Creating a WebGL Game with Unity 5 and JavaScript
Easy guide to creating WebGL games with Unity 5 and JavaScript
Core points:
- Unity 5's WebGL exporter simplifies the process of publishing games to the web, using WebGL and asm.js to render interactive 3D graphics directly in the browser.
- This tutorial guides you how to create a simple game in Unity using JavaScript (UnityScript) that includes setting up projects, creating hero characters that bounce on the platform, and adding a first-person perspective for an immersive gaming experience.
- Scripting in Unity allows dynamic game behavior, such as programmatic platform generation and responsive controls used by mouse input to affect hero characters in the game.
- The key steps to completing the game include adding a user interface with a start button in the game menu, and configuring build settings to export the project as a WebGL application so it can be played in a WebGL-enabled browser.
- This tutorial ends with further improvement suggestions such as adding scores, more platform types and additional input methods, showing Unity's versatility as a cross-platform game development tool.
This article was peer-reviewed by Nilson Jacques Collins, Marc Towler and Matt Burnett. Thanks to all the peer reviewers of SitePoint for getting SitePoint content to its best!
Unity is a cross-platform game engine used to develop video games for PCs, gaming consoles, mobile devices and websites. The latest version (Unity 5) comes with a WebGL exporter, which means developers can easily publish their games to the web. As the name suggests, the WebGL exporter utilizes WebGL (a JavaScript API for rendering interactive 3D computer graphics) and asm.js (a subset of JavaScript developed by Mozilla, touted as the "assembly language for the web"). You can read more about Asm.js and WebGL for Unity and Unreal Engine here.
In this tutorial, I will show you how to get started with Unity. I'll also show you how to create simple games in Unity using JavaScript and how to export your games to the web.
You can view finished games here (you need a desktop browser that supports WebGL), or you can download game files and project files from our GitHub repository.
Let's get started...
Instructions on JavaScript in Unity
When we talk about JavaScript in Unity, we are actually talking about UnityScript, which is a type of JS dialect. Unity itself often mentions this JavaScript, however, more cynical observers believe that "Unity uses JavaScript" is a marketing strategy. In any case, we should make it clear that UnityScript does not comply with any ECMAScript specifications—it also did not try to do so. You can find a good overview of the differences here.
Installation of Unity
To start this tutorial, we need to run the Unity version, which can be downloaded from here. Unity has a installer for Windows and Mac OS X. Linux users can run Unity via Wine, but your results may vary.
After the installation is complete, we can start! Let's open Unity and create a new 3D project.
Project Settings
After Unity is first opened, we should take a moment to understand the main window:
- The leftmost panel is Hire, which outlines all the elements in the current scene. The scene is like a view of the game, such as a level or a menu. There should be a main camera element and a directional light element at present.
- The middle is the scene view, which illustrates the camera and lights in the 3D space in the form of an icon.
- Next to the Scene tab is a Game tab that displays the game itself, just like the player sees. This is designed to test the game in the editor. On the right is the
- Inspector panel where you can modify the element settings. Let's try it out, click on the directional light in the hierarchy. Now we should see a lot of information about this light and be able to turn off its shadow using Shadow Type: No Shadow. At the bottom is the Project window, which displays the file view required to develop the game.
- Now that we are familiar with the interface of Unity, there is one more thing to do before starting development: save the current scene. File>Save Scene Open a
dialog box that points to a folder named Assets. One common way to organize files in Unity is to use subfolders. So add a new folder named Scenes to the Assets folder and save the scene in this folder named Level.unity. Create a hero Our game will be composed of one hero, jumping from one platform to another, jumping higher and higher. If it misses one and falls into nothingness, the game will fail. So let's start with creating a hero. Since the player will watch the game from a first-person perspective, the appearance of the hero is not important, we can use standard sphere geometry. The advantage of the sphere is that it can be created in several steps and it fits the physical properties we need to jump. Create
in thehierarchy
and edit the following properties using the inspector:Let's test what we do by pressing the play button. We should see a sphere in 3D space, located in front of the skyline.
1 2 |
|
In order for the hero to fall, it must add weight. Therefore, we need to add a component to the sphere by clicking the corresponding button in the Inspector and selecting Rigid Body. And since we don't want the hero to rotate, we will freeze the heroes in the rigid body component by turning on the constraint and selecting all axes in the Rotate row. When the scene is played again, we should be able to watch the hero fall.
To save the hero from endless falls, we will create a flat box that serves as a platform. To do this, we have to add a cube and set the Scale.Y value to 0.1. Replaying the scene confirms that the hero lands safely on the platform, although I have to admit it doesn't look natural. So how do we make the hero rebound? By adding some physical materials.
Make the hero rebound
First, we need to create a new physical material for the sphere to make it elastic. To do this, create a new folder called Materials in the Assets folder, and then create a new physical material here. Let's name it Bouncy_Sphere. The value we need to adjust in the inspector is:
1 2 |
|
If we add this material to Sphere Collider, this will make the sphere bounce up and down, but always reach the same height. In order for the sphere to jump higher and higher with each bounce, we also have to add some physical material to the platform. To do this, we create another material called Bouncy_Platform and change its value to:
1 2 3 4 5 |
|
To achieve consistency here, we should also rename the cube element to Platform by double-clicking it in the hierarchy. When we start the game now, we can notice that the sphere jumps higher and higher each time.
We will also create a new standard material called Platform to give the platform a certain color. After creating this material, use #C8FF00 as the albedo color (albedo is a label in the Unity UI), and drag and drop this material onto the platform element. It should be yellow now.
Add a first-person perspective
To add a first-person perspective, we drag and drop the camera (in the hierarchy) onto the sphere. This will make the camera a child of the hero and cause the camera to follow the sphere as it moves. The camera properties must also be adjusted to:
1 2 3 4 5 |
|
We will also create a spotlight as the second child element of the sphere. This will give the player an idea of the hero's current jump height. Adjust the value of the spotlight to:
1 2 3 4 5 6 |
|
(The subsequent steps will be briefly described due to space limitations, and the core logic and key code snippets will be retained)
Skill the programming controller, programmatic creation platform, add game menu, add start game button, publish project as a WebGL browser game, etc., please refer to the original document. Due to space limitations, I will not repeat it here. The key is to understand the core concepts of Unity's scripting system, game object management, physics engine and UI system, and practice them in combination with the code examples in the tutorial.
FAQ (FAQ)
(The FAQ section is also simplified due to space limitations, retaining core questions and brief answers)
How to optimize my WebGL game for better performance?
Reduce the number of draw calls, use less material and combinatorial mesh, use level of detail (LOD), compress textures and audio files, and use Unity's profiler to identify and fix performance bottlenecks.
Can I use WebGL for mobile game development?
Yes, but WebGL games may consume more resources than native applications and require careful optimization.
How to debug my WebGL game?
You can debug using browser tools such as Chrome's developer tools or Firefox's web console.
How to add multiplayer features to my WebGL games?
The backend server is required to manage communication between players, and you can use Unity's built-in network system UNet or a third-party solution (such as Photon).
How to make money on my WebGL game?
In-game ads, in-app purchases or freemium models are available.
How to improve the graphics of my WebGL game?
High quality textures, advanced lighting techniques and shaders are available.
How to make my WebGL games respond to different screen sizes?
Create a flexible and scalable user interface using Unity's UI system, and use the Screen class to obtain player screen size information and adjust the game accordingly.
How to add sound effects and music to my WebGL game?
Use Unity's audio system, import audio files and control playback using AudioSource and AudioClip classes, and you can also use Audio Mixer to create complex soundscapes.
How to protect my WebGL game from cheating?
We can implement server-side verification of game data, obfuscating JavaScript code, and using secure communication protocols.
How to update my WebGL game after posting?
Recompile the game and make changes in Unity, and upload the new build to the server.
Hope this simplified version helps you! Remember that understanding the core concepts and practical experience of the Unity engine is essential for the successful development of WebGL games.
The above is the detailed content of Creating a WebGL Game with Unity 5 and JavaScript. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
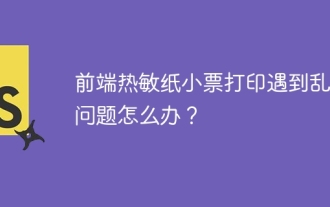
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
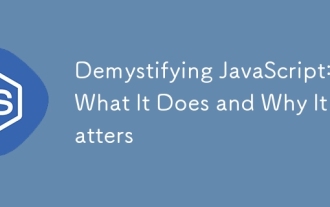
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
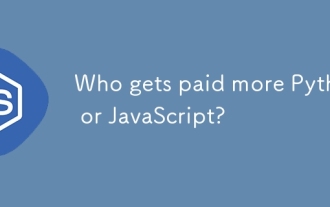
There is no absolute salary for Python and JavaScript developers, depending on skills and industry needs. 1. Python may be paid more in data science and machine learning. 2. JavaScript has great demand in front-end and full-stack development, and its salary is also considerable. 3. Influencing factors include experience, geographical location, company size and specific skills.
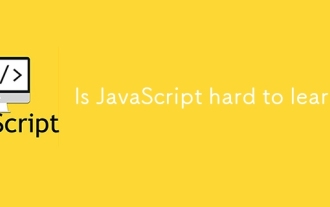
Learning JavaScript is not difficult, but it is challenging. 1) Understand basic concepts such as variables, data types, functions, etc. 2) Master asynchronous programming and implement it through event loops. 3) Use DOM operations and Promise to handle asynchronous requests. 4) Avoid common mistakes and use debugging techniques. 5) Optimize performance and follow best practices.
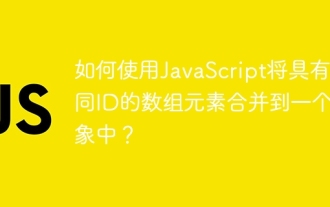
How to merge array elements with the same ID into one object in JavaScript? When processing data, we often encounter the need to have the same ID...
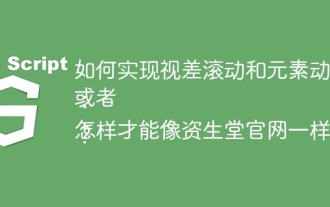
Discussion on the realization of parallax scrolling and element animation effects in this article will explore how to achieve similar to Shiseido official website (https://www.shiseido.co.jp/sb/wonderland/)...
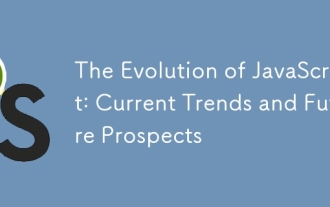
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
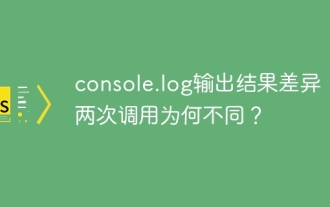
In-depth discussion of the root causes of the difference in console.log output. This article will analyze the differences in the output results of console.log function in a piece of code and explain the reasons behind it. �...
