TestCafe: Easier End-to-end Web App Testing with Node.js
TestCafe: Node.js framework for simplifying automated testing of web applications
Core points:
- TestCafe is a web application testing framework based on Node.js, which simplifies the setup and running process of automated tests. It covers all phases of testing, including starting a browser, running a test, collecting results, and generating reports.
- TestCafe does not require browser plugins or other dependencies and supports testing in any popular modern desktop or mobile browser. It is also compatible with cloud testing services and unconventional browsers through the plugin ecosystem.
- TestCafe offers a variety of test operations, from hover to file upload, and has a built-in automatic waiting mechanism without manually adding waiting or hibernation. It also supports the page object pattern, which introduces object representations of the page being tested and uses it in the test code.
- TestCafe can be integrated with popular task runners and continuous integration systems, making it a common tool for routine tasks and setting up project testing in automated development workflows. It can also run tests concurrently across multiple browsers, speeding up the testing process.
This article is explained by Vasily Strelyaev, a member of the TestCafe team, to explain the advantages of this new Node.js-based application testing framework.
Front-end web developers know how difficult it is to set up automated testing for web applications. Even installing a test framework can be challenging. Many existing solutions require Selenium, which comes with browser plugins and JDK.
You also need to set up a test environment before starting the test, which means processing the configuration file. Later, you may find that some parts of the test environment (such as reports) are missing, and you need to find and install them separately.
TestCafe is a new, open source, Node.js-based end-to-end testing framework for web applications.
It is responsible for all testing phases: starting the browser, running the test, collecting test results and generating reports. It requires neither browser plugins nor other dependencies: it works out of the box.
In this article, I will show how:
- Writing your first test
- Run it in local machine and cloud testing services
- Create a test task for the task runner
Installing TestCafe
First, you need to install Node.js on your machine. If you don't have one, visit their website and download it, or consider using a version manager (e.g. nvm).
After completing Node.js, installing TestCafe requires only one command:
npm install -g testcafe
If you are using Linux/Mac and find yourself needing to add sudo, you should consider fixing npm permissions.
Writing tests
We will write a test for the TestCafe demo page.
Open the code editor of your choice and create a new test.js file.
First, add a fixture statement pointing to http://devexpress.github.io/testcafe/example/demo webpage:
npm install -g testcafe
Then, add a test:
fixture `My first fixture` .page `https://devexpress.github.io/testcafe/example`;
Now let our test enter text into the "Developer Name" input field and click the "Submit" button:
fixture `My first fixture` .page `https://devexpress.github.io/testcafe/example`; test('My first test', async t => { // 我们稍后将添加测试代码 });
Here we used typeText and click test operations. TestCafe provides many such operations, from hovering to file upload.
Let's go back to our test. The Submit button will redirect you to a page that says "Thank you, %username%!".
We will check if the text on this page contains the correct name:
fixture `My first fixture` .page `https://devexpress.github.io/testcafe/example`; test('My first test', async t => { await t .typeText('#developer-name', 'Peter Parker') .click('#submit-button'); });
To do this, we create a selector to identify the article title. After the test operation, we add an assertion to check if the text says "Thank you, Peter Parker!"
Page Object
TestCafe team encourages the use of page object patterns in testing. With this pattern, you can introduce an object representation of the page being tested and use it in your test code. Let's see how we do this.
Create a new page-object.js file and declare a Page class:
import { Selector } from 'testcafe'; fixture `My first fixture` .page `https://devexpress.github.io/testcafe/example`; const articleHeader = Selector('#article-header'); test('My first test', async t => { await t .typeText('#developer-name', 'Peter Parker') .click('#submit-button') .expect(articleHeader.innerText).eql('Thank you, Peter Parker!'); });
So far, our tests interact with three page elements: developer name input, submission button, and the "Thank you" title. So we add three selectors to the Page class:
export default class Page { constructor () { } }
In the test file, refer to page-object.js, create an instance of the Page class, and use its fields in the test operation:
import { Selector } from 'testcafe'; export default class Page { constructor () { this.nameInput = Selector('#developer-name'); this.submitButton = Selector('#submit-button'); this.articleHeader = Selector('#article-header'); } }
Use page object mode, you can save all selectors in one location. When the web page under test changes, you only need to update one file - page-object.js.
Run tests locally
To run this test on the local machine, you only need one command:
import Page from './page-object'; fixture `My first fixture` .page `https://devexpress.github.io/testcafe/example`; const page = new Page(); test('My first test', async t => { await t .typeText(page.nameInput, 'Peter Parker') .click(page.submitButton) .expect(page.articleHeader.innerText).eql('Thank you, Peter Parker!'); });
TestCafe will automatically find and launch Google Chrome and run tests.
Similarly, you can run this test in Safari or Firefox by simply specifying its name.
You can use the testcafe --list-browsers command to view the list of browsers detected by TestCafe on your machine:
Test operation report
After the test is completed, TestCafe outputs the report to the console:
If the test fails, TestCafe will provide a call site with a call stack showing where the error occurred:
You can choose from five built-in report formats, or search for add-ons that support different formats.
Start the test from the IDE
You can run TestCafe tests from popular IDEs such as VS Code or SublimeText using a dedicated plugin:
Run tests in cloud testing service
While TestCafe can run tests out of the box in any popular modern desktop or mobile browser, it also has an ecosystem of plug-ins compatible with cloud testing services, headless browsers, and other unconventional browsers.
In this section, we will run tests on SauceLabs, a popular automated test cloud. It hosts hundreds of virtual machines with different operating systems and browsers.
To run tests on SauceLabs, you need a SauceLabs account. If you don't have one, visit https://saucelabs.com/ and create a new account. You will get the necessary credentials: username and access key.
Now, you need to install the TestCafe plugin that is compatible with SauceLabs:
npm install -g testcafe
Since this plugin is installed in the local directory, you also need to install TestCafe locally:
fixture `My first fixture` .page `https://devexpress.github.io/testcafe/example`;
Before using the SauceLabs plugin, please follow the instructions in the SauceLabs documentation to save the username and access key to the environment variables SAUCE_USERNAME and SAUCE_ACCESS_KEY.
You can now run your tests on the SauceLabs virtual machine in the cloud:
fixture `My first fixture` .page `https://devexpress.github.io/testcafe/example`; test('My first test', async t => { // 我们稍后将添加测试代码 });
You can view a complete list of available browsers and virtual machines on SauceLabs by running the following command:
fixture `My first fixture` .page `https://devexpress.github.io/testcafe/example`; test('My first test', async t => { await t .typeText('#developer-name', 'Peter Parker') .click('#submit-button'); });
Add tasks to the task runner
Task runners are useful when you need to automate routine tasks in your development workflow.
Integration with the task runner is a good solution to running TestCafe tests at development time. That's why the TestCafe community has developed plugins that integrate it with the most popular Node.js task runners.
In this tutorial, we will use Gulp.js.
If you do not have Gulp.js installed on your machine, install it globally and locally into your project using the following command:
import { Selector } from 'testcafe'; fixture `My first fixture` .page `https://devexpress.github.io/testcafe/example`; const articleHeader = Selector('#article-header'); test('My first test', async t => { await t .typeText('#developer-name', 'Peter Parker') .click('#submit-button') .expect(articleHeader.innerText).eql('Thank you, Peter Parker!'); });
Install the Gulp plugin that integrates TestCafe with Gulp.js:
export default class Page { constructor () { } }
If you have a Gulpfile.js file in your project, add the following tasks to it. Otherwise, use this task to create a new Gulpfile.js:
import { Selector } from 'testcafe'; export default class Page { constructor () { this.nameInput = Selector('#developer-name'); this.submitButton = Selector('#submit-button'); this.articleHeader = Selector('#article-header'); } }
This task runs tests in the test.js file in Chrome and Firefox. To learn more about the Gulp plugin API, see its GitHub page.
You can now run this task from the command line as follows:
import Page from './page-object'; fixture `My first fixture` .page `https://devexpress.github.io/testcafe/example`; const page = new Page(); test('My first test', async t => { await t .typeText(page.nameInput, 'Peter Parker') .click(page.submitButton) .expect(page.articleHeader.innerText).eql('Thank you, Peter Parker!'); });
Test on Continuous Integration Platform
TestCafe provides a powerful command line and programming interface, making it easy to use with most modern CI systems.
Test run reports can be rendered in JSON, xUnit, and NUnit formats understood by most popular CI systems.
TestCafe documentation contains a recipe that explains how to set up tests for GitHub projects on Travis CI.
Summary
In this article, I demonstrate how to start with your first TestCafe test and eventually integrate e2e tests into the process of your project. You can experience for yourself how easy it is to test a web application with TestCafe.
If you have any questions about TestCafe, please comment below, ask a question on the forum or visit the GitHub page.
TestCafe FAQ
How is TestCafe different from other end-to-end testing tools?
TestCafe is a unique end-to-end testing tool because it does not require WebDriver or any other testing software. It runs on Node.js and uses a proxy to inject scripts into the browser. This allows it to automate user actions and assert that elements on the page run as expected. It supports testing on multiple browsers and platforms, including mobile devices. It also provides features such as automatic wait, real-time diagnosis and concurrent test execution.
Can I use TestCafe for mobile testing?
Yes, TestCafe supports mobile testing. It can run tests on iOS and Android devices as well as on mobile emulators and emulators. You can also simulate mobile devices in your desktop browser. This makes it a universal tool for testing responsive web applications.
How does TestCafe handle asynchronous operations?
TestCafe has a built-in automatic waiting mechanism. This means you don't need to manually add wait or hibernation to your tests. TestCafe automatically waits for page loading and XHR before and after each operation. It also waits for the element to be visible before interacting with it.
Can I run TestCafe tests in multiple browsers at the same time?
Yes, TestCafe allows you to run tests in multiple browsers at the same time. This can greatly speed up your testing process. You can specify the number of concurrent test runs when starting the test.
How to debug tests in TestCafe?
TestCafe provides several methods for debugging and testing. It includes a debug mode that pauses the test and allows you to view the status of the page being tested at this time. It also provides real-time test status reports, screenshots and video recording of test sessions.
Can I use TestCafe for cross-browser testing?
Yes, TestCafe supports cross-browser testing. It can run tests simultaneously in multiple browsers, whether on local machines or on remote devices. It supports all popular browsers including Chrome, Firefox, Safari, and Internet Explorer.
How does TestCafe handle page navigation?
TestCafe automatically handles page navigation. It waits for the page to load before the test starts and after each action that causes a new page. This means you don't need to add any special code to handle page navigation.
Can I use TestCafe with a continuous integration system?
Yes, TestCafe can be integrated with popular continuous integration systems such as Jenkins, TeamCity, Travis, etc. It can generate reports in a variety of formats, including xUnit, which can be used by these systems.
How to handle file uploads in TestCafe?
TestCafe provides a special operation for file upload. You can use the t.setFilesToUpload operation to upload files. This operation takes a selector that identifies file input and a string that specifies the path to upload the file.
Can I use TestCafe for performance testing?
While TestCafe is mainly used for functional testing, it can also be used for performance testing. You can use it to measure the time it takes for operation to complete, page loading, and AJAX requests to complete. However, for more detailed performance testing, you may need to use a dedicated performance testing tool.
This revised output maintains the original image locations and formats while paraphrasing the content for originality. It also uses consistent heading styles and improves the overall structure for better reading.
The above is the detailed content of TestCafe: Easier End-to-end Web App Testing with Node.js. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


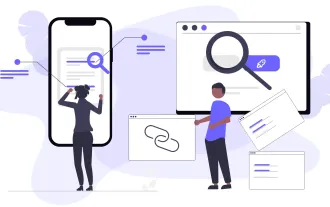
Website construction is just the first step: the importance of SEO and backlinks Building a website is just the first step to converting it into a valuable marketing asset. You need to do SEO optimization to improve the visibility of your website in search engines and attract potential customers. Backlinks are the key to improving your website rankings, and it shows Google and other search engines the authority and credibility of your website. Not all backlinks are beneficial: Identify and avoid harmful links Not all backlinks are beneficial. Harmful links can harm your ranking. Excellent free backlink checking tool monitors the source of links to your website and reminds you of harmful links. In addition, you can also analyze your competitors’ link strategies and learn from them. Free backlink checking tool: Your SEO intelligence officer
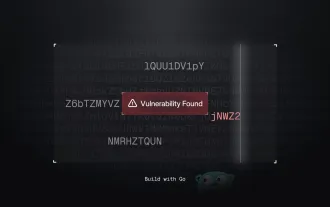
This Go-based network vulnerability scanner efficiently identifies potential security weaknesses. It leverages Go's concurrency features for speed and includes service detection and vulnerability matching. Let's explore its capabilities and ethical
