Re-introducing PDO: the Right Way to Access Databases
PHP Data Objects (PDO): A Comprehensive Guide to Database Interaction
This article provides a thorough guide to using PHP Data Objects (PDO) for database interaction. PDO offers an object-oriented approach, enhanced security, and improved portability compared to older methods like mysql
and mysqli
.
Key Advantages of PDO:
- Object-Oriented Design: PDO's object-oriented nature promotes code reusability and maintainability, aligning with modern PHP development practices.
- Database Abstraction: Easily switch between different database systems (MySQL, PostgreSQL, SQLite, etc.) with minimal code changes.
- Enhanced Security: Parameter binding prevents SQL injection vulnerabilities, a critical security concern.
- Flexible Data Fetching: Retrieve data as arrays, objects, or custom classes, simplifying data manipulation.
- Prepared Statements: Optimize query performance and security, especially for repeated queries.
- Future-Proofing: PDO ensures compatibility with the latest PHP versions, unlike deprecated extensions.
Why Choose PDO over mysql
and mysqli
?
Migrating to PDO offers significant benefits:
- Object-Oriented Programming: PDO encourages object-oriented coding, leading to better code structure, testability, and reusability.
- Database Portability: Easily adapt your application to different database systems without major code revisions.
- SQL Injection Prevention: Parameter binding eliminates the risk of SQL injection attacks.
- Object-Oriented Data Handling: Fetch data directly into objects or custom classes for streamlined data access.
-
Deprecated Extensions: The
mysql
extension is obsolete;mysqli
is an improvement, but PDO provides even more advantages.
Verifying and Installing PDO:
Check if PDO is installed using the command php -i | grep 'pdo'
in your terminal or by examining the output of phpinfo()
.
Installation instructions vary depending on your operating system and PHP installation method. Common package managers (like yum
, apt
, etc.) simplify installation. For Windows users, check your WAMP or XAMPP configuration or the PHP installation directory to enable the php_pdo_mysql.dll
extension.
Working with PDO: A Step-by-Step Overview
-
Connecting to the Database: Instantiate a PDO object with the Data Source Name (DSN), username, and password. For MySQL:
$pdo = new PDO('mysql:host=localhost;dbname=mydb;charset=utf8', 'username', 'password');
-
Executing Queries: Use
exec()
for queries that don't return result sets (INSERT, UPDATE, DELETE).$pdo->exec("INSERT INTO users (name) VALUES ('John')");
-
Fetching Query Results: Use
query()
for queries returning result sets (SELECT). Iterate through results usingfetch()
with various fetch modes (e.g.,PDO::FETCH_ASSOC
,PDO::FETCH_OBJ
,PDO::FETCH_CLASS
).$stmt = $pdo->query('SELECT * FROM users'); while ($row = $stmt->fetch(PDO::FETCH_ASSOC)) { echo $row['name'] . "<br>"; }
Copy after login -
Prepared Statements and Parameter Binding: Prepare statements using
prepare()
and execute them withexecute()
, binding parameters to prevent SQL injection.$stmt = $pdo->prepare('SELECT * FROM users WHERE id = :id'); $stmt->execute([':id' => 1]); $user = $stmt->fetch(PDO::FETCH_ASSOC);
Copy after login -
Handling
IN
Clauses: Construct theIN
clause string manually and use placeholders (?
) for parameter binding. -
Specifying Data Types: Use
bindValue()
to specify parameter data types for better readability, maintainability, and performance.
Error Handling: Set the error mode to exceptions using setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION)
to catch and handle database errors gracefully.
Conclusion:
PDO offers a significant upgrade for database interaction in PHP, providing a robust, secure, and portable solution. Its object-oriented design and features like prepared statements make it an essential tool for modern PHP development. The initial effort of migrating is well worth the long-term benefits.
Frequently Asked Questions (FAQs):
The FAQs section from the original input has been incorporated into the above text for a more comprehensive and integrated guide.
The above is the detailed content of Re-introducing PDO: the Right Way to Access Databases. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










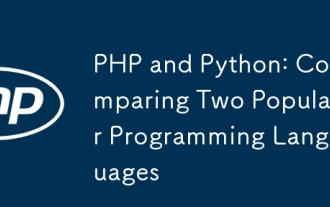
PHP and Python each have their own advantages, and choose according to project requirements. 1.PHP is suitable for web development, especially for rapid development and maintenance of websites. 2. Python is suitable for data science, machine learning and artificial intelligence, with concise syntax and suitable for beginners.
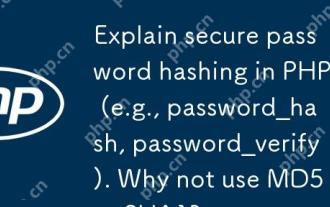
In PHP, password_hash and password_verify functions should be used to implement secure password hashing, and MD5 or SHA1 should not be used. 1) password_hash generates a hash containing salt values to enhance security. 2) Password_verify verify password and ensure security by comparing hash values. 3) MD5 and SHA1 are vulnerable and lack salt values, and are not suitable for modern password security.
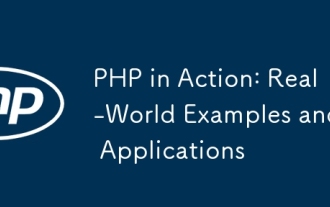
PHP is widely used in e-commerce, content management systems and API development. 1) E-commerce: used for shopping cart function and payment processing. 2) Content management system: used for dynamic content generation and user management. 3) API development: used for RESTful API development and API security. Through performance optimization and best practices, the efficiency and maintainability of PHP applications are improved.
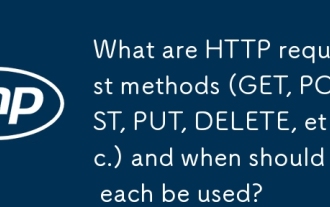
HTTP request methods include GET, POST, PUT and DELETE, which are used to obtain, submit, update and delete resources respectively. 1. The GET method is used to obtain resources and is suitable for read operations. 2. The POST method is used to submit data and is often used to create new resources. 3. The PUT method is used to update resources and is suitable for complete updates. 4. The DELETE method is used to delete resources and is suitable for deletion operations.
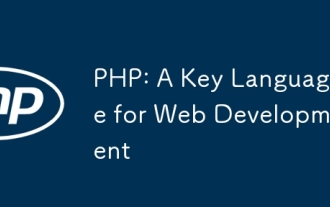
PHP is a scripting language widely used on the server side, especially suitable for web development. 1.PHP can embed HTML, process HTTP requests and responses, and supports a variety of databases. 2.PHP is used to generate dynamic web content, process form data, access databases, etc., with strong community support and open source resources. 3. PHP is an interpreted language, and the execution process includes lexical analysis, grammatical analysis, compilation and execution. 4.PHP can be combined with MySQL for advanced applications such as user registration systems. 5. When debugging PHP, you can use functions such as error_reporting() and var_dump(). 6. Optimize PHP code to use caching mechanisms, optimize database queries and use built-in functions. 7
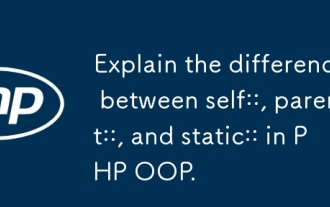
In PHPOOP, self:: refers to the current class, parent:: refers to the parent class, static:: is used for late static binding. 1.self:: is used for static method and constant calls, but does not support late static binding. 2.parent:: is used for subclasses to call parent class methods, and private methods cannot be accessed. 3.static:: supports late static binding, suitable for inheritance and polymorphism, but may affect the readability of the code.
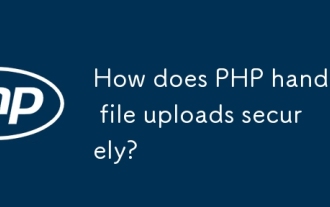
PHP handles file uploads through the $\_FILES variable. The methods to ensure security include: 1. Check upload errors, 2. Verify file type and size, 3. Prevent file overwriting, 4. Move files to a permanent storage location.
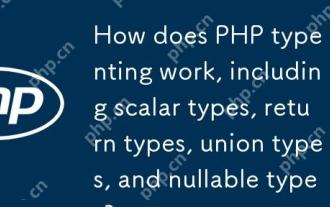
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.
