Integrating a CAPTCHA with the WordPress Registration Form
This tutorial demonstrates how to integrate Google's reCAPTCHA into a WordPress registration form to combat spam registrations. We'll leverage the WordPress HTTP API to verify user responses.
Key Concepts:
- reCAPTCHA: A Google service that distinguishes between humans and bots, preventing automated spam registrations.
- WordPress HTTP API: Used to communicate with the reCAPTCHA API for verification.
- Plugin Development: We'll build a custom plugin to handle reCAPTCHA integration.
Why Use reCAPTCHA?
WordPress's popularity makes it a prime target for bots creating numerous spam accounts. reCAPTCHA provides a robust solution to this problem.
Plugin Development Steps:
-
Obtain reCAPTCHA Keys: Register your domain on the reCAPTCHA website (reCAPTCHA v2 is recommended) and obtain your site key and secret key.
-
Plugin Header: Begin your plugin file (
recaptcha-registration.php
) with the standard plugin header:
<?php /** * Plugin Name: reCAPTCHA Registration * Plugin URI: [Your Plugin URI] * Description: Adds reCAPTCHA to the WordPress registration form. * Version: 1.0.0 * Author: [Your Name] * Author URI: [Your Website] * License: GPL2 * License URI: https://www.gnu.org/licenses/gpl-2.0.html * Text Domain: recaptcha-registration */
- PHP Class: Create a class to manage reCAPTCHA functionality:
class reCAPTCHA_Registration { private $site_key; private $secret_key; public function __construct() { $this->site_key = '[YOUR_SITE_KEY]'; // Replace with your site key $this->secret_key = '[YOUR_SECRET_KEY]'; // Replace with your secret key add_action('register_form', array($this, 'display_recaptcha')); add_action('registration_errors', array($this, 'validate_recaptcha'), 10, 3); } public function display_recaptcha() { ?> <🎜> <div class="g-recaptcha" data-sitekey="<?php echo $this->site_key; ?>" data-callback="recaptchaCallback"></div> <🎜> <input type="hidden" id="g-recaptcha-response" name="g-recaptcha-response"> <?php } public function validate_recaptcha($errors, $sanitized_user_login, $user_email) { $response = isset($_POST['g-recaptcha-response']) ? $_POST['g-recaptcha-response'] : null; if (empty($response)) { $errors->add('empty_recaptcha', __('Please complete the reCAPTCHA.', 'recaptcha-registration')); } else { $verify_response = $this->verify_recaptcha($response); if (!$verify_response['success']) { $errors->add('invalid_recaptcha', __('Invalid reCAPTCHA response.', 'recaptcha-registration')); } } } private function verify_recaptcha($response) { $url = 'https://www.google.com/recaptcha/api/siteverify'; $data = array( 'secret' => $this->secret_key, 'response' => $response, 'remoteip' => $_SERVER['REMOTE_ADDR'] ); $response = wp_remote_post($url, array('body' => $data)); return json_decode(wp_remote_retrieve_body($response), true); } } new reCAPTCHA_Registration();
-
Activate the Plugin: Upload
recaptcha-registration.php
to your/wp-content/plugins/
directory and activate it in your WordPress admin panel. Remember to replace the placeholder keys with your actual keys.
Screenshot of Protected Registration Form:
This improved version uses the newer reCAPTCHA v2 and asynchronous loading for better performance and user experience. It also includes error handling and internationalization. Remember to replace the bracketed placeholders with your actual reCAPTCHA keys. This code is more concise and efficient than the original example.
The above is the detailed content of Integrating a CAPTCHA with the WordPress Registration Form. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










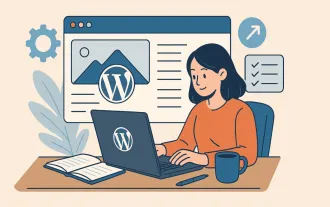
Blogs are the ideal platform for people to express their opinions, opinions and opinions online. Many newbies are eager to build their own website but are hesitant to worry about technical barriers or cost issues. However, as the platform continues to evolve to meet the capabilities and needs of beginners, it is now starting to become easier than ever. This article will guide you step by step how to build a WordPress blog, from theme selection to using plugins to improve security and performance, helping you create your own website easily. Choose a blog topic and direction Before purchasing a domain name or registering a host, it is best to identify the topics you plan to cover. Personal websites can revolve around travel, cooking, product reviews, music or any hobby that sparks your interests. Focusing on areas you are truly interested in can encourage continuous writing
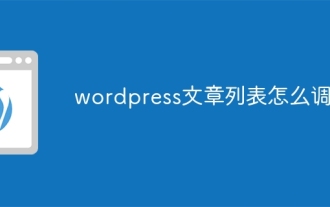
There are four ways to adjust the WordPress article list: use theme options, use plugins (such as Post Types Order, WP Post List, Boxy Stuff), use code (add settings in the functions.php file), or modify the WordPress database directly.
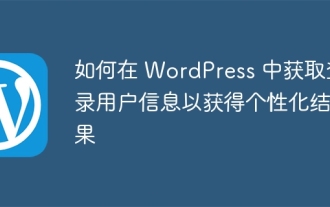
Recently, we showed you how to create a personalized experience for users by allowing users to save their favorite posts in a personalized library. You can take personalized results to another level by using their names in some places (i.e., welcome screens). Fortunately, WordPress makes it very easy to get information about logged in users. In this article, we will show you how to retrieve information related to the currently logged in user. We will use the get_currentuserinfo(); function. This can be used anywhere in the theme (header, footer, sidebar, page template, etc.). In order for it to work, the user must be logged in. So we need to use
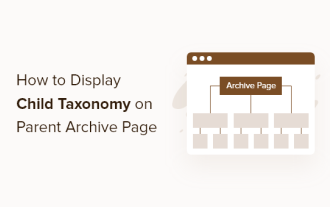
Do you want to know how to display child categories on the parent category archive page? When you customize a classification archive page, you may need to do this to make it more useful to your visitors. In this article, we will show you how to easily display child categories on the parent category archive page. Why do subcategories appear on parent category archive page? By displaying all child categories on the parent category archive page, you can make them less generic and more useful to visitors. For example, if you run a WordPress blog about books and have a taxonomy called "Theme", you can add sub-taxonomy such as "novel", "non-fiction" so that your readers can
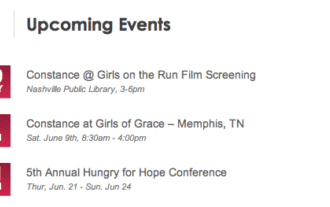
In the past, we have shared how to use the PostExpirator plugin to expire posts in WordPress. Well, when creating the activity list website, we found this plugin to be very useful. We can easily delete expired activity lists. Secondly, thanks to this plugin, it is also very easy to sort posts by post expiration date. In this article, we will show you how to sort posts by post expiration date in WordPress. Updated code to reflect changes in the plugin to change the custom field name. Thanks Tajim for letting us know in the comments. In our specific project, we use events as custom post types. Now
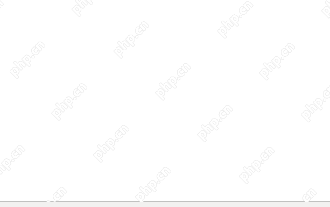
Are you looking for ways to automate your WordPress website and social media accounts? With automation, you will be able to automatically share your WordPress blog posts or updates on Facebook, Twitter, LinkedIn, Instagram and more. In this article, we will show you how to easily automate WordPress and social media using IFTTT, Zapier, and Uncanny Automator. Why Automate WordPress and Social Media? Automate your WordPre
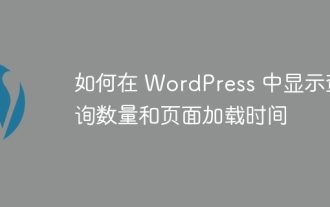
One of our users asked other websites how to display the number of queries and page loading time in the footer. You often see this in the footer of your website, and it may display something like: "64 queries in 1.248 seconds". In this article, we will show you how to display the number of queries and page loading time in WordPress. Just paste the following code anywhere you like in the theme file (e.g. footer.php). queriesin
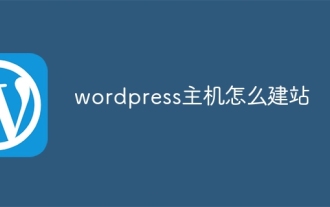
To build a website using WordPress hosting, you need to: select a reliable hosting provider. Buy a domain name. Set up a WordPress hosting account. Select a topic. Add pages and articles. Install the plug-in. Customize your website. Publish your website.
