially, it's a simple component:import React from 'react'; export default class LoginPage extends React.Component { render() { return <div>Login Page</div>; } }
Copy after login -
Add Routing: Import
LoginPage
into./src/app.js
and add a route:// ... other imports ... import LoginPage from './components/auth/LoginPage'; // ... within the main route ... <Route component={LoginPage} path="/login" />
Copy after login -
Okta Application Setup: Create an OpenID Connect application in the Okta developer console. Set the redirect URI to
http://localhost:3000
.
Integrating the Widget:
-
Widget Initialization: In
LoginPage.js
, initialize the OktaSignIn widget with your Okta organization URL and Client ID:import React from 'react'; import OktaSignIn from '@okta/okta-signin-widget'; export default class LoginPage extends React.Component { // ... (constructor, componentDidMount, showLogin, logout methods as described in the original article) ... }
Copy after login -
Rendering the Widget: Use
this.widget.renderEl
to render the widget within a designated div. Implement methods to check login status (componentDidMount
), show the login widget (showLogin
), and handle logout (logout
). The completeLoginPage.js
component (with error handling and login/logout functionality) is provided in the original article. -
Add Login Link: Add a login link to your navigation bar (e.g.,
./src/components/common/Navigation.js
). -
Testing: Run
npm install
andnpm start
. The application should now display the Okta Sign-In Widget.
The complete, functional code and further details on handling various authentication scenarios (social login, password reset, etc.) are available in the original article and its associated GitHub repository. Remember to replace placeholders like {oktaOrgUrl}
and {clientId}
with your actual Okta configuration values.
The above is the detailed content of Build a React App with User Authentication in 15 Minutes. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










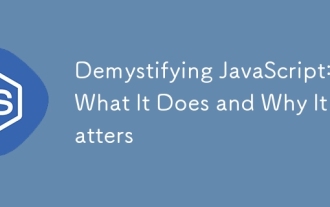
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
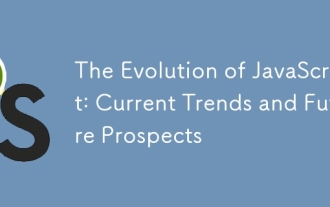
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
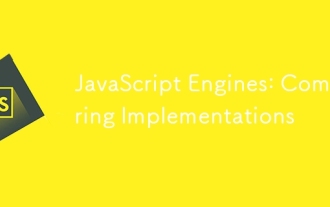
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
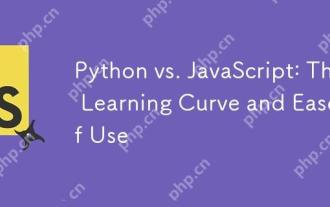
Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
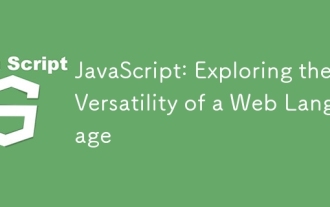
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
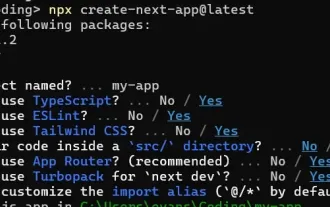
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
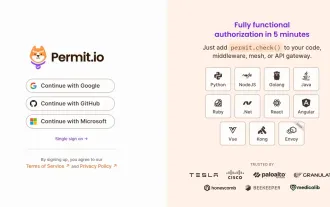
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
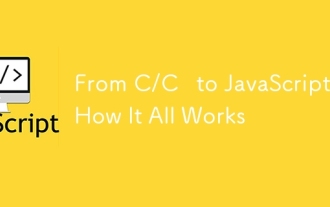
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
