Retro Revolution: Building a Pong Clone in Unity
Unity Pong cloning tutorial: build classic games step by step
Before you start, you can view the game on itch.io
Core points
- Building a Pong clone in Unity includes several key steps, including setting up the game environment, adding player input, managing balls and border collisions, implementing enemy AI, generating balls, and adding basic text instructions.
- Game environment settings include creating a new 2D project in Unity, setting the aspect ratio of the game screen to 4:3, and creating folders for scripts, sprites, prefabs, and materials. You need to download the sprite and add it to the sprite folder and adjust the units per pixel to ensure a clear and clean appearance.
- Player input is added through a C# script called "PlayerController", which sets the player's speed and range of player movement. Add Box Collider 2D to the Player GameObject to handle collisions.
- Ball and border collisions are managed by creating a Physics2D material called "Bounce", with elasticity set to 1 and friction set to 0. Add Circle Collider 2D and Rigidbody 2D to the Ball game object to manage its interaction with the environment.
- Enemy AI is implemented through a C# script called "EnemyController", which sets the enemy's speed and manages movements in response to the position of the ball. The enemy's scope is also set in this script.
- The ball generation is done by creating a "BallSpawner" game object and a C# script called "BallSpawnerController". This script checks for the presence of a ball and, if not, creates a new ball.
Pong game analysis
Pong is one of the earliest video games and the first successful commercial game. When Pong was first created, it's very likely that developers had a hard time with code logic, but nowadays, you can make a simple two-player Pong game with a method call, collider, and sprite. Once you decide to create a single player Pong game, the difficulty of making Pong will increase. In this tutorial, we will create the basic gameplay of Pong and break down a very simple AI alternative that still adds to the game's value.
We must ask, what are the core elements of Pong gameplay? Here is a list of answers to this question:
- Player Input – We want players to be able to move their racket up and down to make a hit.
- Ball Collision – When the ball hits a racket or boundary, it is not allowed to lose any speed.
- Border Collision – The ball must be able to bounce from the top and bottom of the screen so that it does not leave the game area.
- Enemy AI – If the enemy sits on the other end of the screen and does not move, the game's playability is almost zero.
- Generate Ball – When the ball hits one of the boundaries behind the racket, we need it to be regenerated so that we can continue the game.
- Ball-to-racquet collision area detection – This allows the ball to bounce off the racket at a unique angle so that we can better aim the ball when hitting the racket with it.
With this list, we can start writing game programs.
Please note that any number related to the position, rotation, zoom, etc. of the game object are relative and may need to be changed according to your specific settings.
Set the game
Now that we have analyzed the basic principles of Pong, we can start setting up the game. Open Unity and create a new 2D project. Once the editor is opened, set the aspect ratio of the game screen to 4:3. We use 4:3 because this is one of the most common screen ratios and is one of the closest to the standard ratios. In the Resources panel, create four folders called Scripts, Sprites, Prefabs, and Materials. These folders will be used to save all our game resources.
Download the required image of the game and add it to the "Sprite" folder (can be done with drag and drop). The image we just added will be the sprite (interactive game object) used in the game.
We need to change the per-pixel units of the sprite so that they meet the standards. I usually use 64 pixels per pixel unit, as this will make most sprites look clear and clean and keep their relative size. You can think of per-pixel units as pixel density allocated in 1×1 space in the Unity editor.
Let's set the square's units per pixel to 64 and the circle's units per pixel to 128. We can continue to add these three images to the Hierarchy panel.
Now we need to name each resource and set their initial properties and labels. You can name the blue block "Player" and set the player's x position to 6 and its x-scaling ratio to 0.2.
We need to create a tag to separate the racket game object from the other game objects. Broadly speaking, you can think of tags as categories of game objects. Click Untagged (under the player name) and select Add Tag. Create a new tag called "Paddle", reselect the player game object and set its tag to Paddle.
Name the red block "Enemy". Set the enemy's x position to -6 and its x-scaling ratio to 0.2. Make the enemy game object label Paddle.
Name the gray circle "Ball" and create a new label called "Ball". Make sure to set the label of the Ball object to Ball.
(The following steps are only provided with an overview of the steps and key code snippets due to space limitations. Please refer to the original text or supplement it yourself in detail)
Add player input
Create a C# script called "PlayerController" and add the following code (controls the movement of the player's racket):
public float speed = 10; public float topBound = 4.5F; public float bottomBound = -4.5F; void FixedUpdate () { float movementSpeedY = speed * Input.GetAxis("Vertical") * Time.deltaTime; transform.Translate(0, movementSpeedY, 0); // ... (边界限制代码) }
Ball collision
Create a Physics2D material called "Bounce", set its elasticity to 1 and friction to 0. Add Circle Collider 2D and Rigidbody 2D to the Ball game object and set the material to "Bounce".
Border collision
Create four empty game objects as boundaries (LeftBound, RightBound, TopBound, BottomBound), add Box Collider 2D and set its properties. Create a script called "BoundController" that detects the ball colliding with the boundary and destroys the ball.
Enemy AI
Create a script called "EnemyController" that controls the movement of the enemy's racket so that it follows the ball.
public float speed = 1.75F; Transform ball; Rigidbody2D ballRig2D; void FixedUpdate () { ball = GameObject.FindGameObjectWithTag("Ball").transform; ballRig2D = ball.GetComponent<Rigidbody2D>(); // ... (根据球的位置移动敌人的代码) }
Generate ball
Create an empty game object "BallSpawner" and create a script called "BallSpawnerController" to regenerate the ball when it disappears.
Add basic text
Create a UI Text object to display the game description.
Conclusion
You have now successfully created a basic single-player Pong clone in Unity2D. For more practice, try to think about ways to improve the game—for example, adding acceleration to the ball (the more hits the ball, the faster the ball is), adding inertia to the racket, adding difficulty levels by increasing the speed of the enemy, and so on .
(The FAQ part is omitted here due to the length of the article. The original text has included detailed FAQ answers)
The above is the detailed content of Retro Revolution: Building a Pong Clone in Unity. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


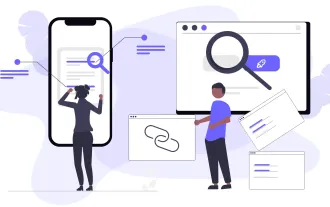
Website construction is just the first step: the importance of SEO and backlinks Building a website is just the first step to converting it into a valuable marketing asset. You need to do SEO optimization to improve the visibility of your website in search engines and attract potential customers. Backlinks are the key to improving your website rankings, and it shows Google and other search engines the authority and credibility of your website. Not all backlinks are beneficial: Identify and avoid harmful links Not all backlinks are beneficial. Harmful links can harm your ranking. Excellent free backlink checking tool monitors the source of links to your website and reminds you of harmful links. In addition, you can also analyze your competitors’ link strategies and learn from them. Free backlink checking tool: Your SEO intelligence officer
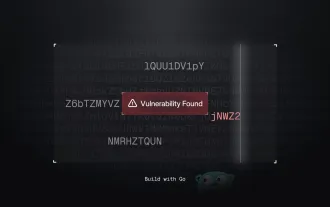
This Go-based network vulnerability scanner efficiently identifies potential security weaknesses. It leverages Go's concurrency features for speed and includes service detection and vulnerability matching. Let's explore its capabilities and ethical
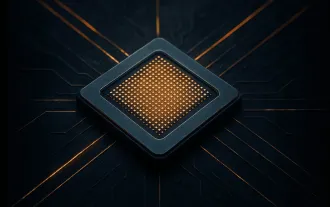
This pilot program, a collaboration between the CNCF (Cloud Native Computing Foundation), Ampere Computing, Equinix Metal, and Actuated, streamlines arm64 CI/CD for CNCF GitHub projects. The initiative addresses security concerns and performance lim
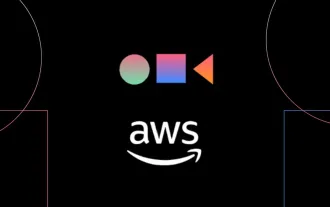
This tutorial guides you through building a serverless image processing pipeline using AWS services. We'll create a Next.js frontend deployed on an ECS Fargate cluster, interacting with an API Gateway, Lambda functions, S3 buckets, and DynamoDB. Th
