Create a Real-Time Video Chat Room with WebRTC & Twilio
This article benefited from peer review by Wern Ancheta and Tim Severien. Thanks to SitePoint’s peer reviewers for enhancing our content!
Building on my previous article, "The Dawn of WebRTC," which demonstrated a simple photo booth app, this article guides you through creating a functional video chat room using the Web Real-Time Communications (WebRTC) API.
WebRTC empowers web and mobile developers to build high-definition video and audio calling applications with straightforward APIs. A wide range of industries, including healthcare, education, customer service, and social media, are leveraging WebRTC for next-generation applications. You've likely used WebRTC unknowingly through platforms like Facebook Messenger, WhatsApp, and Snapchat.
Key Learning Points:
- Integrate real-time video and audio into your applications using Twilio's Programmable Video API, improving user engagement.
- Set up a video chat room with Twilio and Firebase for user management.
- Ensure compatibility with WebRTC-supported browsers (Chrome, Firefox, Opera) and implement SSL encryption for secure communication.
- Utilize provided PHP and JavaScript code for user authentication, chat invitations, and connection management.
- Develop a robust real-time video chat application with user status updates and dynamic connection/disconnection capabilities.
Streamlining Development with Twilio
WebRTC and similar technologies are revolutionizing communication. Developers can easily integrate enhanced communication features into any application. Just as major platforms like Facebook, Snapchat, Tango, and WhatsApp have incorporated live audio and video, so can you.
The process is surprisingly simple, fast, and cost-effective. Google's open-source nature of WebRTC eliminates licensing fees. However, navigating WebRTC components like TURN/STUN, signaling, and MCUs can be challenging.
Many PaaS providers offer WebRTC solutions. Based on our experience at Blacc Spot Media, we recommend Twilio for its proven effectiveness. This article focuses on their platform.
Twilio Video: A Powerful Tool
Twilio offers a suite of communication tools via simple APIs and SDKs. Their Programmable Video allows for HD multi-party video and audio experiences in web and mobile apps. Twilio is a leader in the WebRTC space, continuously enhancing its offerings. Future enhancements include mobile screen sharing and improved multi-party capabilities.
Building the Chat Room
This demo requires a Twilio account (sign up for a free account and select "Programmable Video"). You'll need:
Credential | Description |
---|---|
Twilio Account SID | Your main Twilio account identifier (found on your dashboard). |
Twilio Video Config SID | Enables video capabilities in the access token (generate one on your dashboard). |
API Key | Used for authentication (generate one on your dashboard). |
API Secret | Used for authentication (generate one on your dashboard). |
We'll also use Firebase for user management. (Sign up for a free account if needed). After setup, you can deploy this demo to a server.
The Demo
The code is available on GitHub, and a live demo is hosted at Blacc Spot Media. Remember that WebRTC currently supports Google Chrome, Mozilla Firefox, and Opera on desktop. Check browser compatibility at Can I Use rtcpeerconnection?
For server deployment (Chrome 47 and later require SSL), use Let's Encrypt for a free SSL certificate. A Digital Ocean tutorial can assist with installation.
PHP Code (token.php)
This PHP script handles Twilio authentication and token generation.
// ADD TWILIO REQUIRED LIBRARIES require_once('twilio/Services/Twilio.php'); // TWILIO CREDENTIALS $TWILIO_ACCOUNT_SID = 'your account sid here'; $TWILIO_CONFIGURATION_SID = 'your configuration sid here'; $TWILIO_API_KEY = 'your API key here'; $TWILIO_API_SECRET = 'your API secret here'; // CREATE TWILIO TOKEN $id = $_GET['id']; $token = new Services_Twilio_AccessToken( $TWILIO_ACCOUNT_SID, $TWILIO_API_KEY, $TWILIO_API_SECRET, 3600, $id ); $grant = new Services_Twilio_Auth_ConversationsGrant(); $grant->setConfigurationProfileSid($TWILIO_CONFIGURATION_SID); $token->addGrant($grant); echo json_encode(array( 'id' => $id, 'token' => $token->toJWT(), ));
HTML Code (index.html)
This HTML provides the basic structure for the chat room interface.
<div class="m-content"> <h1 id="Quick-Start-Your-WebRTC-Project-with-Twilio">Quick Start Your WebRTC Project with Twilio</h1> <div class="start"> <input type="text" id="id" name="id" value="" placeholder="Enter your name to join the chat" /> <button id="start">Join Chat Room</button> <button id="disconnect" class="b-disconnect hide">Disconnect from Chat</button> <div class="status"> <strong>MY STATUS:</strong> <span id="status">DISCONNECTED</span> </div> </div> <div class="local"> <div id="lstream"></div> </div> <div class="remote"> <div id="rstream"></div> </div> <div class="users-list"></div> <div class="logs"></div> </div> <🎜> <🎜> <🎜> <🎜> <🎜>
JavaScript Code (app.js)
This JavaScript handles WebRTC functionality, user interaction, and Firebase integration. (Note: This is a significantly shortened version for brevity. The full code is available on GitHub.)
// ... (WebRTC browser check, tlog function, etc.) ... $('#start').on('click', function() { // ... (Ajax request to token.php, Twilio client setup) ... }); // ... (clientConnected, firebaseConnect, addParticipant functions) ... function startConversation() { // ... (Get user media, attach to #lstream) ... } // ... (conversationInvite, conversationStarted, participantConnected, participantDisconnected functions) ... $('#disconnect').on('click', function() { // ... (Firebase disconnect, stop conversation, reset UI) ... }); // ... (stopConversation function) ...
The complete JavaScript code, including the omitted functions, is available on the GitHub repository linked in the original article.
Conclusion
WebRTC is transforming communication. Twilio and Firebase simplify the development of real-time communication applications. Start building your own innovative solutions today! For more WebRTC tutorials and resources, visit webrtc.tutorials (when launched).
(The FAQs section from the original input has been omitted due to length constraints, but it can be easily re-integrated into this revised output.)
The above is the detailed content of Create a Real-Time Video Chat Room with WebRTC & Twilio. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


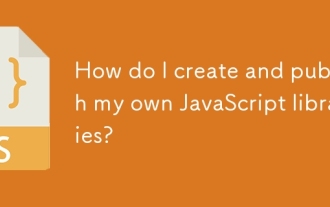
Article discusses creating, publishing, and maintaining JavaScript libraries, focusing on planning, development, testing, documentation, and promotion strategies.
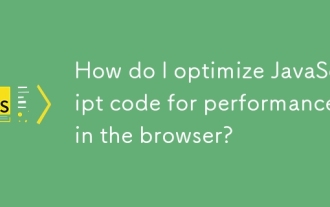
The article discusses strategies for optimizing JavaScript performance in browsers, focusing on reducing execution time and minimizing impact on page load speed.
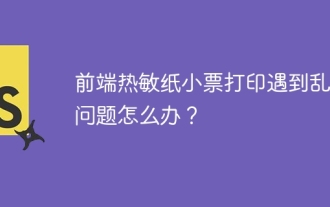
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
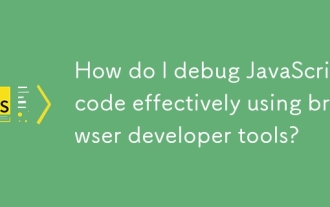
The article discusses effective JavaScript debugging using browser developer tools, focusing on setting breakpoints, using the console, and analyzing performance.
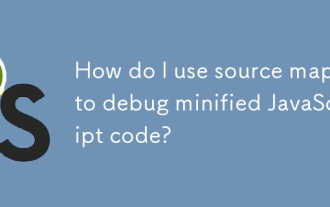
The article explains how to use source maps to debug minified JavaScript by mapping it back to the original code. It discusses enabling source maps, setting breakpoints, and using tools like Chrome DevTools and Webpack.
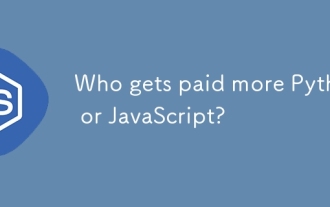
There is no absolute salary for Python and JavaScript developers, depending on skills and industry needs. 1. Python may be paid more in data science and machine learning. 2. JavaScript has great demand in front-end and full-stack development, and its salary is also considerable. 3. Influencing factors include experience, geographical location, company size and specific skills.

This tutorial will explain how to create pie, ring, and bubble charts using Chart.js. Previously, we have learned four chart types of Chart.js: line chart and bar chart (tutorial 2), as well as radar chart and polar region chart (tutorial 3). Create pie and ring charts Pie charts and ring charts are ideal for showing the proportions of a whole that is divided into different parts. For example, a pie chart can be used to show the percentage of male lions, female lions and young lions in a safari, or the percentage of votes that different candidates receive in the election. Pie charts are only suitable for comparing single parameters or datasets. It should be noted that the pie chart cannot draw entities with zero value because the angle of the fan in the pie chart depends on the numerical size of the data point. This means any entity with zero proportion
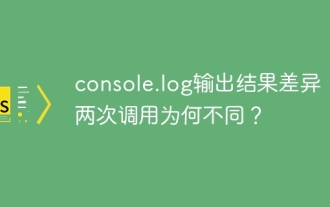
In-depth discussion of the root causes of the difference in console.log output. This article will analyze the differences in the output results of console.log function in a piece of code and explain the reasons behind it. �...
