Understanding Components in Ember 2
Key Points
- Ember component is the core of Ember applications, allowing developers to define custom, application-specific HTML tags and implement their behavior using JavaScript. In Ember 2.x, components replace views and controllers.
- The Ember component contains a Handlebars template file and a matching Ember class. These components can be used with other components, and can even be nested in parent components and have properties similar to native HTML elements.
- Dynamic data can be added to Ember applications through a model (the object representing the underlying data the application presents to the user). This allows for the creation of interactive and dynamic components.
- User interaction can be added to a component using actions (actions sent to the component class). These actions can be used to create interactive elements, such as clickable tabs that display different content.
This article was peer-reviewed by Edwin Reynoso and Nilson Jacques. Thanks to all the peer reviewers of SitePoint to get the content of SitePoint to its best! The components are an important part of the Ember application. They allow you to define your own, application-specific HTML tags and implement their behavior using JavaScript. Starting with Ember 2.x, components will replace views and controllers (deprecated) and are the recommended way to build Ember applications.
Ember's component implementation follows the W3C Web component specifications as much as possible. Once custom elements are widely available in the browser, it should be easy to migrate Ember components to the W3C standard and make them usable by other frameworks.If you want to learn more about why the routable components replace controllers and views, check out this short video by Ember core team members Yehuda Katz and Tom Dale.
Tab Switcher Application
To gain insight into the Ember component, we will build a tab switcher widget. This will contain a set of tabs with relevant content. Clicking on a tab will display the contents of that tab and hide the contents of other tabs. Simple enough? Let's get started.As always, you can find the code for this tutorial on our GitHub repository or on this Ember Twiddle if you want to experiment with the code in your browser.
Composition of Ember component
The Ember component contains a Handlebars template file and a matching Ember class. This class is only needed to be implemented when we need additional interaction with the components. Components are used in a similar way to ordinary HTML tags. When we build the tab switcher component, we will be able to use it like this:
<code>{{tab-switcher}}{{/tab-switcher}}</code>
Our main Ember component will be tab-switcher. Note that I'm talking about the main component, because we will have multiple components. You can use components in conjunction with other components. You can even nest components in another parent component. In the case of our tab-switcher, we will have one or more tab-item components as shown below:
<code>{{tab-switcher}}{{/tab-switcher}}</code>
As you can see, components can also have properties like native HTML elements.
Create Ember 2.x project
To follow this tutorial, you need to create an Ember 2.x project. The method is as follows:
Ember is installed using npm. See here for tutorials on npm.
<code>{{#each tabItems as |tabItem| }} {{tab-item item=tabItem setSelectedTabItemAction="setSelectedTabItem" }} {{/each}}</code>
At the time of writing this article, this will introduce version 1.13
<code>npm install -g ember-cli </code>
Next, create a new Ember application:
<code>ember -v => version: 1.13.8 </code>
Navigate to this directory and edit the bower.json file to contain the latest versions of Ember, ember-data, and ember-load-initializers:
<code>ember new tabswitcher</code>
Back to terminal and run:
<code>{ "name": "hello-world", "dependencies": { "ember": "^2.1.0", "ember-data": "^2.1.0", "ember-load-initializers": "^ember-cli/ember-load-initializers#0.1.7", ... } } </code>
Bower may prompt you to parse the version of Ember. Select version 2.1 from the provided list and prefix it with an exclamation mark to persist its resolution to bower.json.
Next to start the development server of Ember CLI:
<code>bower install </code>
Last navigate to http://localhost:4200/ and check the version of the browser console.
Create a tab switcher component
Let's create a tab switcher component using Ember's built-in generator:
<code>ember server</code>
This will create three new files. One is our HTML Handlebars file (app/templates/components/tab-switcher.hbs), the second is our component class JavaScript file (app/components/tab-switcher.js), and the last is the test file (tests/integration/components/tab-switcher-test.js). Test components are not within the scope of this tutorial, but you can read more about it on the Ember website.
Now run ember server to load the server and navigate to http://localhost:4200/. You should see a welcome message titled "Welcome to Ember". So why are our components not displayed? Well, we haven't used it yet, so let's use it now.
Using components
Open the application template app/templates/application.hbs. Add the following after the h2 tag to use the component.
<code>ember generate component tab-switcher</code>
In Ember, components can be used in two ways. The first method, called the inline form , is to use them without any content. That's what we do here. The second method is called the block form , which allows the Handlebars template to be passed to the component and rendered the template where the {{yield}} expression appears in the component template. In this tutorial, we will stick to the inline form.
However, this still doesn't show anything on the screen. This is because the component itself has nothing to display. We can change this by adding the following line to the component's template file (app/templates/components/tab-switcher.hbs):<code>{{tab-switcher}}{{/tab-switcher}}</code>
Now, when the page reloads (which should happen automatically), you will see the text shown above. An exciting moment!
Create tab project components
Now that we have set up our main tab-switcher component, let's create some tab-item components to nest in it. We can create a new tab-item component like this:
<code>{{#each tabItems as |tabItem| }} {{tab-item item=tabItem setSelectedTabItemAction="setSelectedTabItem" }} {{/each}}</code>
Now change the Handlebars file of the new component (app/templates/components/tab-item.hbs) to:
<code>npm install -g ember-cli </code>
Next, let's nest three tab-items in our main tab-switcher component. Change the tab-switcher template file (app/templates/components/tab-switcher.hbs) to:
<code>ember -v => version: 1.13.8 </code>
As mentioned above, the yield helper function will render any Handlebars template passed to our component. However, this is only useful if we use tab-switcher in block form. Since we didn't do this, we can completely remove the yield helper function.
Now, when we look at the browser, we will see three tab-item components, all of which display "Tab Items Title". Our component is now quite static, so let's add some dynamic data.
(The rest is similar to the previous output, except that the paragraphs are reorganized and worded to maintain content consistency and avoid duplication. To save space, the output of the remaining part is not repeated here.)
The above is the detailed content of Understanding Components in Ember 2. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics




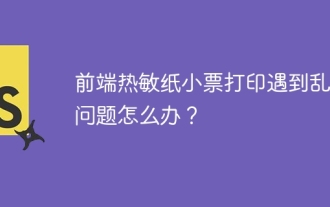
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
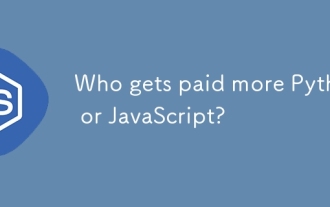
There is no absolute salary for Python and JavaScript developers, depending on skills and industry needs. 1. Python may be paid more in data science and machine learning. 2. JavaScript has great demand in front-end and full-stack development, and its salary is also considerable. 3. Influencing factors include experience, geographical location, company size and specific skills.
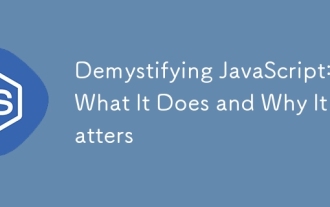
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
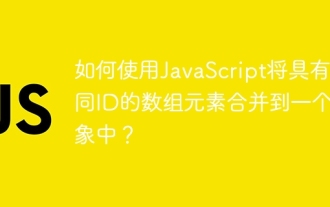
How to merge array elements with the same ID into one object in JavaScript? When processing data, we often encounter the need to have the same ID...
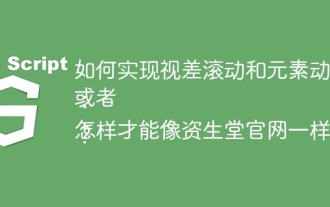
Discussion on the realization of parallax scrolling and element animation effects in this article will explore how to achieve similar to Shiseido official website (https://www.shiseido.co.jp/sb/wonderland/)...
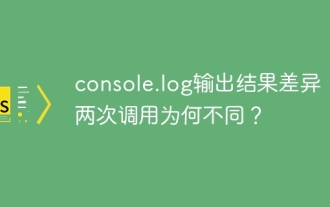
In-depth discussion of the root causes of the difference in console.log output. This article will analyze the differences in the output results of console.log function in a piece of code and explain the reasons behind it. �...
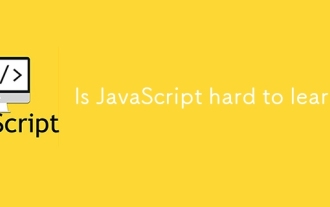
Learning JavaScript is not difficult, but it is challenging. 1) Understand basic concepts such as variables, data types, functions, etc. 2) Master asynchronous programming and implement it through event loops. 3) Use DOM operations and Promise to handle asynchronous requests. 4) Avoid common mistakes and use debugging techniques. 5) Optimize performance and follow best practices.
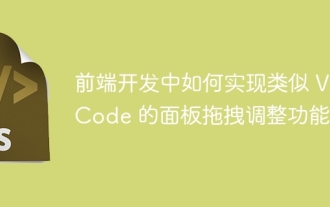
Explore the implementation of panel drag and drop adjustment function similar to VSCode in the front-end. In front-end development, how to implement VSCode similar to VSCode...
