Build your own PHP Framework with Symfony Components
Feb 19, 2025 am 10:00 AMThis tutorial demonstrates building a minimal PHP framework using Symfony components. While not exhaustive, it covers the core elements for a functional application. For deeper dives, consult the official Symfony documentation.
Key Concepts:
This tutorial leverages Symfony components to build a flexible framework. It uses HttpFoundation for managing HTTP requests and responses, replacing standard PHP globals. The Routing component enables dynamic URL handling, and the EventDispatcher component facilitates modularity and extensibility via the Observer pattern. Finally, the HttpKernel component streamlines request processing and response generation.
Project Setup:
Begin with a basic index.php
file and install necessary components using Composer:
php composer.phar require symfony/http-foundation symfony/http-kernel symfony/routing symfony/event-dispatcher
HttpFoundation:
HttpFoundation provides Request
and Response
classes. Initially, index.php
might look like this (using globals):
switch($_SERVER['PATH_INFO']) { case '/': echo 'Home'; break; case '/about': echo 'About'; break; default: echo 'Not Found!'; }
This is improved by using HttpFoundation:
require 'vendor/autoload.php'; use Symfony\Component\HttpFoundation\Request; use Symfony\Component\HttpFoundation\Response; $request = Request::createFromGlobals(); $response = new Response(); switch ($request->getPathInfo()) { case '/': $response->setContent('Home'); break; case '/about': $response->setContent('About'); break; default: $response->setContent('Not Found!')->setStatusCode(Response::HTTP_NOT_FOUND); } $response->send();
HttpKernel:
To encapsulate framework logic, create a Core
class (e.g., lib/Framework/Core.php
):
<?php namespace Framework; use Symfony\Component\HttpFoundation\Request; use Symfony\Component\HttpFoundation\Response; use Symfony\Component\HttpKernel\HttpKernelInterface; class Core implements HttpKernelInterface { public function handle(Request $request, $type = HttpKernelInterface::MASTER_REQUEST, $catch = true) { switch ($request->getPathInfo()) { case '/': return new Response('Home'); case '/about': return new Response('About'); default: return new Response('Not Found!', Response::HTTP_NOT_FOUND); } } }
Update index.php
:
require 'lib/Framework/Core.php'; $request = Request::createFromGlobals(); $app = new Framework\Core(); $response = $app->handle($request); $response->send();
Improved Routing (Routing Component):
The Core
class is enhanced with a routing system using the Routing component:
<?php // ... (previous code) ... use Symfony\Component\Routing\Matcher\UrlMatcher; use Symfony\Component\Routing\RequestContext; use Symfony\Component\Routing\RouteCollection; use Symfony\Component\Routing\Route; use Symfony\Component\Routing\Exception\ResourceNotFoundException; class Core implements HttpKernelInterface { protected $routes; public function __construct() { $this->routes = new RouteCollection(); } public function handle(Request $request) { $context = new RequestContext(); $context->fromRequest($request); $matcher = new UrlMatcher($this->routes, $context); try { $attributes = $matcher->match($request->getPathInfo()); $controller = $attributes['controller']; unset($attributes['controller']); return call_user_func_array($controller, $attributes); } catch (ResourceNotFoundException $e) { return new Response('Not Found!', Response::HTTP_NOT_FOUND); } } public function map($path, $controller) { $this->routes->add($path, new Route($path, ['controller' => $controller])); } }
Routes are now defined in index.php
:
$app->map('/', function() { return new Response('Home'); }); $app->map('/about', function() { return new Response('About'); }); // ...
EventDispatcher:
The EventDispatcher component adds event handling capabilities. Add an on
method and a fire
method to the Core
class and a RequestEvent
class. (Implementation details omitted for brevity, but similar to the example in the original input). Listeners can be added using $app->on('request', ...);
.
This framework provides a foundation for building more complex applications using Symfony's power and flexibility. Remember to consult the official Symfony documentation for more advanced features and component details.
The above is the detailed content of Build your own PHP Framework with Symfony Components. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
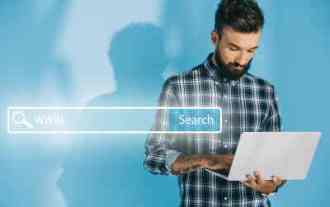
11 Best PHP URL Shortener Scripts (Free and Premium)
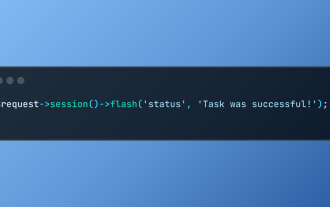
Working with Flash Session Data in Laravel
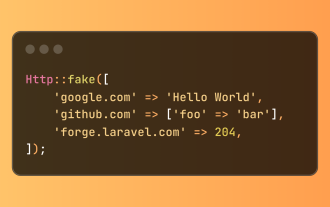
Simplified HTTP Response Mocking in Laravel Tests
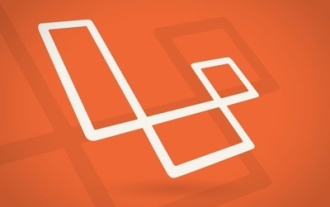
Build a React App With a Laravel Back End: Part 2, React
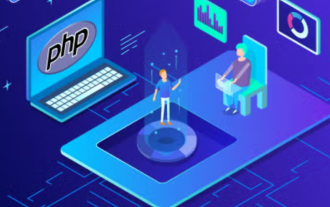
cURL in PHP: How to Use the PHP cURL Extension in REST APIs
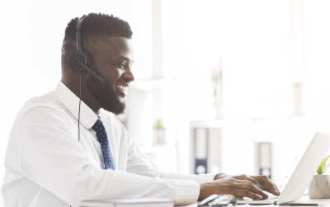
12 Best PHP Chat Scripts on CodeCanyon
