API Building and Testing Made Easier with Postman
Postman: A powerful tool to simplify API development and testing
Key points:
- Postman is a powerful tool that simplifies API development and testing processes, provides functions such as saving past API calls and grouping related API calls, which is convenient and fast.
- APIs (Application Programming Interface) are usually created using the REST (Denotative State Transfer) framework, which sets up guidelines for web-based APIs. The four most common operations performed through the API are viewing, creating, editing, and deleting objects.
- API testing is a crucial part of the development process to ensure functionality, exception handling and security. This can be done by using the command line of the cURL library, but tools like Postman can make this process easier.
- Postman can be used to send requests to APIs such as Facebook Graph API, allowing developers to view posts, like posts, create posts, and delete posts. This tool can be a valuable asset for developers using APIs.
Thanks to Jeff Smith for his help, he was enthusiastic about participating in the peer review of this article.
Popular articles on SitePoint website today:
- What is HTTP/2?
- Use these three CSS methods to tame uncontrollable style sheets
- Your regular WordPress maintenance list
- Shared server hosting: Pros and Cons
- Why does every website require HTTPS
API (Application Programming Interface) is the medium for interaction between applications. APIs that use HTTP or HTTPS are called Web APIs.
If you look around the internet, you will find that a large number of services use APIs. Any service with social login uses the API; mobile applications for e-commerce websites use the API; and even ads you see on the internet use the API!
By creating APIs for your services, you can enable third-party developers to create applications based on your services. For example, social news site Frrole uses the Twitter API to generate contextual insights by analyzing a large number of tweets.
Assuming you have evaluated the pros and cons of creating an API, let's briefly discuss the process of creating and testing an API.
Create API
Although there are many ways to create APIs, the Web API is created using the REST (Denotative State Transfer) framework. The REST framework specifies a set of guidelines that must be followed when creating APIs. Due to the numerous APIs created every day, it becomes the standard for web-based APIs.
The four most common operations performed through the API are viewing, creating, editing, and deleting objects. The REST framework maps four HTTP verbs to these operations: GET, POST, PUT, and DELETE. Many verbs are added to this list, such as PURGE and PATCH, but in this article we will only discuss four basic verbs. An article about pragmatic RESTful API best practices written by Enchant.com founder Vinay Sahni may be useful for first-time developers.
Many frameworks today provide wrappers for the basic HTTP layer, making your development work easier. You just need to call the required command or function and focus on the function. Popular examples include Slim and Toro, two PHP-based miniature frameworks that can help you create REST APIs quickly.
Test API through CLI
The main purpose of creating APIs is to enable other applications (probably your own or developed by third parties) to use these services. Therefore, at every stage of the API development process, a crucial step is to test the API's functionality, exception handling, and security.
Using an API involves making a request to a desired resource (usually a URL) using one of the verbs (or methods). Depending on the requirements of the API you are using, you may need to add a header. One way to request such resources is through the command line.
In this article, we will focus on four parts of API calls—URL, HTTP verb, header, and parameters. We will use the cURL library to send requests to API resources through the CLI. cURL is a command line tool that helps transfer data using URL syntax - supports FTP, FTPS, HTTP, HTTPS.
Let's look at the following command:
<code>curl -i -X POST -H "Content-Type:application/json" http://www.my-api-example.com:port/ -d '{"Name":"Something"}'</code>
-i command stands for include, which tells the command that the header exists in the request. -X option follows the HTTP verb or method immediately. -H specifies the custom header added to the request. Finally, the -d option specifies the custom form data to be passed with the request.
The result of theAPI call is an HTTP response, usually encoded in JSON format. The response is provided with an HTTP response code that provides information about the request status (eg, 200 means OK, 404 means the resource does not exist, 500 means the server error, and 403 means the resource is prohibited). For example, the following response can be sent as a result of a previous request, as well as a 200 status code:
<code>{"message":"success","id":"4"}</code>
Testing such responses within the command line also poses a challenge, especially if the response has a large number of options.
This detailed guide from Codingpedia lists the CLI option list when testing the API.
Simplify testing with Postman
Postman is a powerful API development kit that makes the API development process fast and easy. It can be used as a Chrome extension and native applications for Mac, Windows and Linux. More than a million developers have tried it. To install Chrome extensions, you need to first install Chrome and then go to the project page of the Chrome Web Store.
Let's first understand how to simulate our previous CLI requests through Postman. The following image shows creating an API call in Postman with all four parts discussed above:
In addition to previewing (if the response is HTML), the responses you receive through the request can also be viewed in original or beautiful form. The following image shows different ways to view responses in Postman:
Postman automatically saves API calls you made in the past, which can save time when testing the API. Additionally, they can be grouped into related API calls for your convenience. Here is an example of API call history through Postman:
Using Facebook Graph API via Postman
A good way to demonstrate how Postman works is through the Facebook Graph API. In this post, we will focus on posts on the user’s timeline, how to view the details of posts, and how to create and delete posts.
Access tokens are crucial when using the Graph API. A token is associated with many permissions. For example, you can create a post using a token only if publish_actions is one of the selected fields when generating a token. This additional level of security can help you grant specific actions to a given application when logging in via Facebook.
View posts
To send a request to the Graph API, you need to generate an access token. After generating the token, you should send a GET request to the following URL and use access_token as a parameter:
<code>curl -i -X POST -H "Content-Type:application/json" http://www.my-api-example.com:port/ -d '{"Name":"Something"}'</code>
The following image shows the basic Graph API call with your details:
You can check the list of posts on your timeline by following the command:
<code>{"message":"success","id":"4"}</code>
Here is how the details of the post list on your timeline may appear:
To view the details of a single post, use the following resources:
<code>GET /me/</code>
The details of a single post are as follows:
Like
To like, just send a POST request to the following URL (please note that likes require publish_actions):
<code>GET /me/feed/</code>
Send a like request through Postman as follows:
Similarly, you can delete the like by sending a DELETE request to the same URL:
<code>GET /post-id/</code>
Create a post
To create a post, you need to send some parameters in addition to the POST request. You also need publish_actions to do this.
You can add the following options to your POST request:
- message: Message associated with the post
- link: Link to external resources
- place: The location associated with the post (similar to "check-in" a location)
- tags: any friend or page
- privacy: The audience for posts to be visible
- object_attachment: Any existing Facebook post
The documentation explains how all these functions are used.
You can create posts on your own timeline or on the timeline of users, pages, events or groups based on the URL you choose to send the request:
<code>curl -i -X POST -H "Content-Type:application/json" http://www.my-api-example.com:port/ -d '{"Name":"Something"}'</code>
Let's try to post to our own timeline. If the request succeeds, we will get the ID of the created post. Here is an example of creating a new post using the Graph API:
To edit a post, you need to send a POST request (not PUT) to the following URL and use the same parameters as you would when you create a post:
<code>{"message":"success","id":"4"}</code>
Delete posts
Just like deleting a like, deleting a post requires you to send a DELETE request to the post URL:
<code>GET /me/</code>
Note: We have seen in this article that Postman has many useful features. After upgrading the free version ($9.99 per license), there are more features – like running API collections on multiple data values.
Conclusion
Creating an API is a crucial task, involving many important steps. Postman makes the thorough testing process easier. Currently, Postman seems to solve the problem of the average developer well. However, how it evolves with the paradigm shifts commonly found in the web industry remains to be seen.
Are we missing the important features of Postman? Are you using another client to test the API? Please let us know in the comments below.
Frequently Asked Questions about Building and Testing APIs with Postman
What is Postman and how does it help build and test APIs?
Postman is a popular tool for developers to build and test APIs. It provides a user-friendly interface that allows easy sending HTTP requests and viewing responses. Postman supports various types of HTTP requests, such as GET, POST, DELETE, PUT, etc. It also allows you to save requests and organize them into a collection for easy access and sharing. Postman's built-in test features allow you to write tests for your API directly in the tool itself, making it a comprehensive solution for API development.
Is Postman free to use?
Postman offers free and paid versions. The free version, called Postman Basic, offers a powerful set of features that are enough to meet the needs of individual developers or small teams. Postman offers a paid plan for large teams or businesses that require advanced features and collaboration.
How is Postman Pro different from the basic version?
Postman Pro is a paid version that offers additional features not found in the basic version. These include team collaboration, API monitoring, advanced API documentation, and more. It is designed for professional developers and teams who need to manage complex API development projects.
How to buy Postman?
You can buy Postman from their official website. They offer different pricing plans based on your team size and specific needs. You can choose a plan that suits your needs and follow the prompts to complete the purchase.
What is the role of Postman in software development?
In software development, Postman plays a crucial role in API development and testing. It allows developers to build, test and document APIs on a single platform, thus simplifying the development process. It also supports collaboration, making it easier for teams to work on API projects together.
Can I use Postman for automated testing?
Yes, Postman supports automated testing. You can write tests for your API in Postman and run them automatically using Postman's Collection Runner or Newman (Postman's command line tool). This makes it easier to integrate API testing into your Continuous Integration/Continuous Delivery (CI/CD) pipeline.
How to organize my API requests in Postman?
Postman allows you to organize API requests into collections. A collection is a set of related requests that can be saved together. This makes it easier to manage and share your requests. You can also add folders to the collection for further organization.
Does Postman support different types of authentication?
Yes, Postman supports various authentication types, including Basic Auth, Bearer Token, OAuth 1.0, OAuth 2.0, and more. This makes it a versatile tool for testing APIs with different authentication requirements.
Can I share my Postman collection with others?
Yes, Postman allows you to share your collection with others. This is especially useful for teamwork. If you are using a paid version, you can share the collection by exporting it as a JSON file or sharing directly from Postman.
How to learn to use Postman effectively?
There are many resources to learn Postman. The official Postman website provides comprehensive documentation and tutorials. There are also many online courses, blogs and forums where you can learn the experiences of other Postman users.
The above is the detailed content of API Building and Testing Made Easier with Postman. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










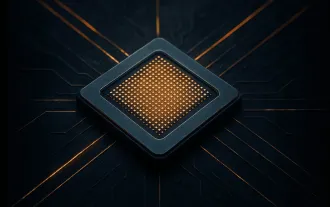
This pilot program, a collaboration between the CNCF (Cloud Native Computing Foundation), Ampere Computing, Equinix Metal, and Actuated, streamlines arm64 CI/CD for CNCF GitHub projects. The initiative addresses security concerns and performance lim
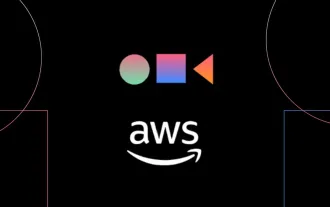
This tutorial guides you through building a serverless image processing pipeline using AWS services. We'll create a Next.js frontend deployed on an ECS Fargate cluster, interacting with an API Gateway, Lambda functions, S3 buckets, and DynamoDB. Th
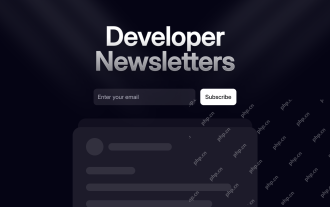
Stay informed about the latest tech trends with these top developer newsletters! This curated list offers something for everyone, from AI enthusiasts to seasoned backend and frontend developers. Choose your favorites and save time searching for rel
