Functional Reactive Programming with Elm: An Introduction
Core points
- Elm is a functional programming language compiled into JavaScript, focusing on simplifying and enhancing front-end development. It uses type inference, is reactive and combines functional programming patterns such as pure views, reference transparency, and immutable data.
- Elm uses modes such as immutable data, pure views describing DOM, one-way data streams, centralized state and centralized data variation descriptions, and limited side effects. These patterns make applications easier to predict, maintain, and robust.
- Elm's security feature avoids the possibility of a value being empty, forcing developers to handle all alternative paths in the application. This provides a lot of confidence for the application and rarely sees runtime errors in Elm applications.
- Elm's architecture follows the Model-View-Update (MVU) pattern, which is different from the Model-View-Controller (MVC) pattern used in many other languages. This pattern makes it easy to build code structures and infer how data flows through the application.
Elm is a functional programming language that has attracted considerable attention recently. This article explores what it is and why you should pay attention to it.
Elm's main focus is to make front-end development simpler and more robust. Elm compiles to JavaScript, so it can be used to build applications for any modern browser.
Elm is a statically typed language with type inference. Type inference means we don't need to declare all types ourselves, we can have the compiler infer many types for us. For example, by writing one = 1
, the compiler knows that one
is an integer.
Elm is an almost purely functional programming language. Elm is based on many functional patterns such as pure views, reference transparency, immutable data, and controlled side effects. It is closely related to other ML languages such as Haskell and Ocaml.
Elm is reactive. Everything in Elm flows through signals. The signals in Elm pass messages over time. For example, clicking a button will send a message via a signal.
You can think of signals as similar to events in JavaScript, but unlike events, signals are first-class citizens in Elm and can be passed, transformed, filtered, and combined.
Elm Syntax
Elm syntax is similar to Haskell, because both are ML family languages.
greeting : String -> String greeting name = "Hello" ++ name
This is a function that takes one string and returns another string.
Why use Elm?
To understand why you should follow Elm, let's discuss some front-end programming trends over the past few years:
Describe the state instead of the transition DOM
Not long ago, we built our application by manually changing the DOM (for example, using jQuery). As the application grows, we introduce more states. Having to encode transitions between all states exponentially increases the complexity of the application, making it more difficult to maintain.
Rather than doing this, React and other libraries popularized the concept of focusing on describing a specific DOM state and then having the library handle DOM transformations for us. We only focus on describing discrete DOM states, not how we get there.
This leads to a significant reduction in code that needs to be written and maintained.
Event and data conversion
In terms of application state, it is common to change the state yourself, such as adding comments to an array.
Instead of doing this, we just need to describe how the application state needs to be changed based on the event, and then let something else apply these transformations for us. In JavaScript, Redux makes this way of building applications popular.
The advantage of this is that we can write "pure" functions to describe these transformations. These functions are easier to understand and test. An additional benefit is that we can control where the application state changes, making our application easier to maintain.
Another benefit is that our views do not need to know how to change the state, they only need to know which events to dispatch.
One-way data flow
Another interesting trend is to let all application events flow in a one-way manner. Rather than allowing any component to communicate with any other component, we send messages through the central message pipeline. This central pipeline applies the transformations we want and broadcasts changes to all parts of the application. Flux is an example.
By doing this, we can better understand all the interactions that occur in the application.
Immutable Data
Variable data makes it difficult to limit its location to change, as any component that has access to it can add or delete content. This leads to unpredictability, as the state can be changed anywhere.
By using immutable data, we can avoid this by strictly controlling the changing location of the application state. Combining immutable data with functions that describe transformations, we get a very powerful workflow, and immutable data helps us enforce a one-way flow by not allowing us to change states at unexpected locations.
Centralized state
Another trend in front-end development is to use centralized "atoms" to save all states. This means we put all the state in a big tree instead of scattering it across the components.
In a typical application, we usually have global application states (e.g., user collections) and component-specific states (e.g., visibility states of specific components). It is controversial whether storing both states in one place is beneficial. But there is a big benefit to at least keeping all application states in one place, that is, providing consistent states across all components of the application.
Pure Components
Another trend is to use pure components. This means that given the same input, the component will always render the same output. No side effects occur inside these components.
This makes it much easier to understand and test our components than before, because they are easier to predict.
Back to Elm
These are excellent models that make applications more robust and easier to predict and maintain. However, to use them correctly in JavaScript, we need to be careful to avoid doing something in the wrong places (e.g. changing the state inside the component).
Elm is a programming language that has considered many of these patterns from the beginning. It makes adopting and using them very natural without worrying about doing something wrong.
In Elm, we build the application by using the following methods:
- Immutable Data
- Description Pure View of DOM
- One-way data flow
- Centralized state
- Centralized data mutation description position
- Restricted side effects
Safety
One of the great advantages of the Elm is the security it provides. By completely avoiding the possibility of a value being null, it forces us to process all alternative paths in the application.
For example, in JavaScript (and many other languages) you can get a runtime error by doing the following:
greeting : String -> String greeting name = "Hello" ++ name
This will return NaN in JavaScript, which you need to deal with to avoid runtime errors.
If you try something similar in Elm:
var list = [] list[1] * 2
The compiler will reject this and tell you that List.head list
returns the Maybe type. The Maybe type may or may not contain values, and we must deal with cases where the value is Nothing.
list = [] (List.head list) * 2
This provides a lot of confidence for our application. Runtime errors are rarely seen in Elm applications.
(The following parts are similar to the original text, make a little adjustment to avoid duplication)
(Sample Application, Let’s go over it piece by piece etc., due to the length of the article, it is omitted here. The original text has fully described the code function and will not be repeated here.)
Conclusion
Elm is an exciting programming language that takes an excellent model for building reliable applications. It has a clean syntax and has many security features built in to avoid runtime errors. It also has an excellent static type system, which is very helpful during the reconstruction process and since it uses type inference it does not hinder development.
Learning how to build the learning curve for an Elm application is not easy, because applications programmed using functional reactive are different from what we are used to, but it is worth it.
(Additional Resources, Frequently Asked Questions, part, due to the length of the article, it is omitted here. The original text has fully described the relevant information and will not be repeated here.)
The above is the detailed content of Functional Reactive Programming with Elm: An Introduction. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










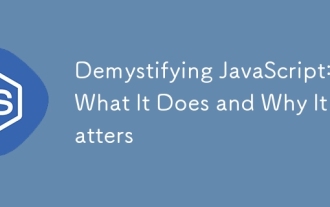
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
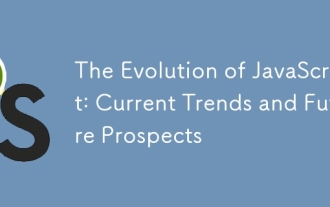
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
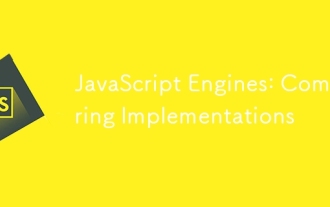
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
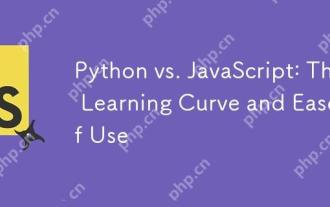
Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
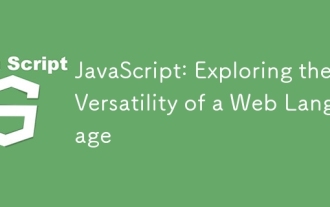
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
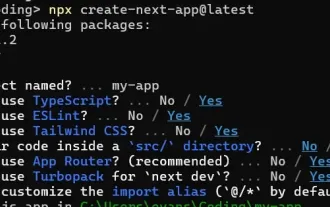
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
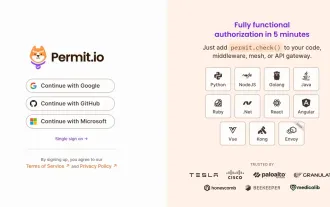
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
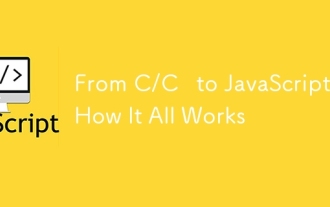
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
