Use Laravel Contracts to Build a Laravel 5 Twig Package
Feb 18, 2025 am 10:48 AMLaravel 5's Contracts: A Deep Dive into Architectural Improvements and Practical Application with Twig
Laravel 5 introduced a significant architectural enhancement: the Contracts package. This article explores the rationale behind this change and demonstrates a practical use case by replacing Laravel's Blade templating engine with Symfony Twig, leveraging the power of Contracts.
Key Concepts:
- Contracts as Interfaces: Laravel Contracts are interfaces defining specific behaviors. The IoC container binds these interfaces to concrete implementations, allowing for easy swapping of services without altering the interface's usage.
- Decoupling and Flexibility: This approach promotes loose coupling, making code more modular, testable, and adaptable to different service providers. Replacing Blade with Twig exemplifies this flexibility.
- Stable API: Contracts provide a stable API, ensuring consistent interaction with framework components, even when underlying implementations change.
Understanding Contracts:
A contract, in essence, is an interface specifying a behavior. This aligns with object-oriented programming principles where interfaces define method signatures without implementation details. Laravel's IoC container facilitates binding interfaces (contracts) to their implementations. For example:
$this->app->bind('App\Contracts\EventPusher', 'App\Services\PusherEventPusher');
Switching to a different service (e.g., Fanout) only requires changing the binding:
$this->app->bind('App\Contracts\EventPusher', 'App\Services\FanoutEventPusher');
Many core Laravel services now utilize contracts, enabling easy overriding. For instance, to replace the Illuminate/Mail
service, implement the IlluminateContractsMail
contract.
Replacing Blade with Twig using Contracts:
Laravel's default templating engine is Blade. This example demonstrates replacing it with Symfony Twig using Contracts.
1. Package Definition (composer.json):
{ "name": "whyounes/laravel5-twig", "description": "Twig for Laravel 5", "authors": [ { "name": "RAFIE Younes", "email": "younes.rafie@gmail.com" } ], "require": { "twig/twig": "1.18.*" }, "autoload": { "psr-0": { "RAFIE\": "src/" } } }
2. View Service Provider (TwigViewServiceProvider.php):
This provider registers the Twig loader and environment within Laravel's service container.
// ... (Provider code as in original example) ...
3. View Factory (TwigFactory.php):
This factory implements IlluminateContractsViewFactory
and handles view creation and rendering using Twig.
// ... (Factory code as in original example) ...
4. View Implementation (TwigView.php):
This class implements IlluminateContractsViewView
and acts as a container for the Twig view, interacting with the TwigFactory
.
// ... (View code as in original example) ...
5. Service Provider Registration (config/app.php):
Register the TwigViewServiceProvider
and comment out the default Laravel view provider:
$this->app->bind('App\Contracts\EventPusher', 'App\Services\PusherEventPusher');
6. Using Twig in Routes (app/Http/routes.php):
Now you can use Twig templates:
$this->app->bind('App\Contracts\EventPusher', 'App\Services\FanoutEventPusher');
7. Twig Template (resources/views/home.twig):
A simple Twig template:
{ "name": "whyounes/laravel5-twig", "description": "Twig for Laravel 5", "authors": [ { "name": "RAFIE Younes", "email": "younes.rafie@gmail.com" } ], "require": { "twig/twig": "1.18.*" }, "autoload": { "psr-0": { "RAFIE\": "src/" } } }
Conclusion:
Laravel 5's Contracts offer a powerful mechanism for extending and customizing the framework. Replacing Blade with Twig highlights the benefits of this architectural pattern, leading to cleaner, more testable, and flexible applications. The consistent API provided by Contracts simplifies the process and ensures maintainability. The complete project can be found at [link to project].
Frequently Asked Questions (FAQs):
(The FAQs section from the original input is already well-written and comprehensive. No changes are needed here.)
The above is the detailed content of Use Laravel Contracts to Build a Laravel 5 Twig Package. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
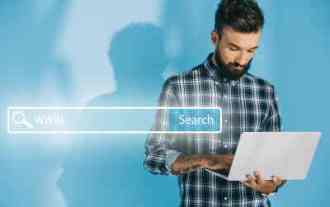
11 Best PHP URL Shortener Scripts (Free and Premium)
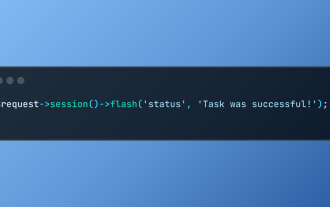
Working with Flash Session Data in Laravel
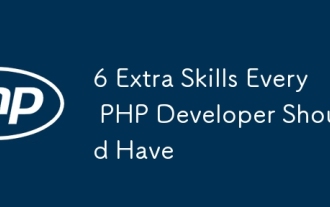
6 Extra Skills Every PHP Developer Should Have
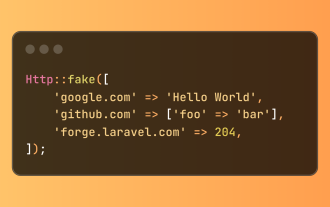
Simplified HTTP Response Mocking in Laravel Tests
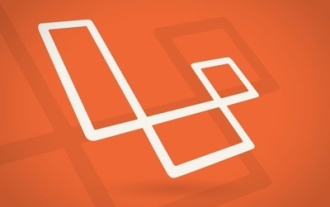
Build a React App With a Laravel Back End: Part 2, React
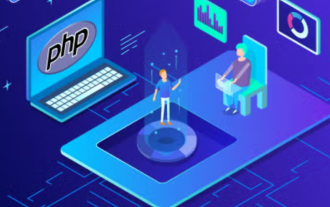
cURL in PHP: How to Use the PHP cURL Extension in REST APIs
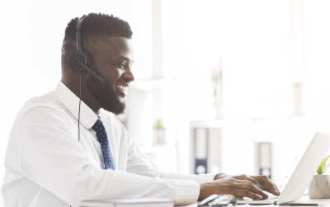
12 Best PHP Chat Scripts on CodeCanyon
