Build a Search Engine with Node.js and Elasticsearch
This article was peer-reviewed by Mark Brown, Vildan Softic and Moritz Kröger. Thanks to all the peer reviewers at SitePoint for making SitePoint’s content perfect!
Elasticsearch is an open source search engine that is becoming increasingly popular due to its high performance and distributed architecture. This article will explore its key features and guide you how to use it to create a Node.js search engine.
Key Points
- Elasticsearch is a high-performance distributed search engine built on Apache Lucene, mainly used for real-time indexing and searching data.
- The system is pattern-free, can automatically detect data structures and types, and supports a large number of operations by using JSON's RESTful API on HTTP.
- Elasticsearch can be easily installed on the main operating system using a package manager such as ZIP files or Homebrew, and requires a Java runtime environment to run.
- Node.js' official Elasticsearch module facilitates the integration of Elasticsearch's functionality with Node.js applications, allowing efficient data indexing and querying.
- The key concepts of Elasticsearch include indexing, types and searches, which enable complex queries, filters and aggregations to refine and analyze data.
- Elasticsearch supports real-time search, which means that the newly indexed data can be searched almost immediately, thereby improving the response speed of applications that rely on the latest information.
- This tutorial provides practical examples and code snippets about setting up Elasticsearch with Node.js, performing various types of searches, and using advanced features such as data aggregation and suggestions.
Introduction to Elasticsearch
Elasticsearch is built on top of Apache Lucene, a high-performance text search engine library. Although Elasticsearch can perform data storage and retrieval, its main purpose is not to act as a database, but a search engine (server), whose main goal is to index, search and provide real-time statistics of data.
Elasticsearch has a distributed architecture that can achieve horizontal scaling by adding more nodes and leveraging additional hardware. It supports thousands of nodes to process petabytes of data. Its horizontal scaling also means it has high availability and can rebalance the data if any node fails.
After importing the data, it will be used immediately for searching. Elasticsearch is schema-free, stores data in JSON documents and can automatically detect data structures and types.
Elasticsearch is also completely API-powered. This means that almost all operations can be done through a simple RESTful API using JSON data on HTTP. It provides many client libraries for almost any programming language, including Node.js. In this tutorial, we will use the official client library.
Elasticsearch is very flexible in terms of hardware and software requirements. While the recommended production environment is 64GB of RAM and as many CPU cores as possible, you can still run it on a resource-constrained system and get decent performance (assuming your dataset isn't very large). To follow the examples in this article, a system with 2GB of memory and a single CPU core is enough.
You can run Elasticsearch on all major operating systems (Linux, Mac OS, and Windows). To do this, you need to install the latest version of the Java runtime environment (see the "Installing Elasticsearch" section). To follow the examples in this article, you also need to install Node.js (any version after v0.11.0 will do), as well as npm.
Elasticsearch terminology
Elasticsearch uses its own terminology, which is different in some cases from a typical database system. Here is a list of commonly used terms and their meanings in Elasticsearch.
Index: This term has two meanings in the context of Elasticsearch. The first is the operation of adding data. When data is added, the text is broken down into tags (such as words), and each tag is indexed. However, indexes also refer to the location where all index data is stored. Basically, when you import the data, it gets indexed into an index. Every time you want to perform anything on your data, you need to specify its index name.
Type: Elasticsearch provides a more detailed classification of documents in the index, called types. Each document in the index should also have a type. For example, we can define a library index and then index multiple types of data (such as articles, books, reports, and presentations) into it. Since indexes have almost fixed overhead, it is recommended to use fewer indexes and more types instead of more indexes and fewer types.
Search: This term may mean the same as you think. You can search for data in different indexes and types. Elasticsearch provides many types of search queries, such as terms, phrases, ranges, fuzzy, and even geographic data queries.
Filter: Elasticsearch allows you to filter search results based on different criteria to further narrow the range of results. If you add a new search query to a set of documents, it may change the order according to the dependency, but if you add the same query as a filter, the order remains the same.
Aggregation: These provide different types of statistics for aggregated data, such as minimum, maximum, average, sum, histogram, and more.
Suggestions: Elasticsearch provides different types of suggestions for input text. These suggestions can be based on terms or phrases, and even suggestions can be completed.
Install Elasticsearch
Elasticsearch is available under the Apache 2 license; it can be downloaded, used and modified for free. Before installing it, you need to make sure that the Java Runtime Environment (JRE) is installed on your computer. Elasticsearch is written in Java and depends on Java libraries to run. To check if Java is installed on your system, you can type the following in the command line.
1 |
|
The latest stable version of Java is recommended (1.8 at the time of writing). You can find a guide on installing Java on your system here.
Next, to download the latest version of Elasticsearch (2.4.0 at the time of writing), visit the download page and download the ZIP file. Elasticsearch does not require installation, and a single zip file contains a complete set of files that run programs on all supported operating systems. Unzip the downloaded file and it's done! There are several other ways to run Elasticsearch, such as getting TAR files or packages for different Linux distributions (see here).
If you are running Mac OS X and have Homebrew installed, you can use brew install elasticsearch to install Elasticsearch. Homebrew will automatically add the executable to your path and install the required services. It also helps you update your application with a single command: brew upgrade elasticsearch.
To run Elasticsearch on Windows, run binelasticsearch.bat from the decompressed directory. For all other operating systems, run ./bin/elasticsearch from the terminal. At this point it should run on your system.
As I mentioned earlier, almost everything you can do with Elasticsearch can be done via the REST API. Elasticsearch uses port 9200 by default. To make sure you run it correctly, visit http://localhost:9200/ in your browser, which should show some basic information about the instance you are running.
For more information on installation and troubleshooting, you can access the documentation.
Graphic User Interface
Elasticsearch provides almost all features through the REST API and does not come with a graphical user interface (GUI). While I've covered how to do all the necessary operations through the API and Node.js, there are some GUI tools that provide visual information about indexes and data, and even some advanced analytics.
Kibana, developed by the same company, provides a real-time summary of the data, as well as some custom visualization and analysis options. Kibana is free and has detailed documentation.
The community has also developed other tools, including elasticsearch-head, the Elasticsearch GUI, and even a Chrome extension called ElasticSearch Toolbox. These tools can help you browse indexes and data in your browser, and even try different searches and aggregate queries. All of these tools provide walkthroughs for installation and use.
Set Node.js environment
Elasticsearch provides an official module for Node.js called elasticsearch. First, you need to add the module to your project folder and save the dependencies for future use.
1 |
|
You can then import the module in the script as follows:
1 |
|
Finally, you need to set up a client that handles communication with Elasticsearch. In this example, I assume you are running Elasticsearch on your local machine with an IP address of 127.0.0.1 and a port of 9200 (default setting).
1 |
|
log option ensures that all errors are logged. For the rest of this article, I will use the same esClient object to communicate with Elasticsearch. The complete documentation of the node module is provided here.
Note: All source code for this tutorial is available on GitHub. The easiest way to follow is to clone the repository to your PC and run the example from there:
1 |
|
Import data
In this tutorial, I will use a dataset of academic articles with randomly generated content. The data is provided in JSON format, and there are 1,000 articles in the dataset. To display the style of the data, an item in the dataset is shown below.
1 2 3 4 |
|
The field name is self-explanatory. The only thing to note is that the body field is not shown here, as it contains a complete randomly generated document (including 100 to 200 paragraphs). You can find the complete dataset here.
While Elasticsearch provides methods for indexing, updating, and deleting individual data points, we will use Elasticserch's batch method to import data, which is used to perform operations on large datasets more efficiently:
1 2 3 |
|
Here, we call the bulkIndex function, passing the library as the index name, article as the type, and the JSON data we want to index. The bulkIndex function calls the bulk method on the esClient object in turn. This method takes an object with the body attribute as a parameter. The value provided to the body property is an array with two entries per operation. In the first entry, the type of the operation is specified as a JSON object. In this object, the index property determines the operation to be performed (in this case the index document), as well as the index name, type name, and document ID. The next entry corresponds to the document itself.
Please note that in the future you can add other types of documents (such as books or reports) to the same index in this way. We can also assign a unique ID to each document, but this is optional – if you don't provide one, Elasticsearch will assign you a unique randomly generated ID to each document.
Assuming you have cloned the repository, you can now import the data into Elasticsearch by executing the following command from the project root:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 |
|
Check whether the data is indexed correctly
A major feature of Elasticsearch is its near-real-time search. This means that once the documents are indexed, they are available for searching within a second (see here). Once the data is indexed, you can check the index information by running indices.js (linked to the source code):
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 |
|
The method in the client's cat object provides different information about the currently running instance. The indices method lists all indexes, their health, the number of documents, and their size on disk. The v option adds a title to the cat method's response.
When you run the above code snippet, you will notice that it outputs a color code to indicate the health of the cluster. Red indicates that there is a problem with the cluster and it is not running. Yellow indicates the cluster is running, but there is a warning, and green indicates everything is fine. Most likely (depending on your settings) you get a yellow state when running on your local machine. This is because the default settings contain five nodes of the cluster, but only one instance is running on your local machine. While you should always strive for a green state in production, for the purposes of this tutorial, you can continue to use Elasticsearch in the yellow state.
1 |
|
Dynamic and custom mapping
As I mentioned earlier, Elasticsearch is patternless. This means that you don't have to define the structure of the data before importing it (similar to defining a table in a SQL database), but Elasticsearch will automatically detect it for you. However, despite being called schemaless, data structures have some limitations.
Elasticsearch calls the structure of the data a mapping. If no mapping exists, Elasticsearch looks at each field of the JSON data when indexing the data and automatically defines the mapping based on its type. If the field already has a mapping entry, it ensures that the new data added follows the same format. Otherwise, it will throw an error.
For example, if {"key1": 12} has been indexed, Elasticsearch will automatically map field key1 to long. Now, if you try to index {"key1": "value1", "key2": "value2"}, it throws an error because it expects the type of field key1 to be long. Meanwhile, the object {"key1": 13, "key2": "value2"} will be indexed without problems and key2 of type string will be added to the map.
Map is beyond the scope of this article, and in most cases, automatic mapping works well. I recommend checking out the elasticsearch documentation, which provides an in-depth discussion of mappings.
Build a search engine
Once the data is indexed, we can implement search engines. Elasticsearch provides an intuitive full-text search query structure called Query DSL—it is based on JSON—to define queries. There are many types of search queries available, but in this article we will look at several of the more common queries. The complete documentation for Query DSL can be found here.
Remember, I have provided a code link for each example shown here. Once the environment is set up and the test data is indexed, you can clone the repository and run any examples on your machine. To do this, just run node filename.js from the command line.
Return all documents in one or more indexes
To perform a search, we will use various search methods provided by the client. The easiest query is match_all, which returns all documents in one or more indexes. The following example shows how we get all stored documents in the index (link to source code).
1 |
|
The main search query is contained in the query object. As we will see later, we can add different types of search queries to this object. For each query, we add a key that contains the query type (match_all in this example) with the value of the object containing the search options. There is no option in this example because we want to return all documents in the index.
In addition to the query object, the search body can also contain other optional properties, including size and from. The size attribute determines the number of documents to be included in the response. If this value does not exist, ten documents are returned by default. The from property determines the starting index of the returned document. This is useful for pagination.
Understand the search API response
If you are recording the response of the search API (results in the above example), it may initially look complicated because it contains a lot of information.
1 |
|
At the highest level, the response includes a take attribute that represents the number of milliseconds it took to find the result, timed_out, which is true only if the result is not found within the maximum allowed time, _shards, which is used to provide relevant nodes Information about status (if deployed as a node cluster), and hits, which contain search results.
In the hits property, we have an object with the following properties:
- total—Indicates the total number of matches
- max_score—The highest score for the item found
- hits - an array containing the items found. In each document in the hits array, we have the index, type, document ID, score, and the document itself (in the _source element).
It's very complex, but the good news is that once you implement a way to extract the results, you will always get the results in the same format no matter what your search query is.
Also note that one of the advantages of Elasticsearch is that it automatically assigns a score to each matching document. This score is used to quantify the relevance of the document, and by default, the results are returned in order of decreasing scores. In the case where we use match_all to retrieve all documents, the scores make no sense and all scores are calculated as 1.0.
The document with a specific value in the match field
Now, let's look at some more interesting examples. To match documents that contain specific values in the field, we can use match query. Below is a simple search body with a match query (link to source code).
1 |
|
As I mentioned earlier, we first add an entry to a query object that contains the search type, match in this example. Within the search type object, we identify the document field to search, here is the title. In it, we put data related to the search, including the query attribute. I hope that after testing the above example, you will start to be amazed at the search speed.
The above search query returns a document whose title field matches any word in the query attribute. We can set the minimum number of matches as shown below.
1 |
|
This query matches documents with at least three specified words in its title. If there are fewer than three words in the query, all words must appear in the title to match the document. Another useful feature added to search queries is ambiguity. This is useful if the user enters an error while writing a query, as fuzzy matches will look for terms with similar spellings. For strings, the ambiguity value is based on the maximum Levinstein distance allowed for each term. Here is an example with ambiguity.
1 |
|
Search in multiple fields
If you want to search in multiple fields, you can use multi_match to search for types. It's similar to match, except that instead of using the field as a key in the search query object, we add a field key, which is an array of fields to search for. Here we search in the title, authors.firstname and authors.lastname fields. (Link to source code)
1 |
|
multi_match query supports other search properties such as minimum_should_match and fuzziness. Elasticsearch supports wildcards (such as *) to match multiple fields, so we can shorten the above example to ['title', 'authors.*name'].
Match the complete phrase
Elasticsearch can also match entered phrases exactly without matching at the term level. This query is an extension of a regular match query called match_phrase. Here is an example of match_phrase. (Link to source code)
1 2 3 4 |
|
Combining multiple queries
So far, in the example, we have used only one query per request. However, Elasticsearch allows you to combine multiple queries. The most common composite query is bool. The bool query accepts four types of keys: must, should, must_not, and filter. As the name implies, the documents in the result must match the query in must, cannot match the query in must_not, if they match the query in should, you will get a higher score. Each of the above elements can receive multiple queries in the form of a query array. Below, we use bool query with a new query type query_string. This allows you to write more advanced queries using keywords like AND and OR. The complete documentation of query_string syntax can be found here. Additionally, we use a scope query (documented here), which allows us to limit fields to a given range. (Link to source code)
In the above example, the query returns the name of the author that contains term1
or1 2 3 |
|
and Their title contains term3, and they were not in 2011, 2012 Or documents published in 2013. Additionally, documents containing a given phrase in their body will get higher scores and will be displayed at the top of the result (because the match query is in the should clause). Filters, Aggregations and Recommendations
In addition to its advanced search capabilities, Elasticsearch offers additional features. Here, we look at three more common features.
Filter
Usually, you may want to refine your search results based on specific criteria. Elasticsearch provides this functionality through filters. In our article data, let's say your search returns several articles from which you want to select only those published in five specific years. You can simply filter out anything that doesn't match your criteria from your search results without changing the search order.
The difference between a filter and the same query in the must clause of a bool query is that the filter does not affect the search score, and the must query will affect. When search results are returned and users filter based on certain criteria, they do not want to change the original result order, but rather just want to delete irrelevant documents from the result. Filters follow the same format as searches, but more commonly, they are defined on fields with definite values rather than text strings. Elasticsearch recommends adding filters through the filter clause of the bool composite search query.Continue with the example above, suppose we want to limit our search results to articles published from 2011 to 2015. To do this, we just need to add a range query to the filter part of the original search query. This will remove any mismatched documents from the result. Here is an example of filtering queries. (Link to source code)
1 |
|
Polymerization
The aggregation framework provides various aggregated data and statistics based on search queries. The two main types of aggregation are metrics and grouping, where metric aggregation tracks and computes metrics on a set of documents, while grouped aggregation builds buckets, each associated with a key and a document condition. Examples of metric aggregation include mean, minimum, maximum, sum and value count. Examples of grouped aggregation include ranges, date ranges, histograms, and terminology. An in-depth explanation of the aggregator can be found here.Aggregations are placed inside the aggregates object, which itself is placed directly in the body of the search object. In the aggregates object, each key is the name assigned by the user to the aggregator. The aggregator type and options should be placed as the value of that key. Next, we look at two different aggregators, a metric aggregator and a bucket aggregator. As a metric aggregator, we try to find the minimum year value (oldest post) in the dataset, and for the bucket aggregator, we try to find the number of occurrences of each keyword. (Link to source code)
1 |
|
1 |
|
Suggestions
Elasticsearch has multiple types of suggestioners that can provide replacement or completion suggestions for input terms (documented here). We will check out the term and phrase suggestion here. The term suggester provides advice for each term in the input text (if any), while the phrase suggester treats the input text as a complete phrase (rather than breaking it down into terms) and provides other phrase suggestions (if any) . To use the suggestion API, we need to call the suggest method on the Node.js client. Here is an example of a term suggester. (Link to source code)
1 |
|
In the request body, consistent with all other client methods, we have an index field that determines the index of the search. In the body property, we add the text we are looking for suggestions, and (as with the aggregate object), we assign a name to each suggester (in this case titleSuggester). Its value determines the type and options of the suggestor. In this case, we are using a term suggester for the title field and limiting the maximum number of suggestions per tag to five (size: 5).
The response of the suggest API contains a key for each suggestor you request, which is an array of sizes that are the same as the number of terms in the text field. For each object in this array, there is an options object whose text field contains suggestions. The following is a partial response to the above request.
1 |
|
1 |
|
Further reading
Elasticsearch provides a wide range of features, far beyond the scope of this article. In this article, I try to explain its functionality at a high level and refer to appropriate resources for further learning. Elasticsearch is very reliable and has excellent performance (I hope you have noticed this when running the examples). This, coupled with growing community support, increases Elasticsearch adoption in the industry, especially among companies that process real-time data or big data.After carefully reading the examples provided here, I highly recommend that you check out the documentation. They provide two main sources, one is a reference to Elasticsearch and its features, and the other is a guide that focuses more on implementation, use cases and best practices. You can also find detailed documentation for the Node.js client here.
Are you already using Elasticsearch? What is your experience? Or are you planning to give it a try after reading this? Please let me know in the comments below.
FAQs (FAQ) about search engine Node Elasticsearch
What is the difference between Elasticsearch and other search engines (such as Elasticlunr or Minisearch)?
Elasticsearch is a powerful, distributed and open source search and analysis engine. It is designed to process large amounts of data and provide real-time search results. On the other hand, Elasticlunr and Minisearch are lightweight client search libraries for JavaScript. They are designed for smaller data sets and are often used in browser-based applications. While Elasticsearch offers more advanced features such as distributed search, data analytics, and machine learning capabilities, Elasticlunr and Minisearch are easier to use for basic search capabilities.
How to implement Elasticsearch in Node.js application?
Implementing Elasticsearch in Node.js application involves several steps. First, you need to install the Elasticsearch package using npm. Then you need to create an Elasticsearch client instance and connect it to your Elasticsearch server. After that, you can use the client's methods to perform various actions, such as indexing documents, searching for data, and managing your Elasticsearch cluster.
Can I build a search engine in my browser using Elasticsearch?
While Elasticsearch is mainly designed for server-side applications, it can be used in browser-based applications with the help of Node.js server. The server can act as a proxy between the browser and the Elasticsearch server, handling all search requests and responses. However, for simpler use cases, a client search library like Elasticlunr or Minisearch might be a better choice.
How does Elasticsearch compare to other npm search packages?
Elasticsearch is one of the most popular search packages on npm, thanks to its powerful features and scalability. It provides a comprehensive set of APIs for indexing, searching and analyzing data. However, it is more complex and resource-intensive than other npm search packages. If you are working on a small project, or you need simple search functionality, other npm packages such as search-index or js-search may be more suitable.
How to build a simple in-browser search engine using JavaScript?
Building a simple in-browser search engine using JavaScript includes creating indexes of data, implementing search functions and displaying search results. You can simplify this process using JavaScript search libraries like Elasticlunr or Minisearch. These libraries provide easy-to-use APIs to index and search data and can be used directly in the browser without server requirements.
What are the advantages of using Elasticsearch for search in my app?
Elasticsearch provides many advantages for implementing search capabilities in your application. It provides real-time search results, which means that once the document is indexed, it becomes searchable. It also supports complex search queries, allowing you to search data based on multiple criteria. Additionally, Elasticsearch is highly scalable and can handle large amounts of data without affecting performance.
How does Elasticsearch handle data indexing?
Elasticsearch uses a data structure called inverted indexing for data indexing. This allows it to quickly find documents that match the search query. When a document is indexed, Elasticsearch analyzes the content and creates a list of unique words, and then stores the words in the inverted index along with information about their position in the document.
Can I use Elasticsearch for data analysis?
Yes, Elasticsearch is not only a search engine, but also a powerful data analysis tool. It supports aggregation, allowing you to aggregate and analyze your data in a variety of ways. You can use Elasticsearch to perform complex data analysis tasks such as calculating mean, sum or count, finding minimum or maximum values, grouping data based on specific conditions, and more.
Is Elasticsearch suitable for big data applications?
Yes, Elasticsearch is designed to handle big data applications. It is a distributed system, which means it can scale horizontally by adding more nodes to the cluster. This allows it to process large amounts of data and deliver fast search results even under heavy loads. Additionally, Elasticsearch supports sharding and replication, which further enhances its scalability and reliability.
How to optimize the performance of Elasticsearch applications?
There are several ways to optimize the performance of your Elasticsearch application. First, you should correctly configure the Elasticsearch cluster, including the number of nodes, shards, and replicas. Second, you should optimize the indexing process by using batch indexing, disabling refreshes, and using the correct analyzer. Finally, you should optimize your search queries by using filters instead of queries as much as possible, avoiding heavy aggregations, and using the "explain" API to understand how the query is executed.
The above is the detailed content of Build a Search Engine with Node.js and Elasticsearch. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
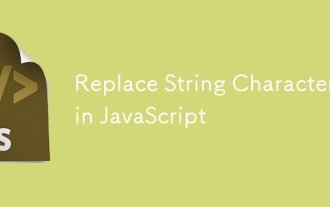
Replace String Characters in JavaScript
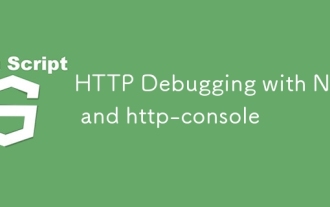
HTTP Debugging with Node and http-console
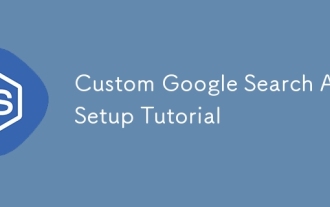
Custom Google Search API Setup Tutorial
