Using the Selenium Web Driver API with PHPUnit
Feb 17, 2025 pm 12:20 PMThis article explores using Facebook's webdriver package for browser emulation in PHP, building upon a previous article (not included here) that covered Selenium with PHPUnit. It focuses on acceptance testing and automating browser interactions.
Key Differences from PHPUnit's Selenium Extension:
- Facebook's webdriver requires manual browser session closure using
tearDown()
, unlike PHPUnit's automatic handling. - It leverages the
RemoteWebDriver
class for Selenium server interaction.
Implementation Steps:
-
Installation: Use Composer to install the Facebook webdriver package:
composer require facebook/webdriver --dev
-
Test Class Setup: Create a PHPUnit test class (e.g.,
UserSubscriptionTestFB.php
) extendingPHPUnit_Framework_TestCase
. ThesetUp()
method initializes theRemoteWebDriver
instance, specifying the Selenium server address (http://localhost:4444/wd/hub
by default) and desired browser capabilities (e.g., Firefox or Chrome).public function setUp() { $this->webDriver = RemoteWebDriver::create('http://localhost:4444/wd/hub', DesiredCapabilities::firefox()); }
Copy after login -
Browser Closure: The
tearDown()
method is crucial for closing the browser session after each test:public function tearDown() { $this->webDriver->quit(); }
Copy after login -
Form Interaction: The
fillFormAndSubmit()
method usesfindElement()
withWebDriverBy
to locate form elements and interact with them.public function fillFormAndSubmit($inputs) { $this->webDriver->get('http://vaprobash.dev/'); // Replace with your URL $form = $this->webDriver->findElement(WebDriverBy::id('subscriptionForm')); // Replace with your form ID foreach ($inputs as $input => $value) { $form->findElement(WebDriverBy::name($input))->sendKeys($value); } $form->submit(); }
Copy after login -
Test Cases: Test methods use data providers (assumed from the previous article) to supply test inputs. Assertions verify expected outcomes (success or error messages). Example:
/** * @dataProvider validInputsProvider */ public function testValidFormSubmission(array $inputs) { $this->fillFormAndSubmit($inputs); $content = $this->webDriver->findElement(WebDriverBy::tagName('body'))->getText(); $this->assertEquals('Everything is Good!', $content); // Replace with your success message }
Copy after login
-
Screenshot Capture: The
takeScreenshot()
method allows capturing screenshots during test execution:$this->webDriver->takeScreenshot(__DIR__ . "/../../public/screenshots/screenshot.jpg");
Copy after login -
Waiting for Elements: The
wait()
method withuntil()
orWebDriverExpectedCondition
handles asynchronous page loading:$this->webDriver->wait(10, 300)->until(WebDriverExpectedCondition::presenceOfElementLocated(WebDriverBy::name('username')));
Copy after login -
Advanced Interactions: The article covers more advanced interactions such as drag-and-drop, alert handling, and keyboard shortcuts.
-
Headless Testing: The article explains how to use XVFB (X virtual framebuffer) for headless browser testing on systems without a graphical display. Both methods (running XVFB separately and using
xvfb-run
) are detailed.
Useful Links (repeated from original):
- https://www.php.cn/link/5847ac0c8efb8552d1b7c42a4c3f2418
- https://www.php.cn/link/676bc6cef834fe54277b1954f6cd4c5c
- https://www.php.cn/link/f31bad5d6425dd6d172c786a1bffe4a7
The article concludes by emphasizing Selenium's broader utility beyond testing, including browser automation tasks. A FAQ section provides further guidance on installation, basic tests, exception handling, assertions, browser selection, element interaction, waiting for elements, screenshot capture, alert handling, and parallel test execution.
The above is the detailed content of Using the Selenium Web Driver API with PHPUnit. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
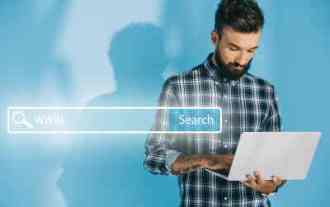
11 Best PHP URL Shortener Scripts (Free and Premium)
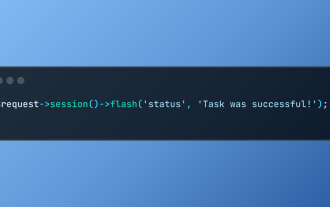
Working with Flash Session Data in Laravel
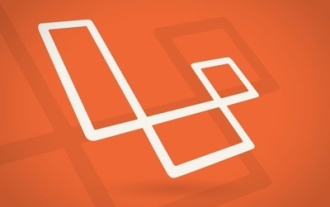
Build a React App With a Laravel Back End: Part 2, React
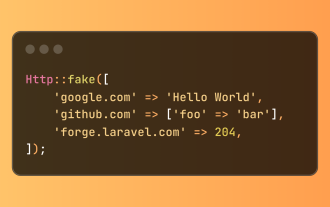
Simplified HTTP Response Mocking in Laravel Tests
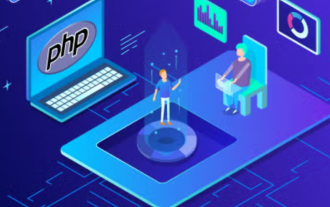
cURL in PHP: How to Use the PHP cURL Extension in REST APIs
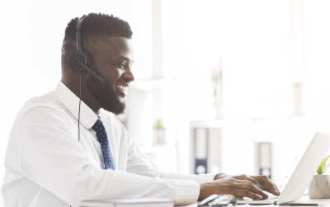
12 Best PHP Chat Scripts on CodeCanyon
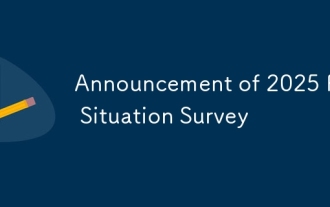
Announcement of 2025 PHP Situation Survey
