Getting Started with PouchDB Client-Side JavaScript Database
This article has been reviewed by Sebastian Seitz and Taulant Spahiu. Thanks to SitePoint's peer reviewers for their contributions!
Modern client-side web applications demand sophisticated data handling capabilities. Browsers now offer robust JavaScript performance and rich APIs (geolocation, peer-to-peer communication, etc.), fueling the growth of complex web apps. This advancement necessitates effective client-side storage solutions, where JavaScript databases like PouchDB excel.
Key Features of PouchDB
- PouchDB, an open-source JavaScript database inspired by Apache CouchDB, seamlessly operates within the browser, leveraging IndexedDB for persistent data storage across page refreshes.
- Installation is straightforward: integrate a standalone script into your HTML or utilize npm for Node.js projects.
- Supports CRUD operations and bulk document manipulation, offering flexible data management with simple JavaScript commands—no rigid schema required.
- Real-time data synchronization and change tracking are built-in, enabling dynamic UI updates based on immediate database changes.
- Facilitates offline data storage with seamless synchronization to CouchDB, ensuring data consistency across devices and sessions, ideal for robust offline-first applications.
Understanding PouchDB
PouchDB is an open-source JavaScript database modeled after Apache CouchDB, optimized for browser environments.
A JavaScript database, in essence, is a JavaScript object offering methods (API) for data manipulation (put, get, search). A simple JavaScript object serves as the most basic example. If you're familiar with Meteor, Minimongo is a comparable client-side database mimicking the MongoDB API.
PouchDB distinguishes itself from Minimongo by utilizing IndexedDB for persistent storage (not just in-memory). IndexedDB is a low-level API for storing substantial structured data, including files/blobs. This ensures data persistence even after page refreshes (though data is browser-specific). Different adapters allow customization of the underlying storage layer.
PouchDB and CouchDB: The Connection
PouchDB is a JavaScript implementation of CouchDB, closely mirroring its API. For instance, fetching all documents in CouchDB uses /db/_all_docs?include_docs=true
, while in PouchDB, it's db.allDocs({include_docs: true})
.
PouchDB empowers applications to store data locally offline and synchronize it with CouchDB upon reconnection.
Getting Started with PouchDB
Include the PouchDB client library to begin. Use the standalone build (making the PouchDB
constructor globally available) via a
Alternatively, for Node.js/browserify/webpack, use npm:
$ npm install pouchdb --save
Then, in your JavaScript:
var PouchDB = require('pouchdb');
Working with PouchDB: Data Manipulation
Database Creation
PouchDB
Creating a database is as simple as calling the
var movies = new PouchDB('Movies');
.info()
Use the
movies.info().then(function(info) { console.log(info); });
Document Management
PouchDB is NoSQL, document-based; there's no fixed schema. Insert JSON documents directly.
Document Creation
.put()
Use the
movies.put({ _id: 'tdkr', title: 'The Dark Knight Rises', director: 'Christopher Nolan' }) .then(function(response) { console.log("Success", response); }) .catch(function(err) { console.log("Error", err); });
Document Retrieval
.get()
Use the
movies.get('tdkr').then(function(doc) { console.log(doc); }).catch(function(err) { console.log(err); });
Document Updates
.put()
Update documents by fetching, modifying, and using _rev
again, providing the
movies.get('tdkr').then(function(doc) { doc.year = "2012"; return movies.put(doc); }).then(function(res) { console.log(res); });
Document Deletion
.remove()
Use the _deleted: true
method (or set .put()
before
movies.get('tdkr').then(function(doc) { return movies.remove(doc); }).then(function(res) { console.log("Remove operation response", res); });
Database Deletion
.destroy()
Use the
movies.destroy();
Bulk Operations
PouchDB offers efficient bulk operations:
Bulk Inserts
.bulkDocs()
Use
movies.bulkDocs([ { _id: 'easy-a', title: "Easy A" }, { _id: 'black-swan', title: 'Black Swan' } ]);
Bulk Reads
.allDocs()
Use
movies.allDocs({ include_docs: true }).then(function(docs) { console.log(docs); }); ``` This method allows for ordering, filtering, and pagination using `startkey`, `endkey`, and `descending` parameters. Real-time Updates with ChangeFeeds --------------------------------- PouchDB's `changes()` API allows monitoring database changes: ```javascript db.changes({ since: 'now', live: true, include_docs: true }) .on('change', function(change) { // Update UI based on change.id and change.doc }) .on('error', function(err) { // Handle errors });
Synchronization with CouchDB
Sync local PouchDB data with a remote CouchDB instance:
var localDB = new PouchDB('mylocaldb'); var remoteDB = new PouchDB('http://localhost:5984/myremotedb'); localDB.sync(remoteDB, { live: true }); // For live, bidirectional syncing
Further Exploration
Explore PouchDB plugins and framework adaptors. The PouchDB Inspector Chrome extension provides a helpful GUI for database inspection.
Frequently Asked Questions
This section provides concise answers to common PouchDB questions, covering installation, database creation, document manipulation, error handling, and synchronization with CouchDB. Refer to the original article for detailed explanations and code examples.<script> tag: <pre class="brush:php;toolbar:false"><code class="html"><script src="https://cdn.jsdelivr.net/pouchdb/5.4.5/pouchdb.min.js"></script>
The above is the detailed content of Getting Started with PouchDB Client-Side JavaScript Database. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


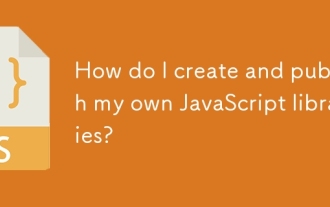
Article discusses creating, publishing, and maintaining JavaScript libraries, focusing on planning, development, testing, documentation, and promotion strategies.
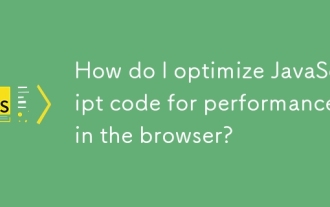
The article discusses strategies for optimizing JavaScript performance in browsers, focusing on reducing execution time and minimizing impact on page load speed.
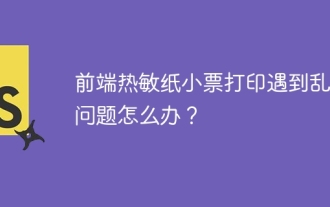
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
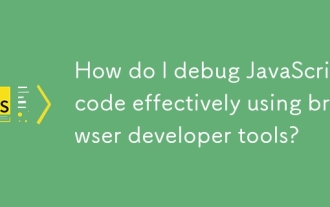
The article discusses effective JavaScript debugging using browser developer tools, focusing on setting breakpoints, using the console, and analyzing performance.
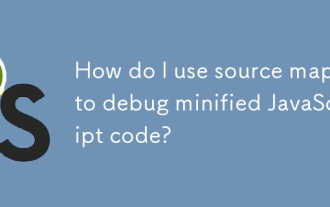
The article explains how to use source maps to debug minified JavaScript by mapping it back to the original code. It discusses enabling source maps, setting breakpoints, and using tools like Chrome DevTools and Webpack.
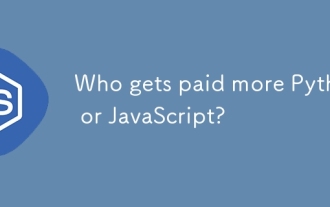
There is no absolute salary for Python and JavaScript developers, depending on skills and industry needs. 1. Python may be paid more in data science and machine learning. 2. JavaScript has great demand in front-end and full-stack development, and its salary is also considerable. 3. Influencing factors include experience, geographical location, company size and specific skills.

This tutorial will explain how to create pie, ring, and bubble charts using Chart.js. Previously, we have learned four chart types of Chart.js: line chart and bar chart (tutorial 2), as well as radar chart and polar region chart (tutorial 3). Create pie and ring charts Pie charts and ring charts are ideal for showing the proportions of a whole that is divided into different parts. For example, a pie chart can be used to show the percentage of male lions, female lions and young lions in a safari, or the percentage of votes that different candidates receive in the election. Pie charts are only suitable for comparing single parameters or datasets. It should be noted that the pie chart cannot draw entities with zero value because the angle of the fan in the pie chart depends on the numerical size of the data point. This means any entity with zero proportion
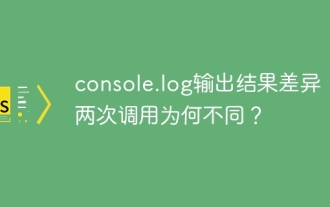
In-depth discussion of the root causes of the difference in console.log output. This article will analyze the differences in the output results of console.log function in a piece of code and explain the reasons behind it. �...
