Practical OOP: Building a Quiz App - Bootstrapping
This article details building a maintainable and extensible quiz application using PHP, focusing on SOLID principles and the Domain Model and Service Layer patterns. My previous approach to PHP MVC development lacked a true understanding of cohesive application structure and concern separation. This series rectifies that.
Key Concepts:
- SOLID Principles & MVC: The article emphasizes the limitations of MVC alone and advocates for a deeper understanding and application of SOLID principles for robust object-oriented programming.
- Layered Architecture: The quiz app is structured in layers (domain model, service layer, presentation layer) for easy component substitution (e.g., database, UI).
- Domain Model Pattern: The database schema mirrors the object-oriented design, prioritizing clean code over direct database mirroring.
- Service Layer Pattern: This pattern encapsulates business logic, decoupling operations from their underlying classes, enabling reusability across different interfaces (web, CLI).
- Practical Implementation: The article provides a step-by-step guide to setting up the application, including coding the service class, defining its interface, and modeling quizzes and questions as entities. A placeholder mapper simplifies initial database interaction.
Why MVC Isn't Sufficient:
While MVC is valuable, its overuse often results in poorly structured, quasi-object-oriented or procedural code disguised within classes. This project uses the Domain Model pattern (from Martin Fowler's Patterns of Enterprise Application Architecture) to create a truly object-oriented system. Object-Relational Mapping (ORM) is acknowledged but deferred, using a manual, simplified mapper for this tutorial. The Service Layer addresses the challenge of coordinating multiple classes for complex operations.
The Service Layer:
Decoupled code, a cornerstone of good object-oriented design, requires a mechanism to combine independent classes. The Service Layer achieves this by grouping system operations into dedicated service classes, promoting reusability across different application parts (web, CLI, etc.).
Project Setup (using Slim):
Slim, a lightweight framework, is used for simplicity. The composer.json
file is provided for dependency management:
{ "require": { "slim/slim": "2.*" }, "autoload": { "psr-4": {"QuizApp\": "./lib/"} } }
Quiz Service Interface (QuizInterface.php
):
This interface defines the core quiz operations:
<?php namespace QuizApp\Service; interface QuizInterface { /** @return Quiz[] */ public function showAllQuizes(); public function startQuiz($quizOrId); /** @return Question */ public function getQuestion(); /** @return bool */ public function checkSolution($id); /** @return bool */ public function isOver(); /** @return Result */ public function getResult(); }
Quiz Mapper Interface (QuizMapperInterface.php
):
The mapper interface handles database interactions:
{ "require": { "slim/slim": "2.*" }, "autoload": { "psr-4": {"QuizApp\": "./lib/"} } }
Entities (Question.php
and Quiz.php
):
These classes model quiz questions and quizzes themselves:
<?php namespace QuizApp\Service; interface QuizInterface { /** @return Quiz[] */ public function showAllQuizes(); public function startQuiz($quizOrId); /** @return Question */ public function getQuestion(); /** @return bool */ public function checkSolution($id); /** @return bool */ public function isOver(); /** @return Result */ public function getResult(); }
Placeholder Mapper (Hardcoded.php
):
A temporary mapper with hardcoded data for testing:
<?php namespace QuizApp\Mapper; interface QuizInterface { /** @return \QuizApp\Entity\Quiz[] */ public function findAll(); /** @param int $i @return \QuizApp\Entity\Quiz */ public function find($i); }
Conclusion and Next Steps:
This initial part sets the foundation. The next part will involve implementing the service class, creating a real database mapper (likely using Doctrine), and developing the controllers and views. The modular design ensures maintainability and extensibility. The full source code for this part is [link to source code].
(FAQs section removed for brevity. The FAQs are well-written and could be included in a separate, follow-up article.)
The above is the detailed content of Practical OOP: Building a Quiz App - Bootstrapping. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










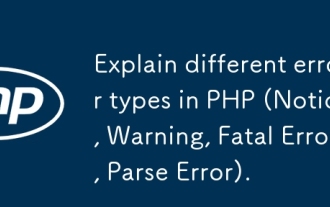
There are four main error types in PHP: 1.Notice: the slightest, will not interrupt the program, such as accessing undefined variables; 2. Warning: serious than Notice, will not terminate the program, such as containing no files; 3. FatalError: the most serious, will terminate the program, such as calling no function; 4. ParseError: syntax error, will prevent the program from being executed, such as forgetting to add the end tag.
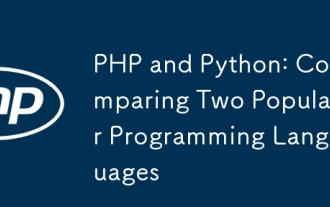
PHP and Python each have their own advantages, and choose according to project requirements. 1.PHP is suitable for web development, especially for rapid development and maintenance of websites. 2. Python is suitable for data science, machine learning and artificial intelligence, with concise syntax and suitable for beginners.
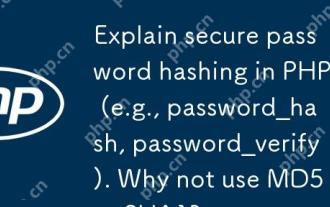
In PHP, password_hash and password_verify functions should be used to implement secure password hashing, and MD5 or SHA1 should not be used. 1) password_hash generates a hash containing salt values to enhance security. 2) Password_verify verify password and ensure security by comparing hash values. 3) MD5 and SHA1 are vulnerable and lack salt values, and are not suitable for modern password security.
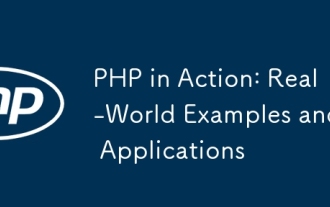
PHP is widely used in e-commerce, content management systems and API development. 1) E-commerce: used for shopping cart function and payment processing. 2) Content management system: used for dynamic content generation and user management. 3) API development: used for RESTful API development and API security. Through performance optimization and best practices, the efficiency and maintainability of PHP applications are improved.
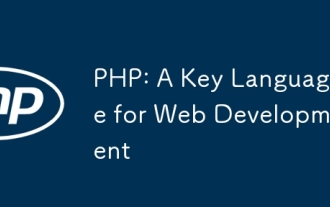
PHP is a scripting language widely used on the server side, especially suitable for web development. 1.PHP can embed HTML, process HTTP requests and responses, and supports a variety of databases. 2.PHP is used to generate dynamic web content, process form data, access databases, etc., with strong community support and open source resources. 3. PHP is an interpreted language, and the execution process includes lexical analysis, grammatical analysis, compilation and execution. 4.PHP can be combined with MySQL for advanced applications such as user registration systems. 5. When debugging PHP, you can use functions such as error_reporting() and var_dump(). 6. Optimize PHP code to use caching mechanisms, optimize database queries and use built-in functions. 7
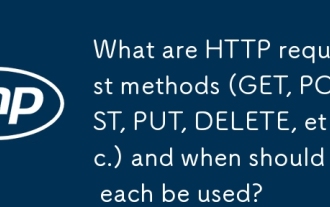
HTTP request methods include GET, POST, PUT and DELETE, which are used to obtain, submit, update and delete resources respectively. 1. The GET method is used to obtain resources and is suitable for read operations. 2. The POST method is used to submit data and is often used to create new resources. 3. The PUT method is used to update resources and is suitable for complete updates. 4. The DELETE method is used to delete resources and is suitable for deletion operations.
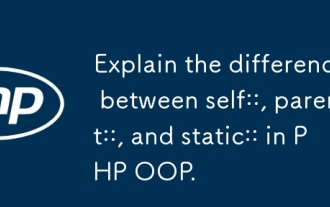
In PHPOOP, self:: refers to the current class, parent:: refers to the parent class, static:: is used for late static binding. 1.self:: is used for static method and constant calls, but does not support late static binding. 2.parent:: is used for subclasses to call parent class methods, and private methods cannot be accessed. 3.static:: supports late static binding, suitable for inheritance and polymorphism, but may affect the readability of the code.
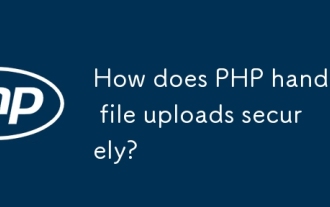
PHP handles file uploads through the $\_FILES variable. The methods to ensure security include: 1. Check upload errors, 2. Verify file type and size, 3. Prevent file overwriting, 4. Move files to a permanent storage location.
