Integrate Elasticsearch with Silex
Efficient integration of Elasticsearch and Silex: Building high-performance applications
This article discusses how to directly read Elasticsearch data by building a small Silex application to achieve efficient integration of the two. This requires setting Silex as a dependency in an existing Composer-based project and adding the Elasticsearch PHP SDK to Composer.
Core points:
- Under the integration of the two by creating a small Silex application to read data directly from Elasticsearch.
- Expose the Elasticsearch PHP SDK as a service to Silex's dependency injection container Pimple, making it easy to access the Elasticsearch Client class object.
- Create Silex controller to build Elasticsearch query parameters and execute the query. According to the results, use Twig template to render nodes or return a 404 error.
- Integrate Drupal, Elasticsearch and Silex to build high-performance applications: Drupal as a content management system, Elasticsearch as a high-performance data storage, and Silex as a PHP framework for fast data retrieval.
The integration of Drupal 7 and Elasticsearch has been explored in the previous article, with the goal of combining these two open source technologies to build high-performance applications with both advantages. (For related code, please refer to the Code Repository Link )
Next, we will create a small Silex app that reads and returns data directly from Elasticsearch.
Silex application construction
Silex is an excellent PHP microframework developed by the Symfony team. It is mainly based on Symfony components, but is more simplified and easy to use. The method to quickly build a Silex application is as follows:
- Add to an existing project as a Composer dependency:
<code>"silex/silex": "~1.2",</code>
- Create a new project with Silex scaffolding:
<code>composer.phar create-project fabpot/silex-skeleton</code>
Eventing Elasticsearch PHP SDK is required for accessing Elasticsearch. Add it to Composer:
<code>"elasticsearch/elasticsearch": "~1.0",</code>
If you use Twig to output data, you also need to add a Twig bridging component (ignored if it already exists):
<code>"symfony/twig-bridge": "~2.3"</code>
To use the SDK, we can expose it as a service to Pimple (Silex's dependency injection container). This can be done in multiple locations (see the code repository for specific examples), but after instantiating the Silex application, you can add the following code:
$app['elasticsearch'] = function() { return new Client(array()); };
This creates a service in the application called elasticsearch
, instantiating the Elasticsearch Client class object. Don't forget to go to the top use
The category:
use Elasticsearch\Client;
Now, the Elasticsearch client can be accessed anywhere via $app['elasticsearch']
.
Connect Elasticsearch
The previous article has imported node data into the node
index, and each node type corresponds to an Elasticsearch document type. For example, the following code returns all nodes of article
type:
<code>http://localhost:9200/node/article/_search</code>
We already know how to instantiate the Elasticsearch SDK client and now we can use it. One way is to create a controller:
<code>"silex/silex": "~1.2",</code>
Controller location depends on how Silex applications are organized. In my example it's in the src/Controller
folder and is automatically loaded by Composer.
We also need to create a route to map to this controller. Again, there are multiple ways to deal with this, in my example I have a src/
file located in the routes.php
folder and introduced in index.php
:
<code>composer.phar create-project fabpot/silex-skeleton</code>
In this example, the controller obtains the Elasticsearch client, builds the query parameters, executes the query, checks the results, and uses the Twig template to render if the node is found, otherwise it returns a 404 error.
Using Twig requires registering a Twig service provider:
<code>"elasticsearch/elasticsearch": "~1.0",</code>
Then create the template file in the templates/
folder.
Conclusion
This article shows how to quickly build a Silex application and use it to return data from Elasticsearch. The goal is not to explain the details of these technologies, but to explore their integration solutions. As a content management system, Elasticsearch as a high-performance data storage, and Silex as a fast data retrieval framework, the combination of the three can build high-performance applications.
(More discussions on error handling, performance optimization, security policies, etc., as well as more detailed code examples and FAQs)
The above is the detailed content of Integrate Elasticsearch with Silex. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
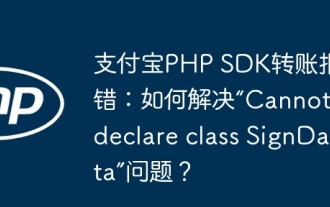
Alipay PHP...
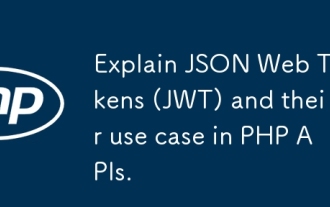
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
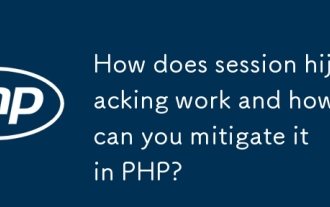
Session hijacking can be achieved through the following steps: 1. Obtain the session ID, 2. Use the session ID, 3. Keep the session active. The methods to prevent session hijacking in PHP include: 1. Use the session_regenerate_id() function to regenerate the session ID, 2. Store session data through the database, 3. Ensure that all session data is transmitted through HTTPS.
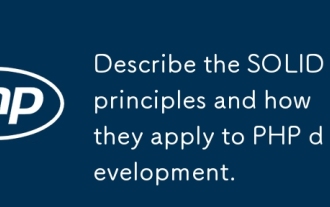
The application of SOLID principle in PHP development includes: 1. Single responsibility principle (SRP): Each class is responsible for only one function. 2. Open and close principle (OCP): Changes are achieved through extension rather than modification. 3. Lisch's Substitution Principle (LSP): Subclasses can replace base classes without affecting program accuracy. 4. Interface isolation principle (ISP): Use fine-grained interfaces to avoid dependencies and unused methods. 5. Dependency inversion principle (DIP): High and low-level modules rely on abstraction and are implemented through dependency injection.
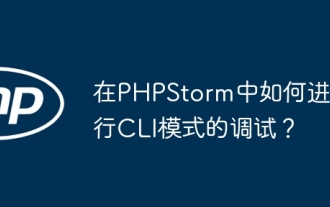
How to debug CLI mode in PHPStorm? When developing with PHPStorm, sometimes we need to debug PHP in command line interface (CLI) mode...
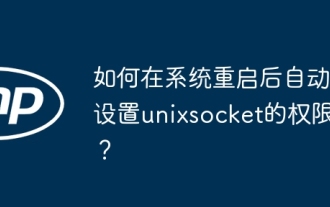
How to automatically set the permissions of unixsocket after the system restarts. Every time the system restarts, we need to execute the following command to modify the permissions of unixsocket: sudo...
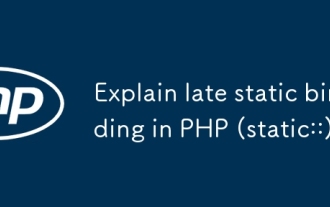
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.
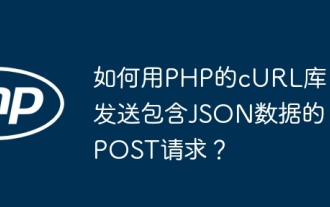
Sending JSON data using PHP's cURL library In PHP development, it is often necessary to interact with external APIs. One of the common ways is to use cURL library to send POST�...
