How to Integrate jQuery Plugins into an Ember Application
Key Points
- Integrating jQuery plug-in into Ember applications can enhance its functionality and user experience by combining the simplicity and versatility of jQuery plug-in with the robustness and scalability of Ember.
- To integrate the jQuery plug-in into an Ember application, first install jQuery using the npm package manager, and then import the plug-in into the relevant Ember components.
- The initialization of the jQuery plug-in in the Ember component should be done within a special function named
didInsertElement
, usingthis.$
instead of$
to ensure that the plug-in is initialized only for this component and does not interfere with other components. - To avoid redundant event handlers, any events set using the plugin should be removed when the component is about to be removed from the DOM, using another Ember hook named
willDestroyElement
.
This article was reviewed by Craig Bilner. Thanks to all SitePoint peer reviewers for getting SitePoint content to its best!
Given the popularity of jQuery, it still plays a crucial role in the field of web development, especially when using frameworks such as Ember, it is no surprise that it is often used. The components of this framework are similar to jQuery plugins, as they are all designed to assume a single responsibility in a project. In this article, we will develop a simple Ember component. This tutorial shows how to integrate the jQuery plug-in into your Ember application. This component acts as a wrapper for the plugin, displaying a list of image thumbnails. Whenever we click on the thumbnail, its larger version will be displayed in the image previewer. This is done by extracting the src
attribute of the clicked thumbnail. We then set the previewer's src
property to the thumbnail's src
property. The complete code for this article can be found on GitHub. With this in mind, let's start this project.
Project Settings
First, let's create a new Ember project. First, execute the following command on the CLI:
npm install -g ember-cli
After completion, you can create the project by running the following command:
ember new emberjquery
This will create a new project in a folder called emberjquery
and install the required dependencies. Now, go to the directory by writing cd emberjquery
. This project contains different files that we will edit in this tutorial. The first file you have to edit is the bower.json
file. Open it and change the current Ember version to 2.1.0. The jQuery plugin I created for this project is available as a Bower package. You can include this line in your project by adding it to your bower.json
file:
"jquerypic": "https://github.com/LaminSanneh/sitepoint-jquerypic.git#faulty"
Now, to install the plug-in and a new version of Ember, run the following command:
npm install -g ember-cli
Since this plugin is not an Ember component, we need to include the required files manually. In the ember-cli-build.js
file, add the following two lines before the return
statement:
ember new emberjquery
These lines import two files and include them in the build. One is the plugin file itself, and the other is the plugin's CSS file. The stylesheet is optional, and you can exclude it if you plan to style the plugin yourself.
Create a new plug-in component
After including the plugin in the application, let's start creating a new component by executing the following command:
"jquerypic": "https://github.com/LaminSanneh/sitepoint-jquerypic.git#faulty"
This command creates a class file and a template file. In the template file, paste the contents from the bower_components/jquerypic/index.html
file. Place the content in the <body>
tag, excluding scripts. At this time, the template file should look like this:
bower install
In the class file, add a function named didInsertElement
:
// 要添加的行 app.import("bower_components/jquerypic/js/jquerypic.js"); app.import("bower_components/jquerypic/styles/app.css"); return app.toTree(); };
We are at a critical point now. With jQuery, plugin initialization usually occurs in the document.ready
function, as shown below:
ember generate component jquery-pic
. This function is called when the component is ready and has been successfully inserted into the DOM. By wrapping our code in this function, we can guarantee two things: didInsertElement
- Plugin is initialized only for this component
- Plugins will not interfere with other components
{{yield}} <div class="jquerypic"> <div class="fullversion-container"> <img class="full-version lazy" src="/static/imghw/default1.png" data-src="https://img.php.cn/" alt=""> </div> <div class="thumbnails"> <img class="thumbnail lazy" src="/static/imghw/default1.png" data-src="https://img.php.cn/" alt=""> <img class="thumbnail lazy" src="/static/imghw/default1.png" data-src="https://img.php.cn/" alt=""> <img class="thumbnail lazy" src="/static/imghw/default1.png" data-src="https://img.php.cn/" alt=""> <img class="thumbnail lazy" src="/static/imghw/default1.png" data-src="https://img.php.cn/" alt=""> <img class="thumbnail lazy" src="/static/imghw/default1.png" data-src="https://img.php.cn/" alt=""> </div> </div>
import Ember from 'ember'; export default Ember.Component.extend({ didInsertElement: function () { } });
$(document).ready(function(){ // 在这里初始化插件 });
(The following content is similar to the original text, but the sentences have been adjusted and polished and maintained the original meaning)
Ember component initializationTo show the problem, let's first add the following code to the
function: didInsertElement
npm install -g ember-cli
Without using Ember, this is how you usually initialize plugins. Now, check your browser window and click on the thumbnail. You should see them loading into the large image previewer as expected. Everything seems to work properly, right? OK, check out what happens when we add a second component instance. Do this by adding another line of code containing the same code I showed earlier into the index template. Therefore, your template should now look like this:
ember new emberjquery
If you switch to the browser window, you should see two component instances appear. When you click on the thumbnail of one of the instances, you can notice the error. The previewer for both instances will change, not just the instance that clicks. To resolve this issue, we need to change the initializer a little bit. The correct statement to use is as follows:
"jquerypic": "https://github.com/LaminSanneh/sitepoint-jquerypic.git#faulty"
The difference is that we are using this.$
instead of $
now. The two component instances should now work properly. Clicking on a thumbnail for one instance should not affect another component. When we use this.$
in a component, we refer to the jQuery handler that is only for that component. Therefore, any DOM operations we perform on it will only affect the component instance. Additionally, any event handler will be set only on this component. When we use the global jQuery property $
, we refer to the entire document. This is why our initialization affects another component. I have to modify my plugin to demonstrate this error, which may be the topic of a future article. However, the best practice for operating components' DOM is to use this.$
.
Destroy the plugin
OK, so far we've learned how to set up event handlers. Now it's time to show how to remove any events we set up with the plugin. We should do this when our components are about to be removed from the DOM. We should do this because we don't want any redundant event handlers to hang there. Fortunately, the Ember component provides another hook called willDestroyElement
. This hook is called every time Ember is about to be destroyed and the component instance is removed from the DOM. My plugin has a stopEvents
method that can be called on the plugin instance. This method should be called in a special hook provided by Ember. So add the following function to the component:
bower install
Modify the didInsertElement
function to look like this:
npm install -g ember-cli
In the didInsertElement
function, we just store the plugin instance in a property of the component. We do this so that we can access it in other functions. In the willDestroyElement
function, we are calling the stopEvents
method on the plugin instance. Although this is a best practice, our application cannot trigger this hook. Therefore, we will set up a demo click handler. In this handler, we will call the stopEvents
method on the plugin instance. This allows me to show that all event handlers have been removed as expected. Now, let's add a click function handler to the component:
ember new emberjquery
Then add a <code><p>
tag to the component template, as shown below:
"jquerypic": "https://github.com/LaminSanneh/sitepoint-jquerypic.git#faulty"
When clicking on this tab, it calls the stopEvents
operation of destroying the plugin. After clicking on a paragraph, the plugin should no longer respond to the click event. To enable events again, you must initialize the plugin as we did in the didInsert
hook. Through the last section, we complete the simple Ember component. Congratulations!
(The conclusion and FAQ parts are similar to the original text, except that the sentences are adjusted and polished and maintained the original meaning)
Conclusion
In this tutorial, you have seen that jQuery plugin still plays a crucial role in our careers. With its powerful API and available JavaScript framework, it is very useful to understand how to combine these two worlds and make them work in harmony. In our example, the component acts as a wrapper for the plugin, displaying a list of image thumbnails. Whenever we click on the thumbnail, its larger version will be displayed in the image previewer. This is just an example, but you can integrate any required jQuery plugin. Again, the code is available on GitHub. Are you using the jQuery plugin in your Ember app? If you want to discuss them, feel free to comment in the section below.
FAQs about integrating jQuery plug-in into Ember applications
What is the purpose of integrating jQuery plug-in into an Ember application?
Integrating jQuery plug-in into Ember applications can significantly enhance the functionality and user experience of your application. The jQuery plugin provides various features such as animations, form validation, and interactive elements that can be easily integrated into Ember applications. This integration allows developers to take advantage of the power of jQuery and Ember to combine the simplicity and versatility of jQuery plug-ins with the robustness and scalability of Ember.
How to install jQuery in my Ember application?
To install jQuery in the Ember application, you can use the npm package manager. Run the command npm install --save @ember/jquery
in your terminal. This adds jQuery to the project's dependencies and makes it workable in the application.
How to use jQuery plugin in my Ember application?
After you install jQuery, you can use the jQuery plug-in in your Ember application by importing it into the relevant Ember component. You can then use the plugin's functions according to the plugin's documentation. Remember to make sure the plugin is compatible with the version of jQuery you are using.
Is there any compatibility issue between jQuery and Ember?
Often, jQuery and Ember work well together. However, depending on the version of jQuery and Ember you are using, there may be some compatibility issues. Always check the documentation for jQuery and Ember for compatibility.
Can I use the jQuery plugin in Ember without installing jQuery?
No, you cannot use the jQuery plugin in Ember without installing jQuery. The jQuery plugin is built on top of the jQuery library and requires it to run. Therefore, you must first install jQuery before you can use any jQuery plugin.
How to update jQuery in my Ember application?
To update jQuery in the Ember application, you can use the npm package manager. Run the command npm update @ember/jquery
in your terminal. This will update jQuery to the latest version.
Can I use multiple jQuery plugins in my Ember application?
Yes, you can use multiple jQuery plugins in your Ember application. However, be aware that using too many plugins can affect the performance of your application. Always thoroughly test your application after adding a new plugin to make sure it still performs as expected.
How to troubleshoot jQuery plug-in issues in Ember applications?
If you encounter jQuery plugin issues in your Ember application, first check the plugin's documentation for any known issues or troubleshooting tips. If you are still having problems, try contacting the plugin developer or the Ember community for help.
Can I use the jQuery plugin in my Ember application without any programming experience?
While you can use the jQuery plugin in your Ember application without any programming experience, it is recommended that you understand the basics of JavaScript and Ember. This will make it easier for you to understand how to integrate and use plugins efficiently.
Is there an alternative to using the jQuery plugin in my Ember application?
Yes, there are alternatives to using jQuery plugin in Ember applications. Ember itself has a rich ecosystem of add-ons that can provide similar features to jQuery plugins. Additionally, you can use native JavaScript or other JavaScript libraries to achieve the same results. However, jQuery plugins usually provide simpler and more convenient solutions.
The above is the detailed content of How to Integrate jQuery Plugins into an Ember Application. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


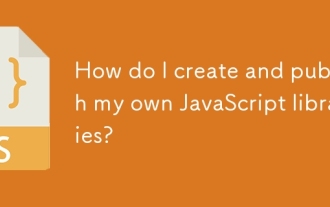
Article discusses creating, publishing, and maintaining JavaScript libraries, focusing on planning, development, testing, documentation, and promotion strategies.
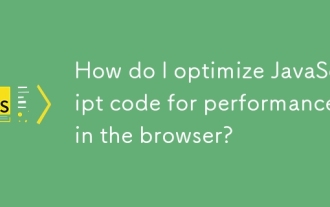
The article discusses strategies for optimizing JavaScript performance in browsers, focusing on reducing execution time and minimizing impact on page load speed.
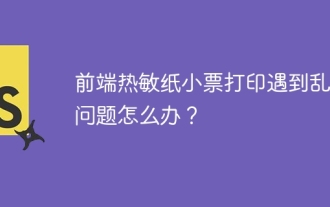
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
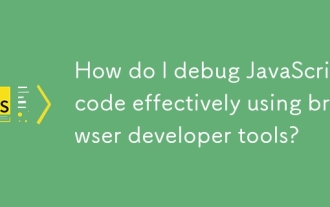
The article discusses effective JavaScript debugging using browser developer tools, focusing on setting breakpoints, using the console, and analyzing performance.
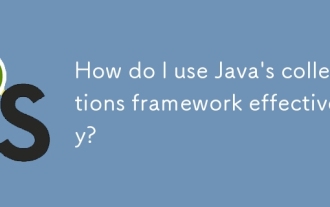
This article explores effective use of Java's Collections Framework. It emphasizes choosing appropriate collections (List, Set, Map, Queue) based on data structure, performance needs, and thread safety. Optimizing collection usage through efficient
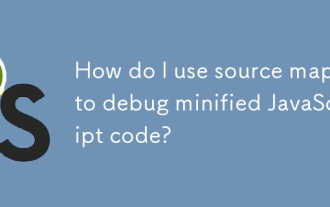
The article explains how to use source maps to debug minified JavaScript by mapping it back to the original code. It discusses enabling source maps, setting breakpoints, and using tools like Chrome DevTools and Webpack.

This tutorial will explain how to create pie, ring, and bubble charts using Chart.js. Previously, we have learned four chart types of Chart.js: line chart and bar chart (tutorial 2), as well as radar chart and polar region chart (tutorial 3). Create pie and ring charts Pie charts and ring charts are ideal for showing the proportions of a whole that is divided into different parts. For example, a pie chart can be used to show the percentage of male lions, female lions and young lions in a safari, or the percentage of votes that different candidates receive in the election. Pie charts are only suitable for comparing single parameters or datasets. It should be noted that the pie chart cannot draw entities with zero value because the angle of the fan in the pie chart depends on the numerical size of the data point. This means any entity with zero proportion
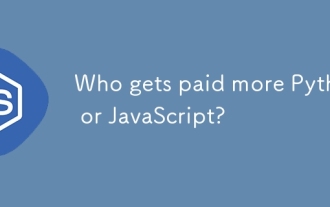
There is no absolute salary for Python and JavaScript developers, depending on skills and industry needs. 1. Python may be paid more in data science and machine learning. 2. JavaScript has great demand in front-end and full-stack development, and its salary is also considerable. 3. Influencing factors include experience, geographical location, company size and specific skills.
