Watch: Adding a Lap Logger to a React Stopwatch
This tutorial extends our React stopwatch to include lap timing functionality – a crucial feature for any serious timekeeping application. We'll leverage our knowledge of React state management, arrays, and conditional rendering to achieve this. This is part 3 of the Building a Stopwatch in React series.
Frequently Asked Questions (FAQs) about Adding Lap Logging to a React Stopwatch
How to Add Lap Logging to a React Stopwatch?
Adding lap logging requires a function triggered by a "Lap" button. This function captures the stopwatch's current time and adds it to an array. The useState
hook manages this array.
const [laps, setLaps] = useState([]); const handleLap = () => { setLaps([...laps, currentTime]); };
Displaying Lap Times
Display lap times by mapping over the laps
array, rendering a component for each lap:
{laps.map((lap, index) => ( <p key={index}>Lap {index + 1}: {lap}</p> ))}
Formatting Lap Times
Improve readability by converting milliseconds to minutes, seconds, and milliseconds using a helper function:
const formatTime = (time) => { const minutes = Math.floor(time / 60000); const seconds = Math.floor((time - minutes * 60000) / 1000); const milliseconds = time - minutes * 60000 - seconds * 1000; return `${minutes}:${seconds}:${milliseconds}`; };
Resetting Lap Times
Resetting lap times involves clearing the laps
array:
const handleReset = () => { setLaps([]); // ...reset stopwatch... };
Ensuring Accurate Lap Times
Accuracy is paramount. The lap logging function should be synchronous to avoid delays in capturing the current time.
Adding Pause Functionality
Introduce a state variable to track whether the stopwatch is running:
const [isRunning, setIsRunning] = useState(false); // ...in the time increment function... if (isRunning) { // ...increment time... }
Resuming from Pause
Preserve the current time when pausing; only reset it during a full reset.
Implementing Lap Functionality (already covered above)
Persisting Lap Times Across Refreshes
Use localStorage
to store lap times, ensuring persistence even after page refreshes:
const handleLap = () => { const newLaps = [...laps, currentTime]; setLaps(newLaps); localStorage.setItem('laps', JSON.stringify(newLaps)); };
Responsive Design
Use CSS media queries to adjust the layout and sizing for different viewport sizes:
@media (max-width: 600px) { .stopwatch { font-size: 20px; } .lap-logger { font-size: 16px; } }
The above is the detailed content of Watch: Adding a Lap Logger to a React Stopwatch. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
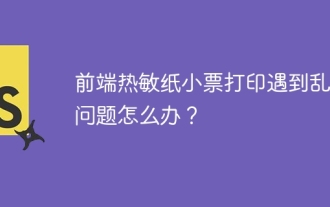
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
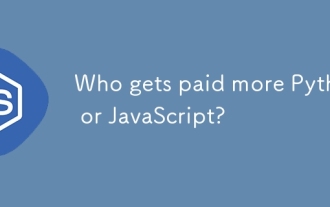
There is no absolute salary for Python and JavaScript developers, depending on skills and industry needs. 1. Python may be paid more in data science and machine learning. 2. JavaScript has great demand in front-end and full-stack development, and its salary is also considerable. 3. Influencing factors include experience, geographical location, company size and specific skills.
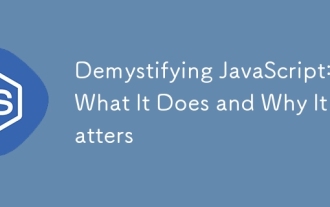
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
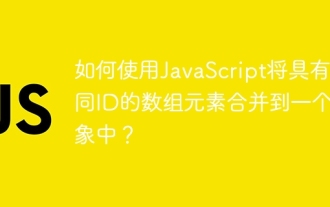
How to merge array elements with the same ID into one object in JavaScript? When processing data, we often encounter the need to have the same ID...
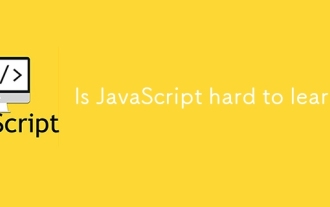
Learning JavaScript is not difficult, but it is challenging. 1) Understand basic concepts such as variables, data types, functions, etc. 2) Master asynchronous programming and implement it through event loops. 3) Use DOM operations and Promise to handle asynchronous requests. 4) Avoid common mistakes and use debugging techniques. 5) Optimize performance and follow best practices.
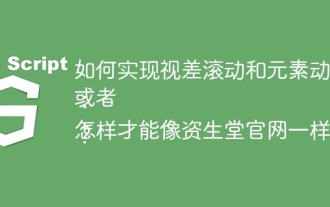
Discussion on the realization of parallax scrolling and element animation effects in this article will explore how to achieve similar to Shiseido official website (https://www.shiseido.co.jp/sb/wonderland/)...
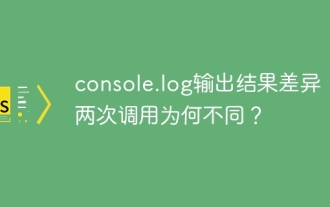
In-depth discussion of the root causes of the difference in console.log output. This article will analyze the differences in the output results of console.log function in a piece of code and explain the reasons behind it. �...
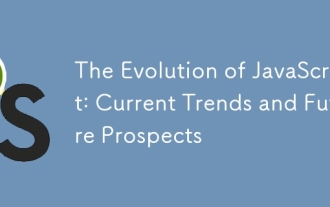
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
