Getting Started with SQLite3: Basic Commands
SQLite: A Comprehensive Guide to Basic Commands
SQLite is a lightweight, serverless SQL database engine implemented as a C library. Unlike client-server databases like MySQL, SQLite operates directly from disk files, eliminating the need for server requests. This makes it ideal for embedded systems and applications requiring local data storage.
Key Features:
- Serverless: Direct disk access simplifies deployment and reduces overhead.
- Cross-platform: Portable database files work across various operating systems.
- Lightweight: Minimal resource consumption makes it suitable for resource-constrained environments.
- Standard SQL Compliance: Supports a significant subset of standard SQL commands.
Getting Started:
We'll use the sqlite3 command-line interface (CLI) for this tutorial. Installation instructions vary by operating system; consult the official SQLite documentation for your platform. For Debian-based systems (like Ubuntu), use:
sudo apt-get install sqlite3 libsqlite3-dev
After installation, launch the CLI by typing sqlite3
in your terminal. You'll see a prompt indicating you can type .help
for assistance.
Meta Commands:
Meta commands, prefixed with a dot (.), manage database settings and operations. .help
lists available meta commands. Useful commands include:
.show
: Displays current settings..databases
: Lists database names and files..quit
: Exits the sqlite3 program..tables
: Shows existing tables..schema
: Displays a table's schema..header ON/.header OFF
: Toggles header display in output..mode column
: Sets output mode to columns..dump
: Exports the database to SQL text format.
Standard SQL Commands:
Standard SQL commands interact with the database data. They are categorized as:
- Data Definition Language (DDL): Defines database structure.
CREATE TABLE
: Creates a new table.ALTER TABLE
: Modifies an existing table (adding or renaming columns).DROP TABLE
: Deletes a table.
- Data Manipulation Language (DML): Manipulates data within tables.
INSERT INTO
: Adds new rows.UPDATE
: Modifies existing rows.DELETE FROM
: Removes rows.
- Data Query Language (DQL): Retrieves data.
SELECT
: Queries data from tables.
Example: A Comment Section Database
Let's create a database for a website's comment section. The table will store: post_id
(auto-incrementing integer primary key), name
, email
, website_url
(nullable), and comment
.
-
Create the database:
sudo apt-get install sqlite3 libsqlite3-dev
Copy after loginCopy after login -
Create the table:
sqlite3 comment_section.db
Copy after login -
Insert data:
CREATE TABLE comments ( post_id INTEGER NOT NULL PRIMARY KEY AUTOINCREMENT, name TEXT NOT NULL, email TEXT NOT NULL, website_url TEXT NULL, comment TEXT NOT NULL );
Copy after login -
Select data (with headers and columnar output):
INSERT INTO comments (name, email, website_url, comment) VALUES ('John Doe', 'john.doe@example.com', 'johndoe.com', 'Great article!');
Copy after login -
Update data:
.headers ON .mode column SELECT * FROM comments;
Copy after login -
Delete data:
UPDATE comments SET email = 'updated@example.com' WHERE post_id = 1;
Copy after login -
Alter table (add a username column):
DELETE FROM comments WHERE post_id = 1;
Copy after login -
Drop table:
ALTER TABLE comments ADD COLUMN username TEXT;
Copy after loginConclusion:
SQLite's simplicity and efficiency make it a powerful tool for various applications. While this tutorial covers the basics, exploring advanced features and integrating SQLite with programming languages like PHP will significantly expand its utility. GUI tools like DB Browser for SQLite can simplify database management for those preferring a visual interface.
Frequently Asked Questions (FAQs):
-
SQLite vs. SQLite3: SQLite3 is a later version with performance improvements and enhanced features.
-
Creating a database:
sqlite3 mydatabase.db
-
Creating a table:
CREATE TABLE mytable (column1 type, column2 type, ...);
-
Inserting data:
INSERT INTO mytable (column1, column2, ...) VALUES (value1, value2, ...);
-
Updating data:
UPDATE mytable SET column1 = value WHERE condition;
-
Deleting data:
DELETE FROM mytable WHERE condition;
-
Selecting data:
SELECT * FROM mytable WHERE condition ORDER BY column;
-
WHERE clause: Filters results based on a condition.
-
ORDER BY clause: Sorts results by a specified column.
-
Closing a database:
.quit
in the sqlite3 CLI.
Remember to replace placeholders like
mydatabase.db
,mytable
,column1
, etc., with your actual database and table names.The above is the detailed content of Getting Started with SQLite3: Basic Commands. For more information, please follow other related articles on the PHP Chinese website!
-

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










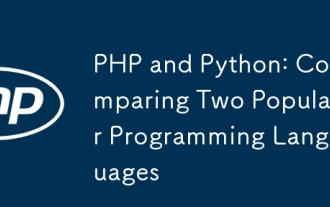
PHP and Python each have their own advantages, and choose according to project requirements. 1.PHP is suitable for web development, especially for rapid development and maintenance of websites. 2. Python is suitable for data science, machine learning and artificial intelligence, with concise syntax and suitable for beginners.
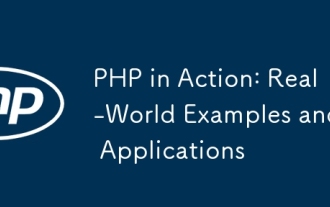
PHP is widely used in e-commerce, content management systems and API development. 1) E-commerce: used for shopping cart function and payment processing. 2) Content management system: used for dynamic content generation and user management. 3) API development: used for RESTful API development and API security. Through performance optimization and best practices, the efficiency and maintainability of PHP applications are improved.
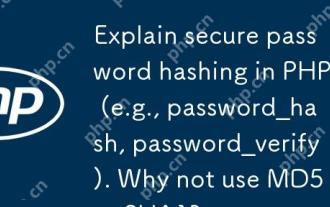
In PHP, password_hash and password_verify functions should be used to implement secure password hashing, and MD5 or SHA1 should not be used. 1) password_hash generates a hash containing salt values to enhance security. 2) Password_verify verify password and ensure security by comparing hash values. 3) MD5 and SHA1 are vulnerable and lack salt values, and are not suitable for modern password security.
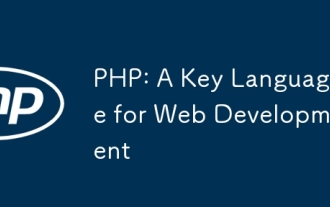
PHP is a scripting language widely used on the server side, especially suitable for web development. 1.PHP can embed HTML, process HTTP requests and responses, and supports a variety of databases. 2.PHP is used to generate dynamic web content, process form data, access databases, etc., with strong community support and open source resources. 3. PHP is an interpreted language, and the execution process includes lexical analysis, grammatical analysis, compilation and execution. 4.PHP can be combined with MySQL for advanced applications such as user registration systems. 5. When debugging PHP, you can use functions such as error_reporting() and var_dump(). 6. Optimize PHP code to use caching mechanisms, optimize database queries and use built-in functions. 7
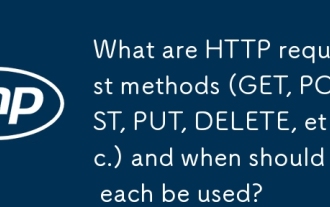
HTTP request methods include GET, POST, PUT and DELETE, which are used to obtain, submit, update and delete resources respectively. 1. The GET method is used to obtain resources and is suitable for read operations. 2. The POST method is used to submit data and is often used to create new resources. 3. The PUT method is used to update resources and is suitable for complete updates. 4. The DELETE method is used to delete resources and is suitable for deletion operations.
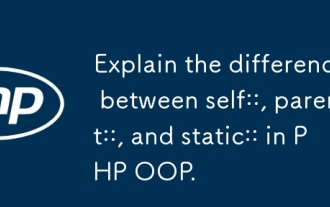
In PHPOOP, self:: refers to the current class, parent:: refers to the parent class, static:: is used for late static binding. 1.self:: is used for static method and constant calls, but does not support late static binding. 2.parent:: is used for subclasses to call parent class methods, and private methods cannot be accessed. 3.static:: supports late static binding, suitable for inheritance and polymorphism, but may affect the readability of the code.
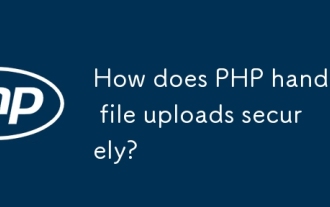
PHP handles file uploads through the $\_FILES variable. The methods to ensure security include: 1. Check upload errors, 2. Verify file type and size, 3. Prevent file overwriting, 4. Move files to a permanent storage location.
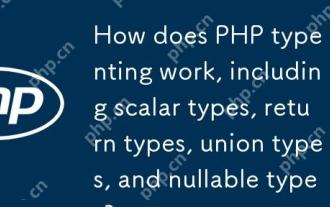
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.
